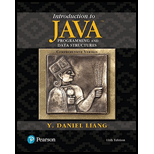
Given Code:
/* Add weighted edges in a new Array List by importing from Priority Queue class*/
List<PriorityQueue<WeightedEdge>> queues = new ArrayList<>();
/* using queue.get() method add new weighted edges with their respective weights*/
queues.get(0).offer(new WeightedEdge(0, 2, 3.5));
queues.get(0).offer(new WeightedEdge(0, 6, 6.5));
queues.get(0).offer(new WeightedEdge(0, 7, 1.5));
queues.get(1).offer(new WeightedEdge(1, 0, 3.5));
queues.get(1).offer(new WeightedEdge(1, 5, 8.5));queues.get(1).offer(new WeightedEdge(1, 8, 19.5));
/* using peek() method of queue compare the weighted edge with index 0 with index 1*/
System.out.println(queues.get(0).peek().compareTo(queues.get(1).peek()));

Want to see the full answer?
Check out a sample textbook solution
Chapter 29 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
- using namespace std; class SinglyLinkedListNode { // INSERT YOUR CODE HERE }; class SinglyLinkedList { public: SinglyLinkedListNode *head; SinglyLinkedListNode *tail; SinglyLinkedList() { this->head = nullptr; this->tail = nullptr; } voidinsert_node(intnode_data) { // INSERT YOUR CODE HERE } }; void free_singly_linked_list(SinglyLinkedListNode* node) { // INSERT YOUR CODE HERE } // Complete the has_cycle function below. /* * For your reference: * * SinglyLinkedListNode { * int data; * SinglyLinkedListNode* next; * }; * */ bool has_cycle(SinglyLinkedListNode* head) { SinglyLinkedListNode* temp = head; bool isCycle = false; while (temp != nullptr) { // INSERT YOUR CODE HERE } } int main() { // INSERT YOUR CODE HERE TO TEST YOUR CODE return0; }arrow_forwardGiven the IntNode class, define the getCount() method in the CustomLinkedList class that returns the number of items in the list not including the head node. Ex: If the list contains: head -> 14 -> 19 -> 4 getCount(headObj) returns 3. Ex: If the list contains: head -> getCount(headObj) returns 0. public class IntNode { private int dataVal; // Node data private IntNode nextNodePtr; // Reference to the next node // Default constructor public IntNode() { dataVal = 0; nextNodePtr = null; } // Constructor public IntNode(int dataInit) { this.dataVal = dataInit; this.nextNodePtr = null; } // Constructor public IntNode(int dataInit, IntNode nextLoc) { this.dataVal = dataInit; this.nextNodePtr = nextLoc; } /* Insert node after this node. Before: this -- next After: this -- node -- next */ public void insertAfter(IntNode nodeLoc) { IntNode tmpNext; tmpNext = this.nextNodePtr;…arrow_forward7. What is output by the following code section? QueueInterface aQueue = new QueueReference Based(); int numl, num2; for (int i = 1; i <= 5; i++) { aQueue.enqueue(i); } // end for for (int i = 1; i <= 5; i++) { numl = (Integer) aQueue.dequeue (); num2 (Integer) aQueue.dequeue (); aQueue.enqueue (numl + num2); aQueue.enqueue (num2 - numl); } // end for while (!aQueue.isEmpty()) { System.out.print (aQueue.dequeue () + } // end forarrow_forward
- In Java In this assignment, you should provide a complete CircularQueueDriver class that fullytests the functionality of your CircularQueue class that you have improved in Lab 8. YourCircularQueueDriver class should:• Instantiate a 3-element CircularQueue.• Use a loop to add strings to the queue until the add method returns false (whichindicates a full queue).• Call showQueue.• Use a loop to remove strings from the queue until the remove method returns null(which indicates an empty queue). As you remove each string from the queue, print theremoved string.Sample Run:Monsieur AMonsieur BMonsieur Cremoved: Monsieur Aremoved: Monsieur Bremoved: Monsieur C CircularQueue Class: public class CircularQueue { private String[] queue; // array that implements a circular queue private int length = 0; // number of filled elements private int capacity; // size of array private int front = 0; // index of first item in queue private int rear = 0; // one past the index of the last item…arrow_forwardThe below code declares a new Vector named 'vec'. What is the size of the vector at the end of the execution? Vector vec = new Vector(); for(int i=1;i<=10; i++) { vec.addElement(i); } vec.removeElement(9); vec.removeElement(10); vec.removeElement(11);arrow_forward//main.cpp #include "linkedListType.h"int main(){Node* head = NULL;orderedLinkedList oll = orderedLinkedList(head); oll.insert(40);oll.insert(100);oll.insert(50); oll.printList(); oll.insert(50);oll.printList(); return 0;} //linkedListType.h #include <stdio.h>#include <iostream>using namespace std; class Node{public:int data;Node* next;}; class orderedLinkedList {Node* head; public:orderedLinkedList(Node* h) {head = h;} void insert(int new_data){Node* head_ref = head; while (head_ref != NULL && (head_ref->next != NULL) && (head_ref->next)->data < new_data){cout << head_ref->data << "\n";head_ref = head_ref->next;} if (head_ref != NULL && (head_ref->next != NULL) && (head_ref->next)->data == new_data){cout << "ERROR: Item to be inserted is already in the list\n";return;} /* Insert new node */Node* new_node = new Node();new_node->data = new_data;if (head_ref != NULL) {new_node->next =…arrow_forward
- Using a doubly linked list class and node class, implement the following methods: Node* lastNode(void); void pushBack(string argData); void popBack(void); void pushFront(string argData); void print(void); void printReverse(void); void insertAfter(Node* argPtr); void deallocateAll(void); Node* searchFor(string argData); int size(void); Hard code a linked list (no UI) in the main to demonstrate your functions. Print forward and backward to show that the links are well-formed.arrow_forwardBooksMan import java.util.HashMap; import java.util.ArrayList; class Book{ String title, ISBN; ArrayList authors; public Book (String title, String ISBN, String authors){ this.title = title; this.ISBN = ISBN; this.authors = new ArrayList(); if (authors != null) { String [] authorArray = authors.split(", "); for (int i = 0; i < authorArray.length; i++) { this.authors.add(authorArray[i]); } } } public String getISBN() { return ISBN; } public String getTitle() { return title; } public ArrayList getAuthors() { return authors; } } public class Main { public static HashMap buildMap(Book[] s) { HashMap books = new HashMap(); if (s != null) { // TODO Write the statements here: to manage each book from the parameter array reference to the hashmap } return books; } public static void main(String argv[]) { Book[] bookArray = new Book[4]; for (int i = 0; i < 4; i++) {…arrow_forwardHow to pass this test case? Please public void testDao(){ MessageDao.reset(); MessageDao messageDao = MessageDao.getInstance(); Assert.assertEquals(messageDao, MessageDao.getInstance()); // test that constructor is private List<Constructor> constructors = Arrays.asList(MessageDao.class.getConstructors()); Assert.assertEquals(constructors.size(), 0); constructors = Arrays.asList(MessageDao.class.getDeclaredConstructors()); Assert.assertEquals(constructors.size(), 1); Assert.assertTrue(Modifier.isPrivate(constructors.get(0).getModifiers())); } We are supposed to fill this out in order to pass this test: package dao; import dto.MessageDto; import java.util.ArrayList; import java.util.HashMap; import java.util.List; import java.util.Map; // TODO fill this out public class MessageDao implements BaseDao<MessageDto> { private static MessageDao instance = new MessageDao(); public static MessageDao getInstance() { } // TODO fill this out @Override public void put(MessageDto…arrow_forward
- //give the output of the following program and draw the link list. struct node{ int info; struct node *link;};typedef struct node *nodePTR;void insort(nodePTR*,struct Info);nodePTR getnode();int main(void){nodePTR p=NULL,head=NULL,save;inti; for(i=0;i<3;i++){ insort (&head,i+5);}save=head;do{ printf("%d",save->info); save=save->link;}while(save!=NULL); return0;}nodePTR getnode(){nodePTR q;q=(nodePTR)malloc(sizeof(struct node));return q;}void insort(NODEPTR *head , int x){ NODEPTR p,q,r; q=NULL; for(p=*head; p!=NULL &&x>p->info; p=p->link) q=p; if(q=NULL) { p=getnode(); p->info=x; p->link=head; head=p; }else{ r=getnode(); q->link=r; r->info=x; r->link=p; }}arrow_forwardIn an array based FIFO queue Q, which of the following is correct about the queue after Q.Dequeue( ) is executed? The size of the array is 9 as shown below: 5 (front) 9 8 7 3(rear) 4 front = 1; rear =8 front = 0; rear =7; front = 2; rear =3; front = 0; rear =8;arrow_forwardpublic class ShoppingListDriver{ public static void main(String[] args){ ShoppingList sl=new ShoppingList(3);sl.insert(null);sl.insert(new Item("Bread", "Carb Food", 2, 2.99));sl.insert(new Item("Seafood","Sea Food", -1, 10.99));sl.insert(new Item("Rice", "Carb Food",2, 19.99));sl.insert(new Item("Salad Dressings","Dessing", 2, 19.99));sl.insert(new Item("Eggs", "Protein",2, 3.99));sl.insert(new Item("Cheese","Protein", 2, 1.59));sl.insert(new Item("Eggs", "Protein",3, 3.99));sl.printNames();sl.print(); System.out.println("After removing Eggs:");sl.remove(new Item("Eggs","Protein",0,0));sl.printNames();sl.print(); }}arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
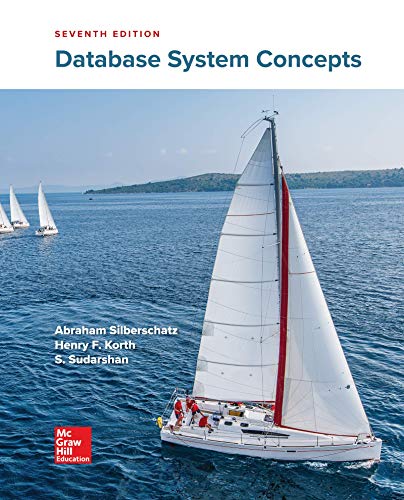
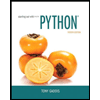
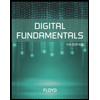
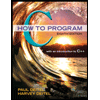
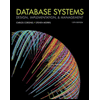
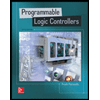