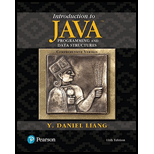
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 29, Problem 29.12PE
Program Plan Intro
Display weighted graphs
Program Plan:
- Create a package “main”.
- Add a java class named “Edge” to the package which is used to get the edges from the graph.
- Add a java class named “Graph” to the package which is used to add and remove vertices, edges.
- Add a java class named “UnweightedGraph” to the package which is used to store vertices and neighbors.
- Add a java class named “WeightedGraph” to the package which is used to get the weighted edges and print the edges.
- Add a java class named “WeightedEdge” to the package which is used to compare edges.
- Add a java class name “Displayable” to the package.
- Declare the functions “get_X ()”, “get_Y ()”, “get_Name ()”.
- Add a java class named “E12” to the package.
- Declare the cities.
- Declare an integer array.
- Create an object for the weighted graph.
- Create a graphview pane.
- Declare “start ()” method that overrides the “start ()” method in the “Application” class.
- Create a scene and place it on the stage.
- Display the stage.
- Declare the class “GraphView”.
- The “Graph” class extends the interface “Displayable”.
- Give the constructor for this class.
- Set the graph and call the function “paint ()”.
- Give function definition for “paint ()”.
- Declare a list that extends Displayable.
- Loop from 0 through size.
- Get the vertices.
- Get the name of the city.
- Add the circle, text and vertices to the pane.
- Loop from 0 through graph size.
- Create a list.
- Loop from 0 through neighbor’s size.
- Get the vertices.
- Inside the “try” block,
- Add the line, text and vertices to the pane.
- Inside the “catch” block,
- Throw the exception.
- Declare a class named “Cities”.
- Declare required variables.
- Give the constructor for this class which sets the name of the city, and the vertices.
- Get and return the co-ordinate of the vertex “x”.
- Get and return the co-ordinate of the vertex “y”.
- Get and return the name of the city.
- Declare function to compare cities.
- Return the value.
- Give the “main ()” method.
- Launch the application.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Union-Find: Maze
Write a program that generates mazes of arbitrary size using the union-find algorithm. A
simple algorithm to generate the maze is to start by creating an N x M grid of cells separated by
walls on all sides, except for entrance and exit. Then continually choose a wall randomly, and
knock it down if the cells are not already connected to each other. If we repeat the process until
the starting and ending cells are connected, we have a maze. It is better to continue knocking
down the walls until every cell is reachable from every cell as this would generate more false
leads in the maze.
Test you algorithm by creating a 15 x 15 grid, and print all the walls that have been knocked
down. D
2). Write a program to implement the following graph representations and display
them.
i. Adjacency list
ii. Adjacency matrix
C++
A program applying the topics involved in data structures (linked list, graph, array etc.)
1. User will be asked to login/signup
2. User will choose for the nearest restaurant (undirected graph?)
3. User will add to cart (from the menu list of the restaurant)
4. User will be asked for order confirmation and mode of payment (cash on delivery, card??) note: new users will have a discount
5. Invoice
Using Dev c++
Chapter 29 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 29.2 - Prob. 29.2.1CPCh. 29.2 - Prob. 29.2.2CPCh. 29.3 - Prob. 29.3.1CPCh. 29.3 - Prob. 29.3.2CPCh. 29.3 - Show the output of the following code: public...Ch. 29.4 - Prob. 29.4.1CPCh. 29.4 - Prob. 29.4.2CPCh. 29.4 - Prob. 29.4.3CPCh. 29.4 - Prob. 29.4.4CPCh. 29.4 - Show the output of the following code: public...
Ch. 29.5 - Prob. 29.5.2CPCh. 29.5 - Prob. 29.5.3CPCh. 29.5 - Prob. 29.5.4CPCh. 29.5 - Prob. 29.5.5CPCh. 29.5 - Prob. 29.5.6CPCh. 29.5 - Show the output of the following code: public...Ch. 29.6 - Prob. 29.6.1CPCh. 29.6 - Prob. 29.6.2CPCh. 29.6 - Prob. 29.6.3CPCh. 29 - (Modify weight in the nine tails problem) In the...Ch. 29 - (Find a minimum spanning tree) Write a program...Ch. 29 - (Create a file for a graph) Modify Listing 29.3,...Ch. 29 - Prob. 29.11PECh. 29 - Prob. 29.12PE
Knowledge Booster
Similar questions
- Programming Language: C++ Create a pseudocode (if you not familiar with this, you can create a program) that check if "directed graph" is actually circle graph. The meaning of circle is that let's say there is 1~6 nodes. The graph start with 1 and only can move to 2, and 2 can only move to 3....etc and after you hit 6, you move back to 1. so 1=>2=>3=>4=>5=>6=>1 this is example of circle directd graph. Please create a pseudocode/program to check if graph is circle or not. Also, include the time complexity of your pseudocode by [Worst case time complexity / Best case time complexity]arrow_forwardArtificial intelligence (Question - 6) ======================= One variation on the game of nim is described in Luger. The game begins with a single pile of stones. The move by a player consists of dividing a pile into two piles that contain an unequal number of stones. For example, if one pile contains six stones, it could be subdivided into piles of five and one, or four and two, but not three and three. The first player who cannot make a move loses the game.(6.1) Draw the complete game tree for this version of Nim if the start state consists of six stones.(6.2) Perform a minimax evaluation for this game. Let 1 denote a win and 0 a loss.arrow_forwardData structure/ C language / Graph / Dijkstra’s algorithm implement a solution of a very common issue: howto get from one town to another using the shortest route.* design a solution that will let you find the shortest paths betweentwo input points in a graph, representing cities and towns, using Dijkstra’salgorithm. Your program should allow the user to enter the input filecontaining information of roads connecting cities/towns. The programshould then construct a graph based on the information provided from thefile. The user should then be able to enter pairs of cities/towns and thealgorithm should compute the shortest path between the two cities/townsentered.Attached a file containing a list of cities/towns with the following data:Field 1: Vertex ID of the 1st end of the segmentField 2: Vertex ID of the 2nd of the segmentField 3: Name of the townField 4: Distance in KilometerPlease note that all roads are two-ways. Meaning, a record may representboth the roads from feild1 to field2…arrow_forward
- C++ A robot is initially located at position (0; 0) in a grid [?5; 5] [?5; 5]. The robot can move randomly in any of the directions: up, down, left, right. The robot can only move one step at a time. For each move, print the direction of the move and the current position of the robot. If the robot makes a circle, which means it moves back to the original place, print "Back to the origin!" to the console and stop the program. If it reaches the boundary of the grid, print \Hit the boundary!" to the console and stop the program. A successful run of your code may look like:Down (0,-1)Down (0,-2)Up (0,-1)Left (-1,-1)Left (-2,-1)Up (-2,0)Left (-3,0)Left (-4,0)Left (-5,0)Hit the boundary! or Left (-1,0)Down (-1,-1)Right (0,-1)Up (0,0)Back to the origin! About: This program is to give you practice using the control ow, the random number generator, and output formatting. You may use <iomanip> to format your output. You may NOT use #include "stdafx.h".arrow_forwardBar Graph, v 1.0 Purpose. The purpose of this lab is to produce a bar graph showing the population growth of a city called Prairieville, California. The bar graph should show the population growth of the city in 20 year increments for the last 100 years. The bar graph should be printed using a loop. The loop can be either a while loop or a for loop. Write a program called cityPopulation Graph.cpp to produce the bar graph. Requirements. 1. Use these population values with their corresponding years: 1922, 2000 people 1942, 5000 people 1962, 6000 people 1982, 9000 people 2002, 14,000 people 2012, 15,000 people 2022, 17,000 people 2. For each year, the program should display the year with a bar consisting of one asterisk for each 1000 people. 3. Each time the loop is performed (that is, each time the loop iterates) one of the year values is displayed with asterisks next to it. That is: 1st time loop is performed/iterates the year 1922 and the correct number of asterisks should be…arrow_forwardVolume of Histogram: Imagine a histogram (bar graph). Design an algorithm to compute the volume of water it could hold if someone poured water across the top. You can assume that each histogram bar has width 1. EXAMPLE Input: {0, 0, 4, 0, 0, 6, 0, 0, 3, 0, 5, 0, 1, 0, 0, 0} (Black bars are the histogram. Gray is water.) Output: 26 0 0 4 0 0 6 0 0 3 0 5 0 1 0 0 0arrow_forward
- Indicate whether the following statements are True or False: Namespaces allow for naming variables, methods, classes without worrying about conflict. T/ F а. b. cin allows us to read text from a file. T / F A simple graph is a graph with nodes having only one incident edge. т/F с. d. In order to perform a binary search on an array, the array has to be sorted. T / F In general, bubble sort is faster than insertion sort. T / F е. A header file of a class describes what member functions do without telling the f. T/ F implementation. g. Enqueue and dequeue operations manipulate an element at the same end of a queue. T/ F h. In doubly linked list, the next pointer of the last data element always points to head. T / F i. In a dictionary, a key can be paired with only one value. т/ F j. In inheritance, functions of the child class can access all members of its parent class. T / F k. In inheritance, functions of the parent class can access all members of its child class. T / F I. Iterators are…arrow_forward#this is a python programtopic: operation overloading, Encapsulation please find the attached imagearrow_forwardOne variation on the game of nim is described in Luger. The game begins with a single pile of stones. The move by a player consists of dividing a pile into two piles that contain an unequal number of stones. For example, if one pile contains six stones, it could be subdivided into piles of five and one, or four and two, but not three and three. The first player who cannot make a move loses the game. (5.1) Draw the complete game tree for this version of Nim if the start state consists of six stones. (5.2) Perform a minimax evaluation for this game. Let 1 denote a win and 0 a loss.arrow_forward
- Automatic Indexes: Starting with the program from 16-2 which processes Death Valley data, modify the program such that it sets the temperature ranges based on the data contained in the file. Allow for the graph to display 10 degrees above the highest max and 10 degrees below the lowest minimum temperature. Use the station name to automatically generate an appropriate title for your graph as well.arrow_forwardKnight's Tour: The Knight's Tour is a mathematical problem involving a knight on a chessboard. The knight is placed on the empty board and, moving according to the rules of chess, must visit each square exactly once. There are several billion solutions to the problem, of which about 122,000,000 have the knight finishing on the same square on which it begins. When this occurs the tour is said to be closed. Your assignment is to write a program that gives a solution to the Knight's Tour problem recursively. You must hand in a solution in C++ AND Java. The name of the C++ file should be "main.cc" and the name of the Java file should be "Main.java". Write C++ only with a file name of main.cc Please run in IDE and check to ensure that there are no errors occuring Output should look similar to: 1 34 3 18 49 32 13 16 4 19 56 33 14 17 50 31 57 2 35 48 55 52 15 12 20 5 60 53 36 47 30 51 41 58 37 46 61 54 11 26 6 21 42 59 38 27 64 29 43 40 23 8 45 62 25 10 22 7 44 39 24 9 28 63arrow_forward(YOU ARE NOT ALLOWED TO USE ARRAYLIST IN THIS PROJECT)Write a Java program to simulate a blackjack game of cards. The computer will play the role of the dealer. The program will randomly generate the cards dealt to the player and dealer during the game. Cards in this game will be represented by numbers 1 to 13 with Ace being represented by a 1. Remember, that face cards (i.e. Jack, Queen, and King) are worth 10 points to a hand while an Ace can be worth 1 or 11 points depending on the user’s choice. The numbered cards are worth their number value to the hand.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
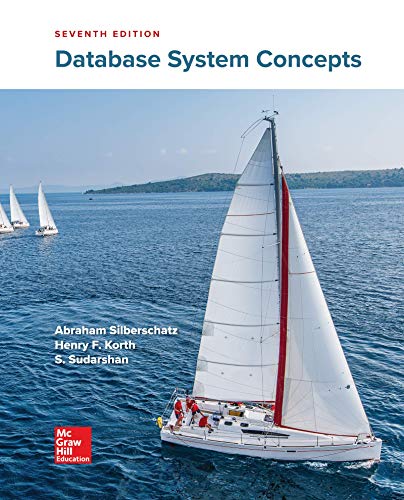
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
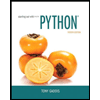
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
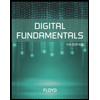
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
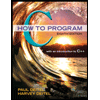
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
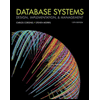
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
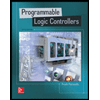
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education