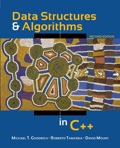
Explanation of Solution
Program code:
//include the header files
#include <iostream>
#include <cstdlib>
//use std namespce
using namespace std;
//define a class matrix
class matrix
{
//class member variables
int **p, m, n;
public:
// constructor
matrix(int row, int col)
{
//set the value of m.
m = row;
//set the value of n.
n = col;
//create the matrix
p = new int*[m];
//iterate a for loop
for (int i = 0; i < m; i++)
//set the size of matrix
p[i] = new int[n];
}
// destructor
~matrix()
{
//iterate a for loop
for (int i = 0; i < m; i++)
//delete the matrix
delete p[i];
delete p;
}
// function to take user input
void matrixInput()
{
//prompt the user to enter the elements
cout<<"Enter matrix elements:";
//iterate a for loop
for(int i = 0; i < m; i++)
{
//iterate a for loop
for(int j = 0; j < n; j++)
{
//scan for the input
cin >> p[i][j];
}
}
}
//method to display matrix
void display()
{
//print the statement
cout <<"The matrix is:";
//iterate a for loop
for(int i = 0; i < m; i++)
{
cout <<endl;
//iterate a for loop
for(int j = 0; j < n; j++)
{
//print the elements
cout << p[i][j] <<" ";
}
}
cout<<endl;
}
// overloading + operator
matrix operator +(matrix &m2)
{
//create object of matrix
matrix T(m, n);
//iterate a for loop
for(int i = 0; i < m; i++)
{
//iterate a for loop
for(int j = 0; j < n; j++)
{
//add the values of maxices
T.p[i][j] = p[i][j] + m2.p[i][j];
}
}
//return the value of T
return T;
}
// overloading * operator
matrix operator *(matrix &b)
{
//create the object of matrix
matrix T(m, b.n);
//if the condition is true
if(n == b.m)
{
//create matrix
matrix T(m, b.n);
//iterate a for loop
for(int i = 0; i < m; i++)
{
//iterate a for loop
for(int k = 0; k < b.n; k++)
{
//set the value of p[i][k]
T.p[i][k] = 0;
//iterate a for loop
for(int j = 0; j < n; j++)
{
//multiply the values and add to the array
T.p[i][k]+= p[i][j] * b.p[j][k];
}
}
}
}
//retun T
return T;
}
};
int main()
{
//call the constructor
matrix m1(2,2);
matrix m2(2,2);
//call the method matrixInput()
m1...

Want to see the full answer?
Check out a sample textbook solution
Chapter 3 Solutions
Data structures and algorithms in C++
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
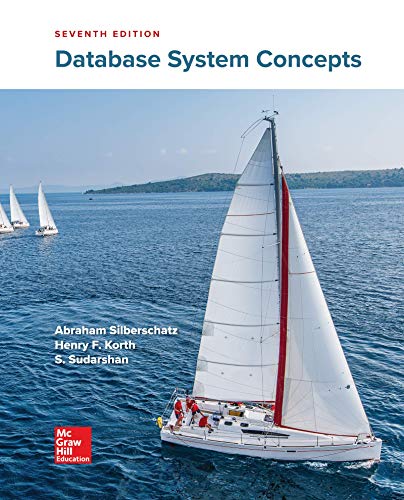
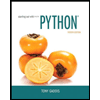
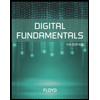
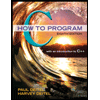
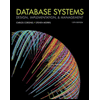
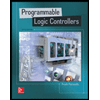