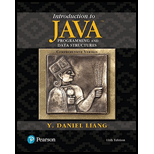
Show the output of the following code:
public class Test {
public static void main(String[] args) {
WeightedGraph<Character> graph = new WeightedGraph<>();
graph.addVertex(‘U’);
graph.addVertex(‘V’);
graph.addVertex(‘X’);
int indexForU = graph.getIndex(‘U’);
int indexForV = graph.getIndex(‘V’);
int indexForX = graph.getIndex(‘X’);
System.out.println(“indexForU is ” + indexForU);
System.out.println(“indexForV is ” + indexForV);
System.out.println(“indexForX is ” + indexForV);
graph.addEdge(indexForU, indexForV, 3.5);
graph.addEdge(indexForV, indexForU, 3.5);
graph.addEdge(indexForU, indexForX, 2.1);
graph.addEdge(indexForX, indexForU, 2.1);
graph.addEdge(indexForV, indexForX, 3.1);
graph.addEdge(indexForX, indexForV, 3.1);
WeightedGraph<Character>.ShortestPathTree tree =
graph.getShortestPath(1);
graph.printWeightedEdges();
tree.printTree();
}
}

Want to see the full answer?
Check out a sample textbook solution
Chapter 29 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Additional Engineering Textbook Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Electric Circuits. (11th Edition)
Elementary Surveying: An Introduction To Geomatics (15th Edition)
Database Concepts (8th Edition)
Concepts Of Programming Languages
Modern Database Management
- class class { public static void main(String args[]) { int a =5; int b =10; first: { second: { third: { if(a == b >>1) break second; } System.out.println(a); } System.out.println(b); } } } Give result for the code Java Try to do ASAParrow_forwardclass overload { int x; double y; void add(int a , int b) { x = a + b; } void add(double c , double d) { y = c + d; } overload() { this.x 0; %3D this.y = 0; } %3D } class Overload_methods { public static void main(String args[]) { overload obj int a = 2; double b - 3.2; obj.add(a, a); obj.add(b, b); System.out.println(obj.x + } = new overload(); + obj.y); } #3Run the codearrow_forwardpublic class Test { } public static void main(String[] args){ int a = 10; System.out.println(a*a--); }arrow_forward
- class Param3 { public int x; private void increase(int p) { x = x*p; } public void calculateX(int y) { increase(y); } public int getX() { return x; } } // in another class Param3 q3 = new Param3(); q3.x = 5; q3.calculateX(7); System.out.println(q3.getX()); what would be the answer for the last two lines ? also above were x = x*p do both x in here are the fields? wouldn't that be cnofusing?arrow_forwardclass operators { public static void main(String args[]) { int x = 8; System.out.println(++x * 3 + " " + x); } } Give result for the code Java Try to do ASAP ?arrow_forward1- public class Con{ public static void main(String[] args) int[] ar=(2,5,7,9}; int i=2; final int j=1; 6. for (int x:ar) 8- { 9 if(ar[i]arrow_forwardFind error and correct it : public class ProgramLayout { public static void main (String[] args) { final double PI = 3.14159; double x; double sum = x+PI ; System.out.println (sum); } // end classarrow_forwardExercise (5): Strings A. String Length method public class MyClass { public static void main(String[] args) { String txt = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"; System.out.println("The length of the txt string is: " + txt.length()); ) } B. Uppercase and Lowercase methods public class MyClass { public static void main(String[] args) { String txt = "Hello World"; System.out.println(txt.toUpperCase ()); // Outputs "HELLO WORLD" System.out.println(txt.toLowerCase ()); // Outputs "hello world" }} C. String concatenation The + operator can be used between strings to combine them. This is called concatenation. You can also use the concat() method to concatenate two strings public class MyClass { public static void main(String[] args) { String firstName = "Amani"; String fatherName = "Sabri"; + fatherName); System.out. println(firstName + System.out.println(firstName.concat (fatherName)); }} 1. Computer Programming (1) By: lect. Amani Abumansourarrow_forwardFor the following four classes, choose the correct relationship between each pair. public class Room ( private String m type; private double m area; // "Bedroom", "Dining room", etc. // in square feet public Room (String type, double area) m type type; m area = area; public class Person { private String m name; private int m age; public Person (String name, int age) m name = name; m age = age; public class LivingSSpace ( private String m address; private Room[] m rooms; private Person[] m occupants; private int m countRooms; private int m countoccupants; public LivingSpace (String address, int numRooms, int numoccupants) m address = address; new int [numRooms]; = new int [numOceupants]; m rooms %3D D occupants m countRooms = m countOccupants = 0; public void addRoom (String type, double area)arrow_forwardpublic class worksheet3_1 { public static void main(String[] arg) { ShadowingExample example = new ShadowingExample(); example.x = 99; example.sampleMethod(); }}class ShadowingExample { int x; public void sampleMethod() { int x = 0; System.out.println("the value of local variable x = " + ………………………………………………); System.out.println("the value of instance variable x = " + …………………………………………); }} what should be written in the second print statement so that the output is: the value of instance variable x = 99 what should be written in the first print statement so that the output is: the value of local variable x = 0 options are: samplemethod.x this.x shadowingexample.x x Answer 1 Question 1arrow_forwardpublic class GameList {Game head;public GameList() {head = null;}public void addFront(String n, int min) {Game g = new Game(n, min);g.next = head;head = g;}} Add a tail pointerarrow_forwardclass TenNums {private: int *p; public: TenNums() { p = new int[10]; for (int i = 0; i < 10; i++) p[i] = i; } void display() { for (int i = 0; i < 10; i++) cout << p[i] << " "; }};int main() { TenNums a; a.display(); TenNums b = a; b.display(); return 0;} Write a copy constructor for the above class TenNums. Make sure it performs deep copy. Group of answer choices TenNums(const TenNums& b) { p = b.p;} TenNums(const TenNums& b) { p = new int[10]; for (int i = 0; i < 10; i++) p[i] = b.p[i];} TenNums(const TenNums b) { p = new int[10]; for (int i = 0; i < 10; i++) p[i] = b.p[i];} TenNums(const TenNums b) { p = b.p;}arrow_forwardarrow_back_iosSEE MORE QUESTIONSarrow_forward_ios
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
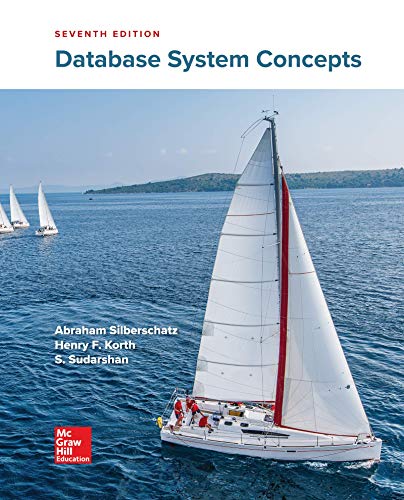
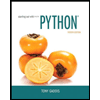
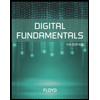
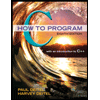
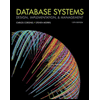
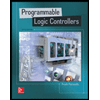