What is a vector class?
The vector class is available in Java and C++ programming languages. It is a class implementing a dynamic array. A dynamic array is an array whose size automatically increases when an element is added to it. The vector class stores elements in a contiguous memory block and assigns the memory to the elements whenever required during execution.
Syntax of vector class
The syntax of vector in C++ language is as follows:
vector <object_type> vector_var_name;
Here,
vector is the keyword used to declare a vector class.
object_type is the data type of the element.
vector_var_name is the name of the vector variable.
To use a vector class in C++, it is mandatory to include the header file: #include<vector> at the beginning of the code.
Example of vector class
Consider the following C++ code:
#include<iostream>
#include<vector>
using namespace std;
int main()
{
vector<string> vec;
vec.push_back("Hello ");
for(vector<string>::iterator it=vec.begin(); it!=vec.end(); ++it)
cout<<*it;
return 0;
}
Output:
Hello
Explanation: The above code begins with the declaration of the header files, followed by the main function. Within main(), the vector vec with string datatype is created. The push_back() function is used to insert elements into the vector. Here, the string Hello is inserted in the vector. The for loop is then used to print the vector elements.
Initialization of vectors in C++
In C++, vectors can be initialized in four ways:
- By pushing values one-by-one
- By using an overloaded constructor
- By using arrays
- By using another initialized vector
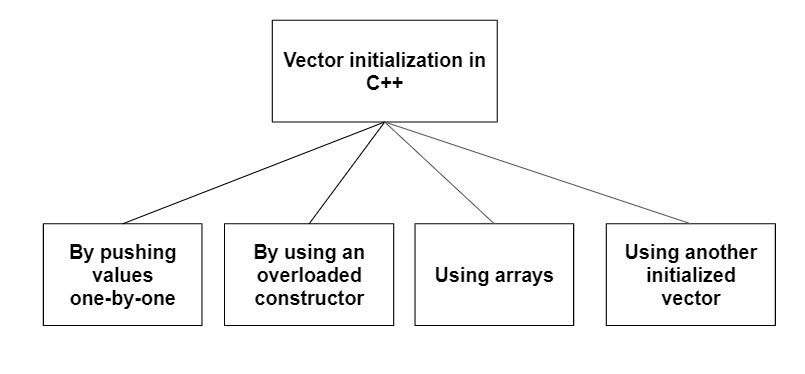
By pushing values one-by-one
The elements in a vector can be inserted (pushed) in a vector class one by one using the push_back() method. The algorithm is as follows:
Start
Declare v of vector type
Invoke push_back() method to insert elements to vector v
Print “Vector elements:”
for (int a:v)
Print all elements of variable a
End
Syntax: vector_name.push_back(element_value);
Example: v1.push_back(a);
By using an overloaded constructor
This approach is used when a vector contains multiple elements with the same value. The algorithm for this approach is as follows:
Start
Initialize a variable (say a)
Create a vector (say vec1) with size ’a’
Initialize vector vec1
Initialize vec2 by vec1
Print the vector elements
End
Syntax: vector<object_type> vector_name(number_of_repetitions, element_value);
Example: int a= 5;
vector<int> v1(a, 8);
By using arrays
In this approach, an array containing the elements to be stored in the vector is passed as a parameter to the vector class. The algorithm for this approach is as mentioned below:
Start
Create a vector (say vec)
Initialize the vector
Print the elements
End
Syntax: vector<object_type> vector_name{value1,value2,....,valuen};
Example: vector<int> vec{2,6,1,4,9};
By using another initialized vector
This approach creates a new vector from an existing vector having the same values. It passes begin() and end() iterators of the initialized vector. The algorithm for this approach is given below:
Start
Create a vector vec1
Initialize the vector vec1 using an array.
Initialize vector vec2 by vec1
Print the elements
End
Syntax: vector<object_type> vector_name1{value1,value2,....,valuen};
vector<object_type> vector_name2(vector_name1.begin(), vector_name1.end())
Example: vector<int> vec1{2,6,1,4,9};
vector<int> vec2(vec1.begin(), vec1.end());
Vector functions in C++
Some functions used in the vector class in C++ include:
Function name | Description | Example |
begin() | This function refers to the first element in the vector. | v1.begin() |
end() | This function refers to the last element in the vector. | v1.end() |
rbegin() | This function refers to the last element in the vector. | v1.rbegin() |
rend() | This function refers to the element preceding the first element in the vector. | v1.rend() |
cbegin() | This function returns a constant iterator referring to the first element in the vector. | v1.cbegin() |
cend() | This function returns a constant iterator referring to the element that follows the last element in the vector. | v1.cend() |
crbegin() | This function returns a constant iterator referring to the last element in the vector. | v1.crbegin() |
crend() | This function returns a constant iterator referring to the element preceding the first element in the vector. | v1.crend() |
size() | This function returns the number of elements present in the vector. | v1.size() |
max_size() | This function indicates the maximum number of elements that can be stored in the vector. | v1.max_size() |
capacity() | This function indicates the storage space allocated to the vector currently. | v1.capacity() |
resize(n) | This function resizes the container to store n elements. | v1.resize(4) |
empty() | This function indicates whether the container is empty or not. | v1.empty() |
reference_operator[g] | This function returns a reference to the g element in a vector. | v1[2] |
at(g) | This function returns a reference to the element at position g in a vector. | v1.at(4) |
front() | This function returns a reference to the first element of the vector. | v1.front() |
back() | This function returns a reference to the last element in the vector. | v1.back() |
data() | This function points to the memory array used to store the vector elements. | v1.data() |
assign() | This function allocates a new value to the vector elements by replacing the older ones. | v1.assign(7, 11) |
push_back() | This function pushes elements to the vector from the back. | v1.push_back(20) |
pop_back() | This function removes elements to the vector from the back. | v1.pop_back(20) |
insert() | This function inserts elements at the specified location. | v1.insert(v1.begin(), 3) |
erase() | This function removes elements at the specified location or range. | v1.erase(4) |
swap() | This function swaps the contents of one vector with another vector having the same data type. | v1.swap(v2) |
clear() | This function deletes all the vector elements. | v1.clear() |
emplace() | This function increases the vector by adding new elements before the specified position. | v1.emplace(v1.begin(), 7) |
emplace_back() | This function adds new elements at the end of the vector. | v1.emplace_back(5) |
Advantages of using vectors
The benefits of using vectors in a program are:
- Vectors are dynamic. (Their size can be increased easily.)
- Inserting and deleting elements in vectors is easy.
- Multiple elements can be stored in vectors.
- Copying elements from one vector to another is easy.
Context and Applications
The topic operations of vector class is a significant concept in programming languages. Students studying the courses listed below study this concept.
- Bachelor of Technology in Computer Science Engineering
- Master of Technology in Computer Science Engineering
- Bachelor of Science in Information Technology
- Master of Science in Information Technology
Practice Problems
Q1. What do vectors implement?
- Dynamic arrays
- Vector object cout
- Big-Ocout
- Current capacity
Answer: Option a
Explanation: Vectors are containers that implement dynamic arrays.
Q2. Which header file should be included in the program to use vectors?
- <Non-member int index cout allocator>
- <Allocator cout>
- <random-access iterator cout allocator>
- <vector>
Answer: Option d
Explanation: The header file <vector> includes the implementation of all vector methods, so it is necessary to add this file in the program while using vectors.
Q3. Which of these is used to access the first element of a vector vec?
- cout.allocator()
- vec.begin()
- v1.push _ back.int index cout()
- random-access cout allocator()
Answer: Option b
Explanation: The begin() method is used to access the first element of a vector. So, for vector vec, the method vec.begin() will be used to access the first element of the vector.
Q4. Which function returns the number of elements in the vector?
- Linear Algorithms operation cout()
- size()
- Coutdestructor return 0()
- scalar vector object()
Answer: Option b
Explanation: The size function returns the number of elements present in the vector.
Q5. Which function removes all vector elements?
- cout v1.push _ back()
- dynamically random-access iteratorcout()
- clear()
- v1.push _ backcout()
Answer: Option c
Explanation: The clear() function deletes all the elements in the vector.
Common Mistakes
Students should follow the correct syntax of vectors and their functions. If students make mistakes in writing the syntax, it will generate a compilation error, and the program will fail to execute.
Related Concepts
- Deque
- Arrays
- Templates
- Functions
- Iterators
- C++ algorithms
Want more help with your computer science homework?
*Response times may vary by subject and question complexity. Median response time is 34 minutes for paid subscribers and may be longer for promotional offers.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.
Operations of vector class Homework Questions from Fellow Students
Browse our recently answered Operations of vector class homework questions.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.