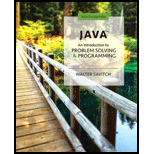
Java: An Introduction to Problem Solving and Programming (8th Edition)
8th Edition
ISBN: 9780134462035
Author: Walter Savitch
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 5, Problem 12PP
Program Plan Intro
Monkey face and hand in JavaFX Application
Program Plan:
- • Import required package.
- • Define “enum” class for monkey hand position.
- • Define “drawMonkeyFace” class.
- ○ Initializes required variables.
- ○ Define main function.
- ○ Define “drawFace” method with argument of “GraphicsContext” object, “at_XBase”, “at_YBase” and “HandPosition” object.
- ■ Invoke “strokeOval” method for object “gContext”.
- ■ Compute the monkey ear width, height and offset to draw the monkey ear.
- ■ Invoke “strokeOval” method for object “gContext”.
- ■ Compute the monkey eye width, height and offset to draw the monkey eye.
- ■ Invoke “strokeOval” method for object “gContext”.
- ■ Compute the monkey mouth width, height and offset to draw the monkey mouth.
- ■ Invoke “strokeArc” for object “gContext” which is used to draw arc.
- ■ Declare required variables for hand offset.
- ■ Check location of hand using “switch” case.
- • If the case is “MONKEY_MOUTH”, then compute hand offset for “X” and “Y” and then call “drawMonkeyHand” method.
- • If the case is “MONKEY_EYE”, then compute hand offset for “X” and “Y” and then call “drawMonkeyHand” method.
- • If the case is “MONKEY_EAR”, then compute hand offset for “X” and “Y” and then call “drawMonkeyHand” method.
- ○ Define “drawMonkeyHand” method with argument of “GraphicsContext” object, “at_XBase” and “at_YBase”.
- ■ Compute palm width and height.
- ■ Invoke “fillOval” method for object “gContext”.
- ■ Compute finger width, height of monkey and offset.
- ■ Invoke “fillOval” method for object “gContext”.
- ○ Define “start” method.
- ■ Create an object “rt” from “Group” class.
- ■ Create an object “s” from “Scene” class.
- ■ Create an object “ca” from “Canvas” class.
- ■ Create object for “GraphicsContext” class.
- ■ Draw monkey ear by calling method “drawFace”.
- ■ Set the font by using the method “setFont”.
- ■ Set text for first monkey face by using method “fillText”.
- ■ Draw monkey eye by calling method “drawFace”.
- ■ Set text for second monkey face by using method “fillText”.
- ■ Draw monkey mouth by calling method “drawFace”.
- ■ Set text for third monkey face by using method “fillText”.
- ■ Set title for given program.
- ■ Call “setScene” method for stage.
- ■ Display the all pics by using method “show”.
Expert Solution & Answer

Trending nowThis is a popular solution!

Students have asked these similar questions
Exercise
docID document text
docID document text
1
hot chocolate cocoa beans
7
sweet sugar
2345
9
cocoa ghana africa
8
sugar cane brazil
beans harvest ghana
9
sweet sugar beet
cocoa butter
butter truffles
sweet chocolate
10
sweet cake icing
11
cake black forest
Clustering by k-means, with preprocessing tokenization, term weighting TFIDF.
Manhattan Distance. Number of cluster is 2. Centroid docID 2 and docID 9.
Change the following code so that there is always at least one way to get from the left corner to the top right, but the labyrinth is still randomized.
The player starts at the bottom left corner of the labyrinth. He has to get to the top right corner of the labyrinth as fast he can, avoiding a meeting with the evil dragon. Take care that the player and the dragon cannot start off on walls. Also the dragon starts off from a randomly chosen position
public class Labyrinth { private final int size; private final Cell[][] grid;
public Labyrinth(int size) { this.size = size; this.grid = new Cell[size][size]; generateLabyrinth(); }
private void generateLabyrinth() { Random rand = new Random(); for (int i = 0; i < size; i++) { for (int j = 0; j < size; j++) { // Randomly create walls and paths grid[i][j] = new Cell(rand.nextBoolean()); } } // Ensure start and end are…
Change the following code so that it checks the following 3 conditions:
1. there is no space between each cells (imgs)
2. even if it is resized, the components wouldn't disappear
3. The GameGUI JPanel takes all the JFrame space, so that there shouldn't be extra space appearing in the frame other than the game.
Main():
Labyrinth labyrinth = new Labyrinth(10);
Player player = new Player(9, 0); Dragon dragon = new Dragon(9, 9);
JFrame frame = new JFrame("Labyrinth Game"); GameGUI gui = new GameGUI(labyrinth, player, dragon);
frame.add(gui); frame.setSize(600, 600); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setVisible(true);
public class GameGUI extends JPanel { private final Labyrinth labyrinth; private final Player player; private final Dragon dragon; //labyrinth, player, dragon are just public classes
private final ImageIcon playerIcon = new ImageIcon("data/images/player.png");…
Chapter 5 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
Ch. 5.1 - Consider the program in Listing 5.4 . Suppose you...Ch. 5.1 - Prob. 2STQCh. 5.1 - Prob. 3STQCh. 5.1 - Suppose every species in the world has a peculiar...Ch. 5.1 - Revise the definition of the method writeOutput in...Ch. 5.1 - Revise the definition of the method readInput in...Ch. 5.1 - Revise the definition of the method...Ch. 5.1 - What is the meaning of (int) that appears in the...Ch. 5.1 - Prob. 9STQCh. 5.1 - Define a method called getDensity that could be...
Ch. 5.1 - Define a method called changePopulation that could...Ch. 5.1 - Define a method called changePopulation that could...Ch. 5.2 - In Listing 5.12, we set the data for the object...Ch. 5.2 - Give preconditions and postconditions for the...Ch. 5.2 - What is an accessor method? What is a mutator...Ch. 5.2 - Give the complete definition of a class called...Ch. 5.2 - Prob. 17STQCh. 5.2 - In the definition of the method in Listing 5.15,...Ch. 5.2 - What is a well-encapsulated class definition?Ch. 5.2 - When should an instance variable in a class...Ch. 5.2 - Prob. 21STQCh. 5.2 - In a class definition, is anything private ever...Ch. 5.2 - In a class definition, is the body of any method...Ch. 5.3 - What is a reference type? Are class types...Ch. 5.3 - When comparing two quantities of a class type to...Ch. 5.3 - Prob. 26STQCh. 5.3 - Write a method definition for a method called...Ch. 5.3 - Given the class Species as defined in Listing...Ch. 5.3 - After correcting the program in the previous...Ch. 5.3 - What is the biggest difference between a parameter...Ch. 5.3 - Prob. 31STQCh. 5.3 - Write an equals method for the class Person...Ch. 5.4 - Rewrite the method drawFaceSansMouth in Listing...Ch. 5 - Design a class to represent a credit card. Think...Ch. 5 - Repeat Exercise 1 for a credit card account...Ch. 5 - Repeat Exercise 1 for a coin instead of a credit...Ch. 5 - Repeat Exercise 1 for a collection of coins...Ch. 5 - Consider a Java class that you could use to get an...Ch. 5 - Consider a class that keeps track of the sales of...Ch. 5 - Consider a class MotorBoat that represents...Ch. 5 - Prob. 8ECh. 5 - Prob. 9ECh. 5 - Prob. 10ECh. 5 - Write a program to answer questions like the...Ch. 5 - Define a class called Counter. An object of this...Ch. 5 - Prob. 3PCh. 5 - Define a Trivia class that contains information...Ch. 5 - Define a Beer class that contains the following...Ch. 5 - Write a grading program for an instructor whose...Ch. 5 - Add methods to the Person class from Self-Test...Ch. 5 - Create a class that represents a grade...Ch. 5 - Write a program that uses the Purchase class in...Ch. 5 - Write a program to answer questions like the...Ch. 5 - Consider a class that could be used to play a game...Ch. 5 - Consider a class BasketballGame that represents...Ch. 5 - Consider a class ConcertPromoter that records the...Ch. 5 - Prob. 9PPCh. 5 - Consider a class Movie that contains information...Ch. 5 - Repeat Programming Project 18 from Chapter 4, but...Ch. 5 - Prob. 12PP
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Make the following game user friendly with GUI, with some simple graphics. The GUI should be in another seperate class, with some ImageIcon, and Game class should be added into the pane. The following code works as this: The objective of the player is to escape from this labyrinth. The player starts at the bottom left corner of the labyrinth. He has to get to the top right corner of the labyrinth as fast he can, avoiding a meeting with the evil dragon. The player can move only in four directions: left, right, up or down. There are several escape paths in all labyrinths. The player’s character should be able to moved with the well known WASD keyboard buttons. If the dragon gets to a neighboring field of the player, then the player dies. Because it is dark in the labyrinth, the player can see only the neighboring fields at a distance of 3 units. Cell Class: public class Cell { private boolean isWall; public Cell(boolean isWall) { this.isWall = isWall; } public boolean isWall() { return…arrow_forwardDiscuss the negative and positive impacts or information technology in the context of your society. Provide two references along with with your answerarrow_forwardA cylinder of diameter 10 cm rotates concentrically inside another hollow cylinder of inner diameter 10.1 cm. Both cylinders are 20 cm long and stand with their axis vertical. The annular space is filled with oil. If a torque of 100 kg cm is required to rotate the inner cylinder at 100 rpm, determine the viscosity of oil. Ans. μ= 29.82poisearrow_forward
- Make the following game user friendly with GUI, with some simple graphics The following code works as this: The objective of the player is to escape from this labyrinth. The player starts at the bottom left corner of the labyrinth. He has to get to the top right corner of the labyrinth as fast he can, avoiding a meeting with the evil dragon. The player can move only in four directions: left, right, up or down. There are several escape paths in all labyrinths. The player’s character should be able to moved with the well known WASD keyboard buttons. If the dragon gets to a neighboring field of the player, then the player dies. Because it is dark in the labyrinth, the player can see only the neighboring fields at a distance of 3 units. Cell Class: public class Cell { private boolean isWall; public Cell(boolean isWall) { this.isWall = isWall; } public boolean isWall() { return isWall; } public void setWall(boolean isWall) { this.isWall = isWall; } @Override public String toString() {…arrow_forwardPlease original work What are four of the goals of information lifecycle management think they are most important to data warehousing, Why do you feel this way, how dashboards can be used in the process, and provide a real life example for each. Please cite in text references and add weblinksarrow_forwardThe following is code for a disc golf program written in C++: // player.h #ifndef PLAYER_H #define PLAYER_H #include <string> #include <iostream> class Player { private: std::string courses[20]; // Array of course names int scores[20]; // Array of scores int gameCount; // Number of games played public: Player(); // Constructor void CheckGame(int playerId, const std::string& courseName, int gameScore); void ReportPlayer(int playerId) const; }; #endif // PLAYER_H // player.cpp #include "player.h" #include <iomanip> Player::Player() : gameCount(0) {} void Player::CheckGame(int playerId, const std::string& courseName, int gameScore) { for (int i = 0; i < gameCount; ++i) { if (courses[i] == courseName) { // If course has been played, then check for minimum score if (gameScore < scores[i]) { scores[i] = gameScore; // Update to new minimum…arrow_forward
- In this assignment, you will implement a multi-threaded program (using C/C++) that will check for Prime Numbers and Palindrome Numbers in a range of numbers. Palindrome numbers are numbers that their decimal representation can be read from left to right and from right to left (e.g. 12321, 5995, 1234321). The program will create T worker threads to check for prime and palindrome numbers in the given range (T will be passed to the program with the Linux command line). Each of the threads works on a part of the numbers within the range. Your program should have some global shared variables: • numOfPrimes: which will track the total number of prime numbers found by all threads. numOfPalindroms: which will track the total number of palindrome numbers found by all threads. numOfPalindromic Primes: which will count the numbers that are BOTH prime and palindrome found by all threads. TotalNums: which will count all the processed numbers in the range. In addition, you need to have arrays…arrow_forwardHow do you distinguish between hardware and a software problem? Discuss theprocedure for troubleshooting any hardware or software problem. give one reference with your answer.arrow_forwardYou are asked to explain what a computer virus is and if it can affect computer’shardware or software. How do you protect your computer against virus? give one reference with your answer.arrow_forward
- Distributed Systems: Consistency Models fer to page 45 for problems on data consistency. structions: Compare different consistency models (e.g., strong, eventual, causal) for distributed databases. Evaluate the trade-offs between availability and consistency in a given use case. Propose the most appropriate model for the scenario and explain your reasoning. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440AZF/view?usp=sharing]arrow_forwardOperating Systems: Deadlock Detection fer to page 25 for problems on deadlock concepts. structions: • Given a system resource allocation graph, determine if a deadlock exists. If a deadlock exists, identify the processes and resources involved. Suggest strategies to prevent or resolve the deadlock and explain their trade-offs. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440 AZF/view?usp=sharing]arrow_forwardArtificial Intelligence: Heuristic Evaluation fer to page 55 for problems on Al search algorithms. tructions: Given a search problem, propose and evaluate a heuristic function. Compare its performance to other heuristics based on search cost and solution quality. Justify why the chosen heuristic is admissible and/or consistent. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440 AZF/view?usp=sharing]arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
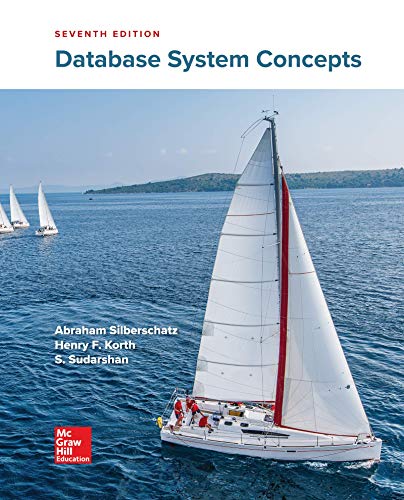
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
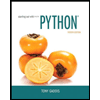
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
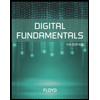
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
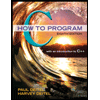
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
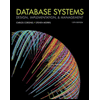
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
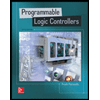
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education