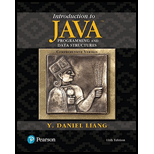
Concept explainers
(Financial application: compute the future investment value) Write a method that computes future investment value at a given interest rate for a specified number of years. The future investment is determined using the formula in
Use the following method header:
public static double futureinvestmentValue (
double investmentAmount, double monthlyinterestRate,int years)
For example, futureinvestmentValue(10000, 0.05/12 , 5) returns 12833 0 59.
Write a test program that prompts the user to enter the investment amount (e.g., 1,000) and the interest rate (e.g., 9%) and prints a table that displays future value for the years from 1 to 30, as shown below:

Want to see the full answer?
Check out a sample textbook solution
Chapter 6 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Additional Engineering Textbook Solutions
Starting Out with Python (3rd Edition)
Problem Solving with C++ (10th Edition)
Starting Out with C++ from Control Structures to Objects (8th Edition)
Starting Out With Visual Basic (7th Edition)
Web Development and Design Foundations with HTML5 (8th Edition)
- (Number of days in a year) Write a method that returns the number of days in a year using the following header: public static int numberOfDaysInAYear(int year)Write a test program that displays the number of days in year from 2000 to 2020.arrow_forwardCode in C# (OOP Concept) Do a program that does the following: Ask for a number from the user in the main method o Pass thenumber to a method call it "Multiplication”. In the Method Multiplication, multiply the number by a number enteredby the user (mention that the number should be positive). Send the result back to the main and print it from the mainModify the previous program if the user enters a negative second number in the method Multiplication, do not acceptthe number and ask the user to reenter it again. . Add another method call it also Multiplication Ask for a float numberfrom the main and send it to the new Multiplication. This method should also ask for another positive number fromthe user and multiply it by the float number. Design and implement a function called "multiply" in C++ that accepttwo numbers and returns either the arithmetic multiplication or repetition of a given string a specific number of timesdepending on the data types of the argument values. The…arrow_forward(language: Intellij-Java) Part One: Create a method which takes two int parameters and swaps them. Print the values before and after swapping. (Example: ‘before swap: x = 5, y = 10; after swap: x=10, y=5). Part2: Write a method which gets a value from the user and convert that value from Celsius to Fahrenheit: - For Celsius to Fahrenheit conversion use: F = 9*C/5 + 32 - Print the result of the conversion Part3: Create a method which takes an integer array input from the user and reverses the elements in the array. Print the reversed array. - For example, the expected “reversed” result for an array [5,33,0,8,2] is [2,8,0,33,5] - Don't use any inbuilt/existing array reverse method. Try to write the reversing logicarrow_forward
- Using METHOD SAMPLE Problem: Create a method that will compute the value of PI, if pi is 3.14. Given formula is : value = pi x square of radius Solution: float area(int radius) final float pi = 3.14f; float val; val = pi * (float) (radius * radius); return(val); Direction: Analyze the given problem and create a java method. Problem: Create a method that will compute the Mass (m). Acceleration(a) is 9.80. if Force (F) is equal to acceleration multiplied by mass.arrow_forwardtask4-Change Summation Integers problem to Finding the minimumproblem. Make sure you properly wrote/updated all text messages, method names,and math calculations.Hint: You can use java.lang.Math.min() method.Example: System.out.printf("The min of the integers %4d and %4d and %4dis %7d\n", a, b, c, MinTest(a, b, c));Submit the source code files (.java files) and the console output screenshotsarrow_forward42) Look at the following method: public static int test2 (int x, int y) if (x < y) { return -5; } else { return (test2 (x - y, y + 5) + 6) ; } } What is returned for test2 (10, 20)? A) -5 B) 1 C) 6 D) 10 43) This term is used for methods that directly call themselves. A) Simple recursion C) Absolute recursion B) Direct recursion D) Native recursion 44) If the base case in a recursive method is never reached: A) The result will always be off by one. C) The method will call itself indefinitely. B) The method will call itself only once. D) The method will never call itself. 45) A(n) is an object that is generated in memory as the result of an error or an unexpected event. A) exception C) default exception handler B) exception handler D) error message 46) All of the exceptions that you will handle are instances of classes that extend this class. A) Exception C) IOException B) RunTimeException D) Error 47) All exceptions are instances of dasses that extend this class. A) Throwable C)…arrow_forward
- Q1) Write a method that checks whether two words are anagrams. Two words are anagrams if they contain the same letters in any order. For example, "silent" and "listen" are anagrams. Write a test program that prompts the user to enter two strings and, if they are anagrams, displays "anagram", otherwise displays "not anagram". Note: The header of the method is as follows: public static boolean isAnagram(String s1, String s2) Sample Input #1: Enter two strings: Silent listen Sample Output #1: The string “Silent” and “listen” are anagrams. Sample Input #1: Enter two strings: teach peach Sample Output #1: The string “teach” and “peach” aren’t anagrams.arrow_forwardHere's the problem " (Math: approximate the square root) There are several techniques for implementingthe sqrt method in the Math class. One such technique is known as theBabylonian method. It approximates the square root of a number, n, by repeatedlyperforming a calculation using the following formula: nextGuess = (lastGuess + n / lastGuess) / 2 When nextGuess and lastGuess are almost identical, nextGuess is theapproximated square root. The initial guess can be any positive value (e.g., 1).This value will be the starting value for lastGuess. If the difference betweennextGuess and lastGuess is less than a very small number, such as 0.0001,you can claim that nextGuess is the approximated square root of n. If not, next-Guess becomes lastGuess and the approximation process continues. Implementthe following method that returns the square root of n.public static double sqrt(long n)" I have my java code here " import java.util.Scanner;public class Main2 {public static void main(String[]…arrow_forwardProblem: Construct a program that simulates the major Bank Transactions: Deposit, Withdraw and Inquire. Your program should use methods for the 3 transactions and another method for the login transaction. Below is the initial structure of the your code: public class BankTransaction { static void DisplayMenu() { /*this method displays the menu of the transactions*/ } static void withdraw(float amount) { /*this method should deduct the amount withdrawn from the current balance*/ } static void deposit(float amount) { /*this method should add the amount deposited to the current balance*/ } static float inquire() { /*this method should return the current balance*/ } static boolean CorrectPinNUmber() { /*this method should ask user to input the pin number. returns TRUE if the pin number is valid*/ It } public static void main(String a[]) { } } Design your main program to test these methods. You can design your own user interface.arrow_forward
- (3) public static void test_b(int n) { if (n>0) test_b(n-2); System.out.println(n + " "); Consider the following method: What is printed by the call test_b(4)? A. 0 2 4 B. 0 2 C. 2 4 D. 4 2 E. 4 20 3 (4) What is the efficacy class of +? n 3 A: ©(1) B: O (log n) C: O (n) D: O (n log n) E: Θ n)arrow_forwardT/F 5) The following two methods will both compute the same thing when invoked with the same value of x. That is,method1(x) = = method2(x).public int method1(int x){if(x > 0) return method1(x – 1) + 1;else return 0;}public int method2(int x){if(x > 0) return 1 + method2(x – 1);else return 0;}arrow_forward3) Write a program called AveMethod (main method) that contains a method called threeAve (method name) that computes the average of three numbers. NO CREDIT if a method is not used. (7 pnts) a) The main method will do the following: asks the user for 3 number (double type). calls the threeAve method print the average of the three numbers b) The threeAve method will do the following: receives 3 numbers (parameters) calculate the average of the three numbers returns the average ● Sample Run: Input the first number: 2.5 Input the second number: 3.5 Input the third number: 6.6 The average is 4.2 Submission ● Copy and paste your code Screen shot the console with the same Sample Run as abovearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
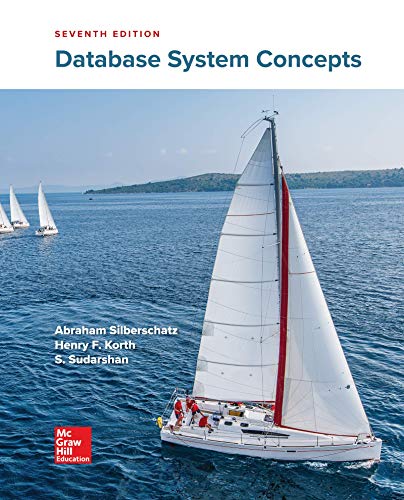
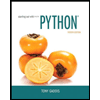
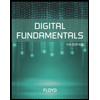
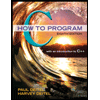
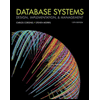
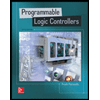