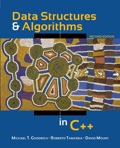
Explanation of Solution
//Swapping in singly Linked List
void swappingNodes(Node **head, Node* x, Node* y)
{
//if both nodes are same
if (x == y) return;
// Search for y (keep track of previous of node y and Current node y )
Node *prevY = NULL, *currentY = *head;
while (currentY && currentY!= y)
{
prevY = currentY;
currentY = currentY->next;
}
// Search for x (keep track of previous of node x and Current node x )
Node *prevX = NULL, *currentX = *head;
while (currentX && currentX != x)
{
prevX = currentX;
currentX = currentX->next;
}
// If either x or y is not present in the list
if (currentX == NULL || currentY == NULL)
return;
// If x is not head of linked list
if (prevX != NULL)
prevX->next = currentY;
// Else make y as new head
else
*head = currentY;
// If y is not head of linked list
if (prevY != NULL)
prevY->next = currentX;
// Else make x as new head
else
*head = currentX;
// Swap next pointers
Node *temp = currentY->next;
currentY->next = currentX->next;
currentX->next = temp;
}
Explanation:
The above algorithm is used to swap two nodes of a single linked list...
Explanation of Solution
Algorithm to swap two nodes “x” and “y” in a doubly linked list:
//swapping nodes in doubly linked list
void swapingNodes(struct Node* x, struct Node* y)
{
if ( x->prev != NULL)
{
x->prev->next = y;
}
Else
{
head = y;
}
if ( y->next !=NULL)
{
y->next->prev = x;
}
//swapping pointers
x->next = y->next;
y->p...
Explanation of Solution
Algorithm that takes more time:
The singly linked list takes more time than doubly...

Want to see the full answer?
Check out a sample textbook solution
Chapter 3 Solutions
Data structures and algorithms in C++
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
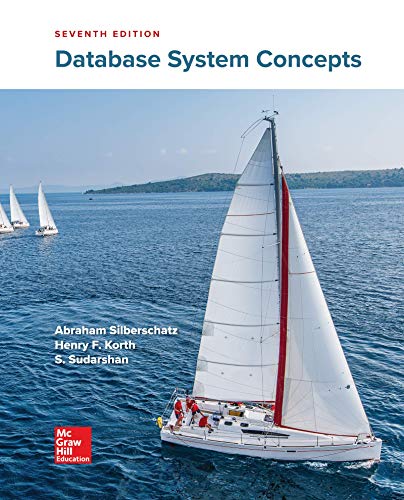
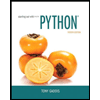
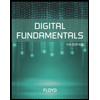
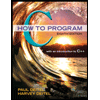
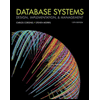
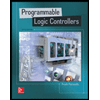