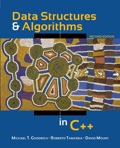
Concept explainers
Explanation of Solution
Program code:
//include the required header files
#include <stdio.h>
#include <stdlib.h>
//Basic structure of node
struct node
{
//structure members
int data;
struct node * next;
};
// Function declaration
void createList(struct node **head, int n);
void displayList(struct node *head);
int count(struct node *head);
//define the main() function
int main()
{
//declare the required variables
int n, choice;
//set the head as null
struct node *head = NULL;
//iterate a while loop
while(choice != 0)
{
//prompt the choices
printf("--------------------------------------------\n");
printf(" CIRCULAR LINKED LIST PROGRAM \n");
printf("--------------------------------------------\n");
printf("1. Create List\n");
printf("2. Display list\n");
printf("3. Count nodes\n");
printf("0. Exit\n");
printf("--------------------------------------------\n");
//prompt the user to enter the choice
printf("Enter your choice : ");
//scan for the value
scanf("%d", &choice);
//switch cases
switch(choice)
{
//if the choice is 1
case 1:
//prompt the user to enter the number of nodes to create
printf("Enter total node to create: ");
//scan for the value
scanf("%d", &n);
//call the method createList()
createList(&head, n);
//break the case
break;
//if the choice is 2
case 2:
//call the method displayList()
displayList(head);
// Hold screen
getchar();
getchar();
//break the case
break;
case 3:
//print the number of nodes
printf("Total nodes = %d\n", count(head));
// Hold screen
getchar();
getchar();
//break the case
break;
case 0:
//print the statement
printf("Exiting from application");
exit(0);
//break from the loop
break;
default:
//print the statement
printf("Error! Invalid choice. Please choose between 0-3");
}
//print new line
printf("\n\n\n\n\n");
}
//return
return 0;
}
//define the function count()
int count(struct node *head)
{
//declare the required variables
int total = 0;
struct node *current = head;
// Iterate till end of list
do
{
//set the value of current
current = current->next;
//increment the total by 1
total++;
//end condition
} while (current != head);
// Return total nodes in list
return total;
}
//define the function createList()
void createList(struct node **head, int n)
{
//declare the required variables
int i, data;
struct node *prevNode, *newNode;
//set the nodes
prevNode = NULL;
newNode = NULL;
// Creates and links rest of the n-1 nodes
for(i=1; i<=n; i++)
{
// Create a new node
newNode = (struct node *) malloc(sizeof(struct node));
//prompt the user to enter the data
printf("Enter data of %d node: ", i);
//scan for the values
scanf("%d", &data);
//set the nodes
newNode->data = data;
newNode->next = NULL;
// Link the previous node with newly created node
if (prevNode != NULL)
prevNode->next = newNode;
// Move the previous node ahead
prevNode = newNode;
// Link head node if not linked
if (*head == NULL)
*head = newNode;
}
// Link last node with first node
prevNode->next = *head;
//print the statement
printf("\nCIRCULAR LINKED LIST CREATED SUCCESSFULLY\n");
}
// Display node content of circular linked list
void displayList(struct node *head)
{
//set the values
struct node *current;
int n = 1;
// Nothing to print in list
if(head == NULL)
{
//print the statement
printf("List is empty...

Want to see the full answer?
Check out a sample textbook solution
Chapter 3 Solutions
Data structures and algorithms in C++
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
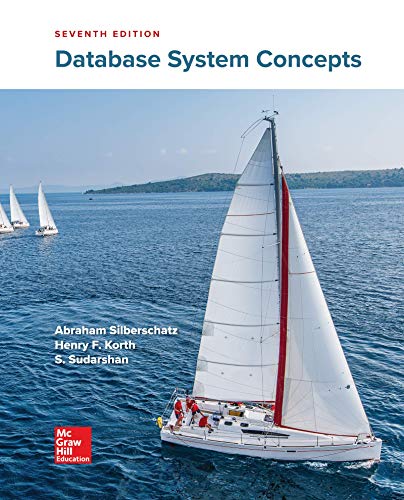
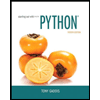
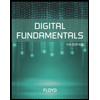
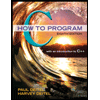
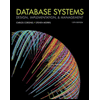
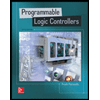