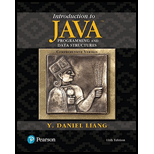
(Math: approximate the square root) There are several techniques for implementing the sqrt method in the Math class. One such technique is known as the Babylonian method. It approximates the square root of a number, n, by repeatedly performing the calculation using the following formula:
nextGuess = (lastGuess + n / lastGuess) / 2
When nextGuess and 1astGuess are almost identical, nextGuess is the approximated square root. The initial guess can be any positive value (e.g., 1). This value will be the starting value for 1astGuess. If the difference between nextGuess and 1astGuess is less than a very small number, such as 0. 0001 , you can claim that next Guess is the approximated square root of n. If not, nextGuess becomes 1astGuess and the approximation process continues. Implement the following method that returns the square root of n:
public static double sqrt(long n)

Want to see the full answer?
Check out a sample textbook solution
Chapter 6 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Additional Engineering Textbook Solutions
Web Development and Design Foundations with HTML5 (8th Edition)
Java: An Introduction to Problem Solving and Programming (8th Edition)
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Starting Out With Visual Basic (8th Edition)
INTERNATIONAL EDITION---Engineering Mechanics: Statics, 14th edition (SI unit)
Elementary Surveying: An Introduction To Geomatics (15th Edition)
- 4) Roll the dice 2 Make a program that tests the probability of a certain score when rolling x dice. The user should be able to choose to roll eg 4 dice and test the probability of a selected score eg 11. The program should then do a number of simulations and answer how big the probability is for the selected score with as many dice selected. There must be error checks so that you cannot enter incorrect sums, for example, it is not possible to get the sum 3 if you roll 4 dice. TarningsKast2 How many dices do you want to throw? 11 Which number do you want the probability for? The probability the get the number 11 with 4 dices is 7.19% Calculatearrow_forward1) Simple Calculator: In Python, implement a simple calculator that does the following operations: summation, subtraction, multiplication, division, sqrt, power, natural log and abs. a) Follow the instructions below: To work with the calculator, the user is asked to enter the first number, then the operation, and finally, a second number if required. Your code has to recognize the need for the second number and ask for it if required. After performing one operation, the calculator prints the output of the operation. After performing one operation, the calculator must not exit. It has to start again for the next operation. The calculator will be closed if the user writes 'e' as any input. Use functions to perform the operations and the appropriate conditions to prevent common errors such as entering characters as one of the numbers etc. b) Run your code and provide the results for at least one example per operation. - -arrow_forwardBisection method only octave/math labarrow_forward
- Python Programming ONLY PLEASE NO JAVA Use recursion to determine the number of digits in an integer n. Hint: If n is < 10, it has one digit. Otherwise, it has one more digit than n / 10. Write the method and then write a test program to test the method Your test program must: Ask the user for a number Print the number of digits in that number. (describe the numbers printed to the console) Use program headers and method header comments in your code.arrow_forwardFinancial Application:• Write a program that computes future investment value at a given interest rate for aspecified number of months and prints the report shown in the sample output. • Given the annual interest rate, the interest amount earned each month is computedusing the formula: Interest earned = investment amount * annual interest rate /1200 (=months * 100) • Write a method, computeFutureValue, which receives the investment amount, annualinterest rate and number of months as parameters and does the following: o Prints the interest amount earned each month and the new value of theinvestment (hint: use a loop). o Returns the total interest amount earned after the number of months specified bythe user. The main method will:o Ask the user for all input needed to call the computeFutureValue method.o Call computeFutureValueo Print the total interest amount earned by the investment at the end of thenumber of months entered by the user. Sample Program runningEnter the investment…arrow_forwardFinancial Application:• Write a program that computes future investment value at a given interest rate for aspecified number of months and prints the report shown in the sample output. • Given the annual interest rate, the interest amount earned each month is computedusing the formula: Interest earned = investment amount * annual interest rate /1200 (=months * 100) • Write a method, computeFutureValue, which receives the investment amount, annualinterest rate and number of months as parameters and does the following: o Prints the interest amount earned each month and the new value of theinvestment (hint: use a loop). o Returns the total interest amount earned after the number of months specified bythe user. The main method will:o Ask the user for all input needed to call the computeFutureValue method.o Call computeFutureValueo Print the total interest amount earned by the investment at the end of thenumber of months entered by the user. Sample Program runningEnter the investment…arrow_forward
- Area and Perimeter: Write a program that prints the area and perimeter of a circle with a radius of 5.5 using the formula:• Perimeter = 2 * radius * PI• Area = radius * radius * PI• Use Math.PI for PI in your programarrow_forwardOnly Java programmingarrow_forwardParallel Lines python 3 Write a program to draw a set of linear lines with equation y = mx + b where m is the slope of the line and b is the y-intercept. Prompt user to enter the slope and y-intercept. Draw the line from x=-10 to x=10. Use numpy arange method to generate x values from -10 to 10 with step size of 0.1. For example., x = np.arange(-10, 10, 0.1) Use the plt.axis() function to set the range of x and y values from -10 to 10. Add xlabel with x-axis, ylabel with y-axis, and title Parallel Lines. After you have drawn the line, draw two lines that are parallel to the first line. Hint: Two lines are parallel if they have the same slope but different y-intercept. Draw two lines where the y-intercept value is one unit below the first line, and one unit above the first line. or example, if the first line y-intercept is 4, then draw lines with y-intercept of 3 and y-intercept of 5.arrow_forward
- C# Part 1: In your program, write a method that accepts a positive integer and returns true if the number is a prime number and false otherwise. Note that 2 is the only even prime number. An odd integer is prime if it not divisible by any odd integer less than or equal to the square root of the number. Part2: A prime number whose reversal is also a prime number is called emirp. For example, 11, 13, 79 and 359 are emirps. Write a program that outputs to a ListBox the first 100 emirps. Your program must contain the method from Part 1 that returns true if a number is prime; false otherwise and another method that returns the reversal of a positive number. You can reverse the integer in the following way: • Convert the integer into a string value = num.ToString(); • Use the following code to reverse the integer string newNum = “”; for (int l = value.Length; l > 0; l--) newNum = newNum + value[l-1]; Also, your program should have a TextBox that will accept a positive integer from the…arrow_forwardpython-Exercise 1 Write a python program to display the greater number between 2 numbers. The numbers are given by user. Input : 17 -6 Output: The greatest number is 17 2.Use a class and add a method called greatestNumberarrow_forward*Using Python The scientist has 500 cages in which to hold her rabbits. Each cage holds one pair of rabbits. Assuming that no rabbits ever die, when will she run out of cages? Your program must do the following: Print a table that contains the following information for each month. The number of months that have passed. The number adult rabbit pairs (those over 1 month old). The number of baby rabbits pairs produced this month. The total number of rabbit pairs in the lab. Calculate how many months it will take until the number of rabbits exceeds the number of available cages. Stop printing when you run out of cages. Print a message giving how many months it will take to run out of cages Output file should look like the following. Comments in the file begin with '#', and must appear as shown too: Code must contain def main(): #main function need in all programs for automated testing """ Program starts here """ #end of main program if __name__ == '__main__': main() #excucte main…arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
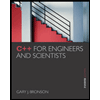
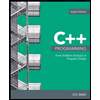