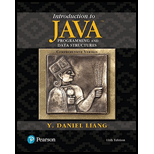
(Modify weight in the nine tails problem) In the text, we assign the number of the flips as the weight for each move. Assuming the weight is three times of the number of flips, revise the

Program Plan:
- Create a package “main”.
- Add a java class named “Edge” to the package which is used to get the edges from the graph.
- Add a java class named “Graph” to the package which is used to add and remove vertices, edges.
- Add a java class named “UnweightedGraph” to the package which is used to store vertices and neighbors.
- Add a java class named “WeightedGraph” to the package which is used to get the weighted edges and print the edges.
- Add a java class named “WeightedEdge” to the package which is used to compare edges.
- Add a java class named “NineTailModel” to the package which is used to compare edges.
- Add a java class named “Test” to the package.
- Import the required packages.
- Declare the main class.
- Give the “main ()” method.
- Allocate the memory for the “Test” class.
- Define “Test”.
- Get the initial nine coins from the user.
- Create an object for the “ModifiedWeightedNineTailModel” class.
- Create an array list.
- Display the steps to flip the coin.
- Display the number of flips.
- Define “ModifiedWeightedNineTailModel” class.
- Create an edges and graph.
- Obtain a BSF tree rooted at the target node.
- Define “getEdges” method.
- Create an array list.
- Create all the edges for the graph by calling “getFlippedNode” and “getNumberOfFlips” methods.
- Add edge for a legal move from the node u to v.
- Return the edge.
- Define “getNumberOfFlips” method.
- Declare the required variables.
- Check if the “node1” is not equal to “node2” means increment the “count”.
- Return the value.
- Define “getNumberOfFlips” method.
- Return the total number of flips.
- Give the “main ()” method.
The given program is used to modify weight in the nine tails problem is as follows:
Explanation of Solution
Program:
Edge.java: Refer book from chapter 28 – Listing 28.1.
Graph.java: Refer book from chapter 28 – Listing 28.3.
UnweightedGraph.java: Refer book from chapter 28 – Listing 28.4.
WeightedGraph.java: Refer book from chapter 29 – Listing 29.2.
WeightedEdge.java: Refer book from chapter 29 – Listing 29.1.
NineTailModel.java: Refer book from chapter – Listing 28.13.
Test.java:
//import the required statement
import java.util.ArrayList;
import java.util.List;
import java.util.Scanner;
//definition of "Test" class
public class Test
{
//main method
public static void main(String[] args)
{
//memory allocation
new Test();
}
//definition of "Test"
public Test()
{
// get the input from the user
System.out.print("Enter an initial nine coin H’s and T's: ");
Scanner input = new Scanner(System.in);
String s = input.nextLine();
//declare the variable
char[] initialNode = s.toCharArray();
//create an object
ModifiedWeightedNineTailModel model = new ModifiedWeightedNineTailModel();
//create an ArrayList
java.util.List<Integer> path =
model.getShortestPath (NineTailModel.getIndex(initialNode));
//display the steps to flip the coin
System.out.println("The steps to flip the coins are ");
for (int i = 0; i < path.size(); i++)
NineTailModel.printNode(
NineTailModel.getNode (path.get(i).intValue()));
//display the number of flips
System.out.println("The number of flips is " +
model.getNumberOfFlips (NineTailModel.getIndex(initialNode)));
}
//definition of "ModifiedWeightedNineTailModel"
public static class ModifiedWeightedNineTailModel extends NineTailModel
{
//constructor
public ModifiedWeightedNineTailModel()
{
// create edges
List<WeightedEdge> edges = getEdges();
// create a graph
WeightedGraph<Integer> graph = new WeightedGraph<Integer>(
edges, NUMBER_OF_NODES);
/* obtain a BSF tree rooted at the target node*/
tree = graph.getShortestPath(511);
}
//definition of "getEdge" method
private List<WeightedEdge> getEdges()
{
// create an ArrayList
List<WeightedEdge> edges = new ArrayList<WeightedEdge>();
//check the condition
for (int u = 0; u < NUMBER_OF_NODES; u++)
{
//check the condition
for (int k = 0; k < 9; k++)
{
// get the node for vertex u
char[] node = getNode(u);
//check the condition
if (node[k] == 'H')
{
/*call the "getFlippedNode" method*/
int v = getFlippedNode(node, k);
int numberOfFlips = getNumberOfFlips(u, v);
/* add edge for a legal move from node u to node v*/
edges.add(new WeightedEdge(v, u, numberOfFlips));
}
}
}
//return statement
return edges;
}
//definition of "getNumberOfFlips" method
private static int getNumberOfFlips(int u, int v)
{
//declare the variables
char[] node1 = getNode(u);
char[] node2 = getNode(v);
int count = 0;
//check the condition
for (int i = 0; i < node1.length; i++)
//check the condition
if (node1[i] != node2[i]) count++;
//return statement
return 3 * count;
}
//definition of "getNumberOfFlips" method
public int getNumberOfFlips(int u)
{
//return statement
return (int)((WeightedGraph<Integer>.ShortestPathTree)tree).getCost(u);
}
}
}
Enter an initial nine coin H’s and T's: HHHTTTHHH
The steps to flip the coins are
HHH
TTT
HHH
HHH
THT
TTT
TTT
TTT
TTT
The number of flips is 24
Want to see more full solutions like this?
Chapter 29 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Additional Engineering Textbook Solutions
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Mechanics of Materials (10th Edition)
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Modern Database Management
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
- (Intro to Python) Write a program that adds all even numbers between 1 and 101 and outputs the sum. Define and call at least one function. If you solved this using a “for” loop, redo this using a “while” loop and vice versa.arrow_forward(Computer mini-project) Area of a unit circle equals rt. Cover a circle with a 2 by 2 square and follow Algorithm 5.5 to estimate number Tt based on 100, 1,000, and 10,000 random numbers. Compare results with the exact value t = 3.14159265358... and comment on precision. %3D Please build an algorithm, test it and explain the results. Please include code. 351 /5,000arrow_forward((Simulation: The Tortoise and the Hare) In this problem, you’ll re-create one of the truly great moments in history, namely the classic race of the tortoise and the hare. You’ll use random number generation to develop a simulation of this memorable event. Our contenders begin the race at square 1 of 70 squares. Each square represents a possible position along the race course. The finish line is at square 70. The first contender to reach or pass square 70 is rewarded with a pail of fresh carrots and lettuce. The course weaves its way up the side of a slippery mountain, so occasionally the contenders lose ground. Use variables to keep track of the positions of the animals (i.e., position numbers are 1–70). Start each animal at position 1 (i.e., the “starting gate”). If an animal slips left before square 1, move the animal back to square 1. Generate the percentages in Fig. 10.24 by producing a random integer i in the range 1 ≤i ≤10. For the tortoise, perform a “fast plod” when 1 ≤i…arrow_forward
- (Use Python) Use the Design Recipe to write a function, print_histogram that consumes a list of numbers and prints a histogram graph using asterisks to represent each number in the list. Use one output line per number in the list. You may assume that only integers are passed to this function. Your function should ignore negative values. Test Result print_histogram([ 2, 0, 4, 1]) ** ***** print_histogram([10, 5, 3, -1, 8]) **************************arrow_forward(Factorials) The factorial function is used frequently in probability problems. The factorialof a positive integer n (written n! and pronounced “n factorial”) is equal to the product of the positive integers from 1 to n. Write a program that evaluates the factorials of the integers from 1 to 5.Print the results in tabular format. What difficulty might prevent you from calculating the factorialof 20?arrow_forward(USING MATLAB!!!) Write a function “PlotXY” that will plot data points represented by the x and y vectors which are passed as the first two input arguments. In other words, this function takes at least two input arguments. In case a third argument is passed, it is a color. Add a title to the plot to display the total number of arguments passed to the function. hints: check Matlab help on plot() regarding changing the plot properties (color, linewidth). Here is an example of calling the function and the resulting plot: >> x=-pi:pi/50:2*pi; >> y = sin(x);>> PlotXY(x,y,’r’)arrow_forward
- (Introduction to Python) Include your name and program output in a comment at the top of the script you submit. Note: upload a script for example scriptname.py (do not copy-paste the interactive mode commands) Write complete code that asks the user to input two integer numbers. Define and call a function called remainder that takes two parameters x and y and prints the remainder when x is divided by y. When y is zero, it should not perform the division and instead print "cannot divide by zero" to prevent the runtime error. Sample output is as follows: Enter a number: x Enter another number: y Remainder of x divided by y is z (you'll fill in the values of x, y and z) Note: upload a script for example labD.py or scriptname.py (do not copy-paste the interactive mode commands)arrow_forward(Geometry: area of a regular polygon) A regular polygon is an n-sided polygon in which all sides are of the same length and all angles have the same degree (i.e., the polygon is both equilateral and equiangular). The formula for computing the area of a regular polygon is n x s? Area 4 X tan Here, s is the length of a side. Write a program that prompts the user to enter the number of sides and their length of a regular polygon and displays its area. Here is a sample run:arrow_forward(Don't copy) Please Help-- - java processing Create a T.V static program create a setup in draw and in the draw you have to create a nested for loop that puts a random pixel at each point.arrow_forward
- (Integer) Bit Operators. Write a program that takes begin and end values and prints out a decimal, binary, octal, hexadecimal chart like below. If any of the characters are printable ASCII characters, then print those, too. If none is, you may omit the ASCII column header.arrow_forward(USE 1 DECIMAL FOR CALCULATION) A researcher believes that design and the safety of the cars is very important for the customers when buying a car. In order to understand the brand perception of customers' researcher shows three different car pictures and ask them can you classify this car in picture) as "sporty" or "safety? the following cross tabulation is obtained. Test the claim that car brand and consumer perception is dependent Automobiles Sporty Safety BMW 60 130 Mercedes 90 120 Renault 50 50 Calculate the test statistics .arrow_forward(Rounding Numbers) Function floor can be used to round a number to a specific decimal place. The statementy = floor(x * 10 + 0.5) / 10;rounds x to the tenths position (the first position to the right of the decimal point). The statementy = floor(x * 100 + 0.5) / 100;rounds x to the hundredths position (the second position to the right of the decimal point). Write a program that defines fourfunctions to round a number x in various ways:A. roundToInteger(number)B. roundToTenths(number)C. roundToHundredths(number)D. roundToThousandths(number)For each value read, your program should print the original value, the number rounded to the nearest integer, the number rounded to the nearest tenth, the number rounded to the nearest hundredth and the number rounded to the nearest thousandth.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
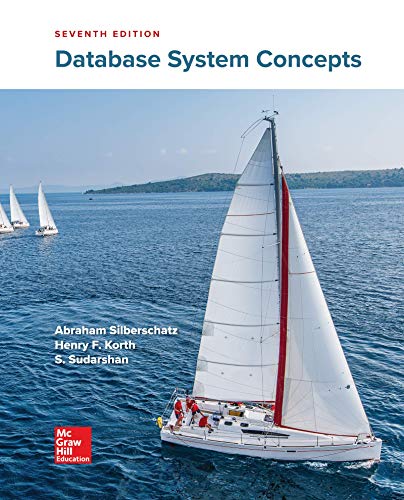
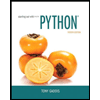
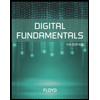
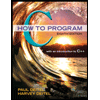
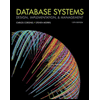
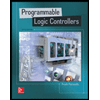