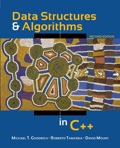
Explanation of Solution
Program code:
//include the required header files
#include<iostream>
#include<cstdio>
#include<cstdlib>
/* Node Declaration*/
using namespace std;
//create a structure
struct node
{
//structure members
int info;
struct node *next;
struct node *prev;
}*start;
/*Class Declaration */
class double_llist
{
//public access modifier
public:
//declare the member functions
void create_list(int value);
void add_begin(int value);
void add_after(int value, int position);
void delete_element(int value);
void search_element(int value);
void display_dlist();
void count();
void reverse();
//define a method
double_llist()
{
//set the value of start
start = NULL;
}
};
/* Main*/
int main()
{
//declare the required variables
int choice, element, position;
double_llist dl;
//iterate a while loop
while (1)
{
//display the choices
cout<<endl<<"----------------------------"<<endl;
cout<<endl<<"Operations on Doubly linked list"<<endl;
cout<<endl<<"----------------------------"<<endl;
cout<<"1.Create Node"<<endl;
cout<<"2.Add at begining"<<endl;
cout<<"3.Add after position"<<endl;
cout<<"4.Delete"<<endl;
cout<<"5.Display"<<endl;
cout<<"6.Count"<<endl;
cout<<"7.Reverse"<<endl;
cout<<"8.Quit"<<endl;
//prompt the user to enter the choice
cout<<"Enter your choice : ";
cin>>choice;
//switch cases
switch ( choice )
{
//case 1
case 1:
//prompt the user to enter the element
cout<<"Enter the element: ";
//scan for the value
cin>>element;
//call the method create_list(element)
dl.create_list(element);
//new line
cout<<endl;
//break fron the case
break;
case 2:
//prompt the user to enter the element
cout<<"Enter the element: ";
//scan for the value
cin>>element;
//call the method add_begin(element)
dl.add_begin(element);
//new line
cout<<endl;
//break fron the case
break;
case 3:
//prompt the user to enter the element
cout<<"Enter the element: ";
//scan for the value
cin>>element;
//prompt the user to enter the position
cout<<"Insert Element after postion: ";
cin>>position;
//call the method add_after(element)
dl.add_after(element, position);
//new line
cout<<endl;
//break fron the case
break;
case 4:
//if the condition is true
if (start == NULL)
{
//print the statement
cout<<"List empty,nothing to delete"<<endl;
//break fron the condition
break;
}
//prompt the user to enter the element
cout<<"Enter the element for deletion: ";
//scan for the value
cin>>element;
//call the method delete_element(element)
dl.delete_element(element);
//new line
cout<<endl;
//break fron the case
break;
case 5:
//call the method display_dlist(element)
dl.display_dlist();
//new line
cout<<endl;
//break fron the case
break;
case 6:
//call the method count(element)
dl.count();
//break fron the case
break;
case 7:
//if the condition is true
if (start == NULL)
{
//print the statement
cout<<"List empty,nothing to reverse"<<endl;
//break fron the condititon
break;
}
//call the method reverse(element)
dl.reverse();
//new line
cout<<endl;
//break fron the case
break;
case 8:
//exit from while loop
exit(1);
default:
//print the statement
cout<<"Wrong choice"<<endl;
}
}
//return zero
return 0;
}
/* Create Double Link List*/
void double_llist::create_list(int value)
{
//create struct vaiable
struct node *s, *temp;
//set the value of temp
temp = new(struct node);
//insert the value
temp->info = value;
//set next as Null
temp->next = NULL;
//if the condition is true
if (start == NULL)
{
//set prev equal to Null
temp->prev = NULL;
//set start equal to temp
start = temp;
}
else
{
//set s equal to start
s = start;
//iterate a while loop
while (s->next != NULL)
//assign the value
s = s->next;
s->next = temp;
temp->prev = s;
}
}
/* Insertion at the beginning */
void double_llist::add_begin(int value)
{
//if the condition is true
if (start == NULL)
{
//print the statement
cout<<"First Create the list."<<endl;
return;
}
//inserting the element
struct node *temp;
temp = new(struct node);
temp->prev = NULL;
temp->info = value;
temp->next = start;
start->prev = temp;
start = temp;
cout<<"Element Inserted"<<endl;
}
/* Insertion of element at a particular position*/
void double_llist::add_after(int value, int pos)
{
//if the condition is true
if (start == NULL)
{
//print the statement
cout<<"First Create the list."<<endl;
//return
return;
}
//create struct variables
struct node *tmp, *q;
//create the required variables
int i;
q = start;
//iterate a for loop
for (i = 0;i < pos - 1;i++)
{
//set the value of q
q = q->next;
//if the value of q equal to null
if (q == NULL)
{
//print the statement
cout<<"There are less than ";
cout<<pos<<" elements...

Want to see the full answer?
Check out a sample textbook solution
Chapter 3 Solutions
Data structures and algorithms in C++
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
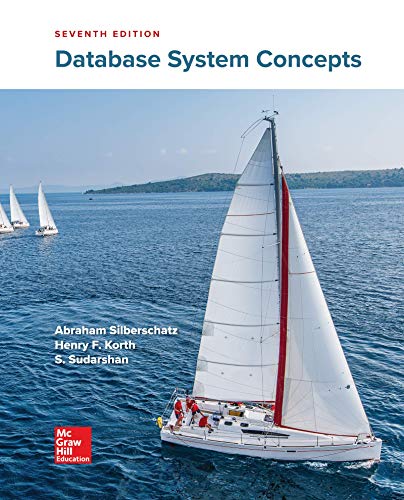
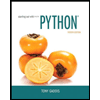
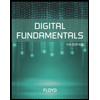
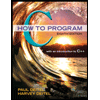
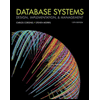
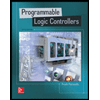