python 3 Write a program to draw a set of linear lines with equation y = mx + b where m is the slope of the line and b is the y-intercept. Prompt user to enter the slope and y-intercept. Draw the line from x=-10 to x=10. Use numpy arange method to generate x values from -10 to 10 with step size of 0.1. For example., x = np.arange(-10, 10, 0.1) Use the plt.axis() function to set the range of x and y values from -10 to 10. Add xlabel with x-axis, ylabel with y-axis, and title Parallel Lines. After you have drawn the line, draw two lines that are parallel to the first line. Hint: Two lines are parallel if they have the same slope but different y-intercept. Draw two lines where the y-intercept value is one unit below the first line, and one unit above the first line. or example, if the first line y-intercept is 4, then draw lines with y-intercept of 3 and y-intercept of 5.
Parallel Lines
Write a program to draw a set of linear lines with equation
y = mx + b
where m is the slope of the line and b is the y-intercept. Prompt user to enter the slope and y-intercept. Draw the line from x=-10 to x=10. Use numpy arange method to generate x values from -10 to 10 with step size of 0.1. For example.,
x = np.arange(-10, 10, 0.1)Use the plt.axis() function to set the range of x and y values from -10 to 10. Add xlabel with x-axis, ylabel with y-axis, and title Parallel Lines.
After you have drawn the line, draw two lines that are parallel to the first line. Hint: Two lines are parallel if they have the same slope but different y-intercept. Draw two lines where the y-intercept value is one unit below the first line, and one unit above the first line. or example, if the first line y-intercept is 4, then draw lines with y-intercept of 3 and y-intercept of 5.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

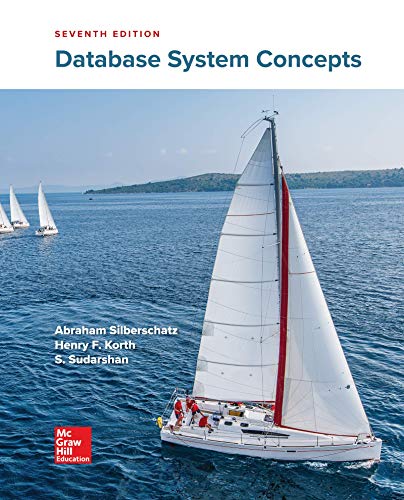
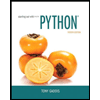
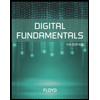
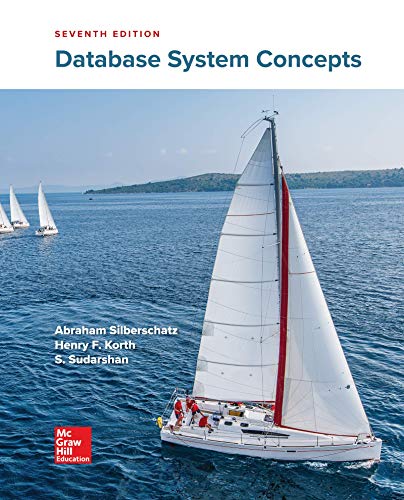
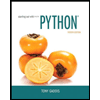
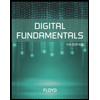
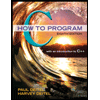
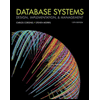
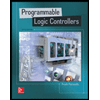