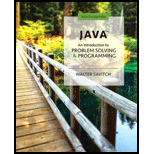
Concept explainers
Write a program that reads a bank account balance and an interest rate and displays the value of the account in ten years. The output should show the value of the account for three different methods of compounding interest: annually, monthly, and daily. When compounded annually, the interest is added once per year at the end of the year. When compounded monthly, the interest is added 12 times per year. When computed daily, the interest is added 365 times per year. You do not have to worry about leap years; assume that all years have 365 days. For annual interest, you can assume that the interest is posted exactly one year from the date of deposit. In other words, you do not have to worry about interest being posted on a specific day of the year, such as December 31. Similarly, you can assume that monthly interest is posted exactly one month after it is deposited. Since the account earns interest on the interest, it should have a higher balance when interest is posted more frequently. Be sure to adjust the interest rate for the time period of the interest. If the rate is 5 percent, you use 5/12 percent when posting monthly interest and 5/365 percent when posting daily interest. Perform this calculation using a loop that adds in the interest for each time period, that is, do not use some sort of algebraic formula. Your program should have an outer loop that allows the user to repeat this calculation for a now balance and interest rate. The calculation is repeated until the user asks to end the program.

Want to see the full answer?
Check out a sample textbook solution
Chapter 4 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
Additional Engineering Textbook Solutions
Elementary Surveying: An Introduction To Geomatics (15th Edition)
Web Development and Design Foundations with HTML5 (8th Edition)
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Starting Out With Visual Basic (8th Edition)
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
Starting Out with C++ from Control Structures to Objects (9th Edition)
- Write a program that reads the employee's name and base salary and then calculates the tax and net amount Salary where: Net salary = Basic salary If the basic salary is less than 500 (no tax) Net salary = basic salary - tax value So that the tax amount is 5% of the basic salary Then it prints the employee's name and net salary %3D 2:40 PM /arrow_forwardCan answer the task below?arrow_forwardWrite a program that can be used to calculate the federal tax. The tax is calculated as follows: For single people, the standard exemption is $4,000; for married people, the standard exemption is $7,000. A person can also put up to 6% of his or her gross income in a pension plan. The tax rates are as follows: If the taxable income is: Between $0 and $15,000, the tax rate is 15%. Between $15,001 and $40,000, the tax is $2,250 plus 25% of the taxable income over $15,000. Over $40,000, the tax is $8,460 plus 35% of the taxable income over $40,000. Prompt the user to enter the following information: Marital status If the marital status is “married,” ask for the number of children under the age of 14 Gross salary (If the marital status is “married” and both spouses have income, enter the combined salary.) Percentage of gross income contributed to a pension fund Your program must consist of at least the following functions: Function getData: This function asks the user to…arrow_forward
- Write a program that computes and displays the charges for a patient’s hospital stay. First, the program should ask if the patient was admitted as an in-patient or an out-patient. If the patient was an in-patient the following data should be entered: • The number of days spent in the hospital• The daily rate• Charges for hospital services (lab tests, etc.)• Hospital medication charges.If the patient was an out-patient the following data should be entered:• Charges for hospital services (lab tests, etc.)• Hospital medication charges.Use a single, separate function to validate that no input is less than zero. If it is, it should be re-entered before being returned.Once the required data has been input and validated, the program should use two overloaded functions to calculate the total charges. One of the functions should accept arguments for the in-patient data, while the other function accepts arguments for out- patient data. Both functions should return the total charges.arrow_forwardWrite a program that generates a plate number based on the user's answer to the following questions: 1. What is your favorite color of the rainbow (ROYGVIB)? a. Use the first letter of the chosen color as the first character of the plate number. b. Display "Invalid Input" and ask the user to re-enter his/her answer if the answer is not a valid color of the rainbow. 2. What is your month of birth? a. Use the last letter of the month as the second character of the plate number. b. Display "Invalid Input" and ask the user to re-enter his/her answer if the answer is not a valid month. 3. What is your first name? a. Use the third letter of the first name as the third character of the plate number. No need to include the second name if you have a second name. Make sure to use "cout" and "cin" to collect the necessary inputs from the user. For the number section: 1. Ask the user his/her age and use the age as the first and second digits of the plate number. a. Display "Invalid Input" and ask…arrow_forwardWrite a program that reads the amount of a monthly mortgage payment andthe amount still owed—the outstanding balance—and then displays theamount of the payment that goes to interest and the amount that goes toprincipal (i.e., the amount that goes to reducing the debt). Assume that theannual interest rate is 7.49 percent. Use a named constant for the interest rate.Note that payments are made monthly, so the interest is only one twelfth ofthe annual interest of 7.49 percent.arrow_forward
- Write a program that computes the molecular weight of a carbohydrate (ingrams per mole) based on the number of hydrogen, carbon, and oxygenatoms in the molecule. The program should prompt the user to enter thenumber of hydrogen atoms, the number of carbon atoms, and the numberof oxygen atoms. The program then prints the total combined molecularweight of all the atoms based on these individual atom weights:Atom Weight(grams I mole)H 1.00794c 12.01070 15.9994For example, the molecular weight of water (H20) is: 2(1.00794) +15.9994 = 18.01528.arrow_forwardWrite a program that reads from the user a character ('q' or 'c'). If the character is 'c', the program reads from the user the radius rof a circle and prints its area. • If the user enters 'q', the program asks the user to enter the length and width of a quadrilateral. We assume the quadrilateral is either a square or rectangle. You should print if the quadrilateral is square or rectangle. • Otherwise, it prints "Wrong character" PS: Use the following formulas: area of circle=3.14*r Sample Run1: Enter a character (q or c): q Enter the Length and Width: 80 150 |It is a rectangle Sample Run2: Enter a character (q or c): q Enter the Length and Width: 80 80 It is a square Sample Run3: Enter a character (q or c): c Enter the radius: 2 The area of the circle is 12.56 Sample Run4: Enter a character (q or c): d Wrong characterarrow_forwardA parking garage charges a $2.00 minimum fee to park for up to three hours and an additional $1 per hour for each hour or part thereof over four hours. The maximum charge for any given 24-hour period is $10.00. Assume that no car parks for longer than 24 hours at a time. Write a program that will calculate and print the parking charges for each of three customers who parked their cars in this garage yesterday. You should enter the hours parked for each customer. Your program should print the results in a neat tabular format, and should calculate and print the total of yesterday's receipts. The program should use the function calculate Charges to determine the charge for each customer.arrow_forward
- A mobile phone service provider has three different subscription packages for its customers: Package A: For $39.99 per month, 450 minutes are provided. Additional minutes are $0.45 per minute. Package B: For $59.99 per month, 900 minutes are provided. Additional minutes are $0.40 per minute. Package C: For $69.99 per month, unlimited minutes are provided. Write a program that calculates a customer’s monthly bill. It should ask the user to enter the letter of the package the customer has purchased (A, B, or C) and the number of minutes that were used. The program should display the total charges.arrow_forwardWrite a program that reads from the user a character (*q' or 'c'). If the character is 'c', the program reads from the user the radius r of a circle and prints its area. If the user enters 'q', the program asks the user to enter the length and width of a quadrilateral. We assume the quadrilateral is either a square or rectangle. You should print if the quadrilateral is square or rectangle. Otherwise, it prints "Wrong character" PS: Use the following formulas : area of circle=3.14*r? Iarrow_forwardPlease write a program which prints out the following story. The user gives a name and a year, which should be inserted into the printout. Please type in a name: Mary Please type in a year: 1572 Mary is a valiant knight, born in the year 1572. One morning Mary woke up to an awful racket: a dragon was approaching the village. Only Mary could save the village's residents. The story should change according to the input given by the user. Sample output 1arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
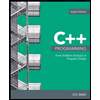