What is JavaScript?
JavaScript (or js) is a scripting language used to script (program) web pages. It is used to make the web page dynamic and interactive. JavaScript codes are written in a web page's hypertext markup language (HTML) code and run automatically when the page loads. JavaScript codes do not require any particular application (app) or software for compilation or execution.
Components of JavaScript
ECMAScript (client-side script)
ECMAScript is a scripting language. It is used to standardize the application of JavaScript. This part provides all the core functionality required in JavaScript code.
Document object model (DOM)
It is an application programming interface (API) used to manipulate HTML and extensible markup language (XML) documents. It represents a document in the form of a tree node.
Browser object model (BOM)
It is the central part of JavaScript programming on the internet. It offers objects that expose the web browser’s functionality.
JavaScript engine
JavaScript engine is a program that converts the JavaScript code into machine-level language. It takes the JavaScript program as an input and converts it into binary digits (CPU-understandable language). Later, the interpreter interprets and processes the code. JavaScript engine is usually inbuilt in modern web browsers.
JavaScript runtime environment
JavaScript engine does not run individually. It runs within the JavaScript runtime environment. In other words, the JavaScript program executes within the runtime environment.
Applications of JavaScript
Since JavaScript is a widely-used programming language, it has various applications. These include:
Client-side validation
With JavaScript, the inputs provided by the user can be easily validated before updating them to the server.
HTML pages manipulation
Adding and deleting HTML tags or modifying HTML content becomes easy with JavaScript.
Add user notifications
Elements such as dynamic pop-ups can be added to web pages with JavaScript.
Loading of Back-end data
The Ajax library of JavaScript helps load the backend data, which enhances the user experience.
Presentations
JavaScript presents the data on the web page beautifully to give the website a striking look and feel.
How to run a JavaScript code
JavaScript codes need a browser for execution. The code is written within an HTML page and when the user requests the HTML page, the JavaScript code is sent to the browser. The browser is then responsible for its execution. Further, all modern web browsers support JavaScript. Hence, regardless of the web browser a visitor uses, JavaScript can be supported.
Fundamentals of a JavaScript code
Like every other programming language, JavaScript has its specifications to be followed while writing a code. Below are the JavaScript fundamentals every programmer needs to know:
Syntax and Case-sensitivity
JavaScript is a case-sensitive language. That means all the function names, class names, variables, and operators are case-sensitive. For instance, the variables myhome and Myhome are different. Apart from this, identifiers, expressions, comments, and statements are used in JavaScript code.
Identifiers
Names of variables, functions, parameters, or classes are called identifiers in JavaScript. They should fulfill the following criteria:
- The first character of an identifier should be a letter (a-z, A-Z), underscore (_), or a dollar sign ($).
- The rest of the characters can be letters (a-z, A-Z), underscore(_), and dollar signs ($).
Generally, the camel case is used for identifiers. Examples of identifiers are counter, inArray, beginWith.
Comments
Comments can be single-line or block-line. Single-line comments are preceded by double forward-slash (//).
For instance: //this is a comment
A forward slash and asterisk precede a block-line comment (/*) and end with an asterisk and backward slash (*/). For instance:
/*
This is a block line comment
With multiple lines
*/
Statements
In JavaScript, adding a semicolon at the end of a statement is not necessary but it is suggested to use a semicolon to make the code more readable. For instance, var a = 25; is a statement.
Further, for multiple statements, the code block is preceded by a left curly bracket ({) and ends with a right curly bracket (}). For instance,
if(x < y) {
console.log(‘x is less than y’);
return 1;
}
Expressions
An expression is a code that evaluates (solves) a value. For instance, 3 + 4
Keywords and reserved words
Keywords and reserved words have specific uses. They cannot be used as identifiers. Some of the widely used keywords in JavaScript are for, break, class, while, if, double, catch, try, function, public, float, and continue.
Variables
In JavaScript, variables are used to hold values of any type of data. They are placeholders for values and declared with the var keyword. They can be local or global variables. Local variables exist only within a function (method), whereas global variables are declared outside a function and accessible throughout the program.
Examples of variables are:
- var a
- var message
Data types
JavaScript primarily consists of the following data types:
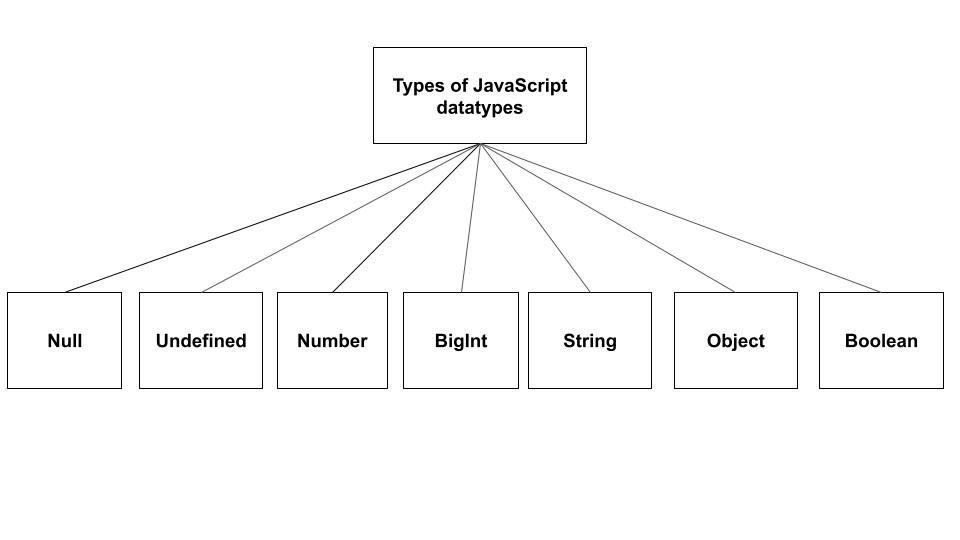
- Null - The null data type consists of only one value null, which indicates an empty object pointer. It is a second primitive data type. Example: if (a ==null)
- Number - The number data type includes integer and float values. Integer values hold an integer, whereas float values hold decimal and floating-point values. Example: let a = 35
- String - String contains zero or more character sequences. It either begins and ends with a single quote (‘) or double quote (“). It is worth noting that a string that begins with a single quote ends with a single quote, and if it begins with a double quote, it should end with a double quote. Example: let name = “ABC”
- Object - Object is a collection of properties. Each property is classified as a key-value pair. Example: const human = {firstName:"Mark", lastName:"Robert", age:40};
- Boolean - A boolean data type has only two values, that is, true or false. Example: isPending = true
- Undefined – If a variable is not assigned a value, it is undefined. Example: var message
- BigInt – It represents integers with arbitrary precision. BigInt data type is created by adding n to the end of the integer. Example: const x = 2n ** 53n
Conditional statements in JavaScript
In JavaScript, conditional statements decide whether the code should be executed or not. It performs the action based on the condition specified. The different types of conditional statements in JavaScript are:
- if – It executes the code block when the condition is true
- else – It executes the code block when the condition is false
- else if – Here a new condition can be specified. The code written in this block will be executed if the above condition is false.
- switch – This statement is used to specify alternative code blocks.
Operators in JavaScript
JavaScript operators are symbols used to apply arithmetic or logic operations on operands. JavaScript has different types of operators such as arithmetic, logic, bitwise, comparison, assignment, and special operators.
Examples of operators are addition (+), multiplication (*), OR(|), AND(&), less than(<).
Context and Applications
The concept of JavaScript fundamentals are essential for professional web programmers and students studying for:
- Bachelors and Masters in Computer Applications
- Bachelors and Masters in Technology (Computer Science)
Practice Problems
1. Which of these is not a data type in JavaScript?
- Undefined
- BigInt
- Null
- Float
Answer: Option d
Explanation: JavaScript contains the following datatypes – number, Boolean, null, BigInt, undefined, string, and object.
2. Under which HTML tag is JavaScript written?
- <step-by-step>
- <self-paced>
- <script>
- <meta>
Answer: Option c
Explanation: The JavaScript code is written under the <script> tag in an HTML document.
3. Which of these is true about JavaScript?
- It is a full-stack implementation language.
- JavaScript has function expressions called fields.
- JavaScript is a scripting language.
- JavaScript has an eloquent JavaScript keyword named strict mode.
Answer: Option c
Explanation: Unlike Java, C++, and other programming languages, JavaScript is used to program webpages. Hence, it is a scripting programming language.
4. Which of the following is not a type of JavaScript operator?
- Arithmetic operator
- Logic operator
- String
- Bitwise operator
Answer: Option c
Explanation: String is a data type in JavaScript whereas arithmetic, logic, bitwise, conditional, and assignment operators are types of operators in JavaScript.
5. Which of the following keywords is used to define a function in JavaScript language?
- main loops
- function
- int
- basics
Answer: Option b
Explanation: The function keyword is used to define functions in JavaScript. After the keyword, the function name and parentheses are added to the declaration. For instance, function circle().
Common Mistakes
JavaScript and Java programming languages are often considered similar. However, it is not true. JavaScript is a scripting language used for programming (scripting) web pages, whereas Java is an object-oriented programming language used in software and app development.
Related Concepts
- JavaScript prototypes
- JavaScript operators
- JavaScript control flow
- JavaScript objects
- JavaScript arrow function
Want more help with your computer science homework?
*Response times may vary by subject and question complexity. Median response time is 34 minutes for paid subscribers and may be longer for promotional offers.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.
Fundamentals of Javascript Homework Questions from Fellow Students
Browse our recently answered Fundamentals of Javascript homework questions.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.