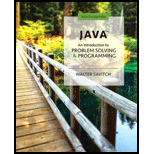
Write a program that asks the user to enter the size of a triangle (an integer from 1 to 50). Display the triangle by writing lines of asterisks. The first line will have one asterisk, the next two, and so on. with each line having one more asterisk than the previous line, up to the number entered by the user. On the next line write one fewer asterisk and continue by decreasing the number of asterisks by 1 for each successive line until only one asterisk is displayed. (Hint: Use nested for loops; the outside loop controls the number of lines to write, and the inside loop controls the number of asterisks to display on a line.) For example, if the user enters 3, the output would be
*
**
***
**
*

Want to see the full answer?
Check out a sample textbook solution
Chapter 4 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
Additional Engineering Textbook Solutions
Introduction To Programming Using Visual Basic (11th Edition)
Thinking Like an Engineer: An Active Learning Approach (4th Edition)
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Concepts Of Programming Languages
SURVEY OF OPERATING SYSTEMS
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
- Write a program that prompts the user to enter apoint (x, y) and checks whether the point is within the rectangle centred at (1, 1)with width 10 and height 5. For example, (2, 2) is inside the rectangle and (6, 4)is outside the rectangle, as shown in Figure . (Hint: A point is in the rectangleif its horizontal distance to (0, 0) is less than or equal to 10 / 2 and its verticaldistance to (0, 0) is less than or equal to 5.0 / 2. Test your program to cover allcases.) Here are two sample runs. Enter a point with two coordinates: 2 2 ↵EnterPoint (2.0, 2.0) is in the rectanglearrow_forwardWe need to use the java languagearrow_forwardWrite a program that reads from the user a character ('q' or 'c'). If the character is 'c', the program reads from the user the radius rof a circle and prints its area. • If the user enters 'q', the program asks the user to enter the length and width of a quadrilateral. We assume the quadrilateral is either a square or rectangle. You should print if the quadrilateral is square or rectangle. • Otherwise, it prints "Wrong character" PS: Use the following formulas: area of circle=3.14*r Sample Run1: Enter a character (q or c): q Enter the Length and Width: 80 150 |It is a rectangle Sample Run2: Enter a character (q or c): q Enter the Length and Width: 80 80 It is a square Sample Run3: Enter a character (q or c): c Enter the radius: 2 The area of the circle is 12.56 Sample Run4: Enter a character (q or c): d Wrong characterarrow_forward
- Use Python to write a program that asks the user to enter a pocket number and displays whether the pocket is green, red, or black. The program should display an error message if the user enters a number that is outside the range of 0 through 56. On a roulette wheel, the pockets are numbered from 0 to 36. The colors of the pockets are as follows: Pocket 0 is green. For pockets 1 through 20, the odd-numbered pockets are red and the even-numbered pockets are black. For pockets 21 through 38, the odd-numbered pockets are black and the even-numbered pockets are red. For pockets 39 through 48, the odd-numbered pockets are red and the even-numbered pockets are black. For pockets 49 through 56, the odd-numbered pockets are black and the even-numbered pockets are red.arrow_forwardWrite a program that lets the user guess whether a randomly generated integer would be even or odd. The program randomly generates an inte- ger and divides it by 2. The integer is even if the remainder is 0, otherwise odd. The program prompts the user to enter a guess and reports whether the guess is correct or incorrect.arrow_forwardWrite a program that generates a random number in the range of 1 through 1000, and asks the user to guess what the number is. If the user guess is higher than the random number, the program should display Too high, try again. If the users guess is lower than the random number, the program should display Too low, try again. If the user guesses the number, the application should congratulate the user and generate a new random number so the game can start over. If the user doesn't want to continue the game the user should type an appropriate stop symbol to terminate the program (such stop conditions were discussed in the class). For each game iteration, count the number of guesses made by the user and display them. The program should contain the following functions: check_guess(): this function checks if the user's guess is lower, higher or equal to the random number. random_gen(): function that generates one random number for one game iteration main(): the function where the program…arrow_forward
- Write a program that lets the user guess whether a flipped coin displays the head or the tail. The program randomly generates an integer 0 or1, which represents head or tail. The program prompts the user to enter a guess and reports whether the guess is correct or incorrect.arrow_forwardWrite a program that generates a plate number based on the user's answer to the following questions: 1. What is your favorite color of the rainbow (ROYGVIB)? a. Use the first letter of the chosen color as the first character of the plate number. b. Display "Invalid Input" and ask the user to re-enter his/her answer if the answer is not a valid color of the rainbow. 2. What is your month of birth? a. Use the last letter of the month as the second character of the plate number. b. Display "Invalid Input" and ask the user to re-enter his/her answer if the answer is not a valid month. 3. What is your first name? a. Use the third letter of the first name as the third character of the plate number. No need to include the second name if you have a second name. Make sure to use "cout" and "cin" to collect the necessary inputs from the user. For the number section: 1. Ask the user his/her age and use the age as the first and second digits of the plate number. a. Display "Invalid Input" and ask…arrow_forwardWrite a program that prompts the user to enter the number of students and each student’s name and score, and finally displays the names of the students with the lowest and secondlowest scores.arrow_forward
- Write a program that promotes the user to enter the number of students and each student's name and score ,and finally displays the student with the highest score and the second highest score.arrow_forwardTo make telephine numbers easier to remember, some companies use letters to show their telephone number. For example, the telephine number 438-5626 can be shown as GET-LOAN. In some cases, to make a telephone number meaningful, compaines might use more than seven letters. For example, 225-5466, can be displayed as CALL-HOME, whic uses eight letters. Write a program that prompts the user to enter a telephone number expressesd in letters and outputs the corresponding telephone number in digits. If the user enters more than seven letter, then process only the first seven letters. Also, output the -(hyphen) after the third digit. Allow the user to use uppercase and lowercase letters, as well as spaces between words. Moreover, your program should proces as many telephone as the user wants. (Hint: You can read the entered telephine number as a string and then use the charAt() to extract each character.)arrow_forwardwrite a program that asks the user for the length and width of a rectangle, the program should calculate and display the rectangle’s perimeter (2 x length) + (2 x width) and width (length x width). Round the circumference and area to 2 decimal places.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
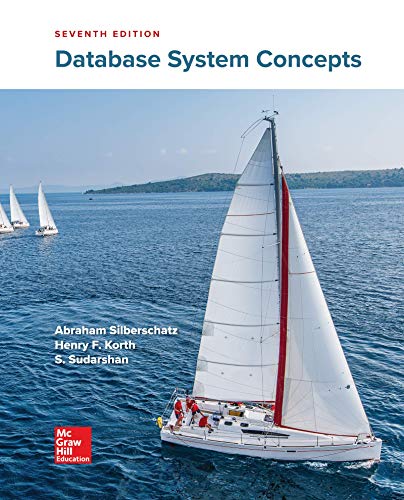
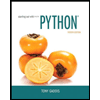
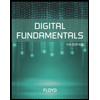
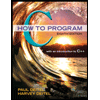
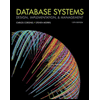
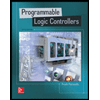