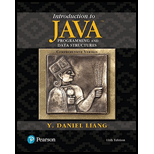
Concept explainers
(Math: pentagonal numbers) A pentagonal number is defined as n(3n − 1)/2 for n = 1, 2, ... , and so on. Therefore, the first few numbers are 1, 5, 12, 22, .... Write a method with the following header that returns a pentagonal number:
public static int getPentagonalNumber(int n)
For example, getPentagonal Number (1) returns 1 and getPentagonalNumber(2) returns 5. Write a test

Program Plan:
- Declare the class “Penta”
- Definition for main class
- Use for loop to iterate the variable “j” equals to “100”.
- Check whether “j” mod “10” equals to 0.
- Call the method “getPentagonalNumber()”.
- Otherwise, call the method “getPentagonalNumber()” concatenate with space.
- Method definition for “getPentagonalNumber()”
- Return “n * (3 * n - 1) / 2”.
Program to display first 100 pentagonal numbers with 10 numbers on each line using the method “getPentagonalNumber ()”
Explanation of Solution
Program:
//Definition for class "Penta"
public class Penta
{
//Definition for main class
public static void main(String[] args)
{
//For loop to iterate the variable
for (int j = 1; j <= 100; j++)
//Check whether the variable "j" mod 10 equals to "0"
if (j % 10 == 0)
//Call the method "getPentagonalNumber"
System.out.println(getPentagonalNumber(j));
else
//Call the method "getPentagonalNumber"
System.out.print(getPentagonalNumber(j) + " ");
}
//Method definition for "getPentagonalNumber"
public static int getPentagonalNumber(int n)
{
//Return the result
return n * (3 * n - 1) / 2;
}
}
1 5 12 22 35 51 70 92 117 145
176 210 247 287 330 376 425 477 532 590
651 715 782 852 925 1001 1080 1162 1247 1335
1426 1520 1617 1717 1820 1926 2035 2147 2262 2380
2501 2625 2752 2882 3015 3151 3290 3432 3577 3725
3876 4030 4187 4347 4510 4676 4845 5017 5192 5370
5551 5735 5922 6112 6305 6501 6700 6902 7107 7315
7526 7740 7957 8177 8400 8626 8855 9087 9322 9560
9801 10045 10292 10542 10795 11051 11310 11572 11837 12105
12376 12650 12927 13207 13490 13776 14065 14357 14652 14950
Want to see more full solutions like this?
Chapter 6 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Additional Engineering Textbook Solutions
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
C Programming Language
Absolute Java (6th Edition)
Artificial Intelligence: A Modern Approach
Web Development and Design Foundations with HTML5 (9th Edition) (What's New in Computer Science)
Problem Solving with C++ (9th Edition)
- 1)Write a method that takes an array of ints and returns the sum of all numbers that are even. For example, given an array {3, 4, 6}, you would return 10. 2)For this exercise, you are given an array of integers. Return true if every element in the array is an odd number, otherwise return false. 3) Given an array of strings, return the array in reverse order. For example, given: reverse({"one", "two", "three"}); You would return: {"three", "two", "one"} 4) For this exercise, you need to shift all the elements in the array one space to the right (increase the index of the element by 1). The last element will wrap around and become the new first element. For example, given: moveUp({"first", "second", "third", "fourth"}); You would return: {"fourth", "first", "second", "third"}arrow_forwardtask4-Change Summation Integers problem to Finding the minimumproblem. Make sure you properly wrote/updated all text messages, method names,and math calculations.Hint: You can use java.lang.Math.min() method.Example: System.out.printf("The min of the integers %4d and %4d and %4dis %7d\n", a, b, c, MinTest(a, b, c));Submit the source code files (.java files) and the console output screenshotsarrow_forward2. A pentagonal number is defined as n(3n-1)/2 for n = 1, 2, ..., etc.. Therefore, the first few numbers are 1, 5, 12, 22, ... . Write a method with the following header that returns a pentagonal number: %3D public static int getPentagonalNumber(int n) For example, getPentagonalNumber(1) returns 1 and getPentagonalNumber(2) returns 5. Write a test program that uses this method to display the first 100 pentagonal numbers with 10 numbers on each line. Numbers are separated by exactly one space.arrow_forward
- What is the solution of this problem using java language?arrow_forward(Number of days in a year) Write a method that returns the number of days in a year using the following header: public static int numberOfDaysInAYear(int year)Write a test program that displays the number of days in year from 2000 to 2020.arrow_forwarda.Write the method that finds the first 4 numbers out of 100 randomly generated numbers. After the numbers are generated in main, they should be sent to the method given as an example below and the smallest four numbers should be found. Write the numbers found on the console screen in the main method.(will be coded in java) Method name: public static int[] smallestFindFirstFourth(int[] array) Note: Sorting algorithms or ready-made functions will never be used. b.In the attached city list.txt file, the names of the provinces in Turkey are given in a single line according to the format given in Figure 1. In the application you will write, write the city names read from this file into the license plate list.txt file in the format shown in Figure 2. In more descriptive terms, citylist.txt is a file given to you. license plate list.txt is the file you will get with your code. city list: Adana, Adıyaman, Afyon, Ağrı, Amasya, Ankara, Antalya, Artvin, Aydın, Balıkesir, Bilecik, Bingöl, Bitlis,…arrow_forward
- a.Write the method that finds the first 4 numbers out of 100 randomly generated numbers. After the numbers are generated in main, they should be sent to the method given as an example below and the smallest four numbers should be found. Write the numbers found on the console screen in the main method.(will be coded in java) Method name: public static int[] smallestFindFirstFourth(int[] array) Note: Sorting algorithms or ready-made functions will never be used. b.In the attached city list.txt file, the names of the provinces in Turkey are given in a single line according to the format given in Figure 1. In the application you will write, write the city names read from this file into the license plate list.txt file in the format shown in Figure 2. In more descriptive terms, citylist.txt is a file given to you. license plate list.txt is the file you will get with your code. city list: Adana, Adıyaman, Afyon, Ağrı, Amasya, Ankara, Antalya, Artvin, Aydın, Balıkesir, Bilecik, Bingöl, Bitlis,…arrow_forwardComplete the convert() method that casts the parameter from a double to an integer and returns the result.Note that the main() method prints out the returned value of the convert() method. Ex: If the double value is 19.9, then the output is: 19 Ex: If the double value is 3.1, then the output is: 3 code: public class LabProgram { public static int convert(double d){ /* Type your code here */ } public static void main(String[] args) { System.out.println(convert(19.9)); System.out.println(convert(3.1)); }}arrow_forwardWhat is the solution for this question ( using java language)arrow_forward
- Write down only the methods that are required; testers are not requiredarrow_forward(language: Intellij-Java) Part One: Create a method which takes two int parameters and swaps them. Print the values before and after swapping. (Example: ‘before swap: x = 5, y = 10; after swap: x=10, y=5). Part2: Write a method which gets a value from the user and convert that value from Celsius to Fahrenheit: - For Celsius to Fahrenheit conversion use: F = 9*C/5 + 32 - Print the result of the conversion Part3: Create a method which takes an integer array input from the user and reverses the elements in the array. Print the reversed array. - For example, the expected “reversed” result for an array [5,33,0,8,2] is [2,8,0,33,5] - Don't use any inbuilt/existing array reverse method. Try to write the reversing logicarrow_forwardPlease help me! I have a lot of mini problems. I'm not sure how to start After #6, I have other problems. Those are : 7.) Write a method that reads a one-line sentence as input and then displays the following response: If the sentence ends with a question mark (?) and the input contains an even number of characters, display the word Yes. If the sentence ends with a question mark and the input contains an odd number of characters, display the word No. If the sentence ends with an exclamation point (!), display the word Wow. In all other cases, display the words You always say followed by the input string enclosed in quotes. Your output should all be on one line. Be sure to note that in the last case, your output must include quotation marks around the echoed input string. In all other cases, there are no quotes in the output. Your program does not have to check the input to see that the user has entered a legitimate sentence. Notes: This code requires a three-way selection statement and…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
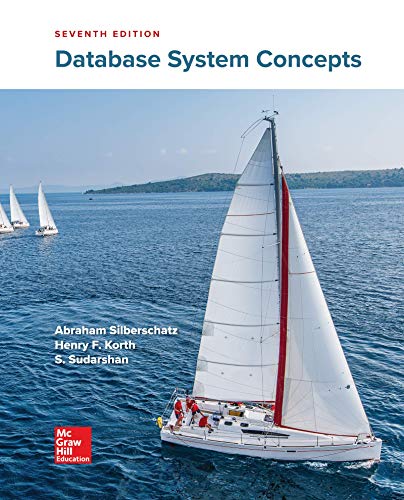
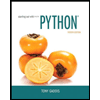
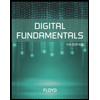
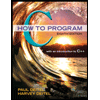
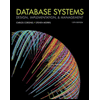
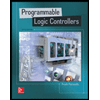