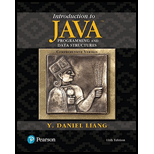
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 25, Problem 25.9PE
Program Plan Intro
Program Plan:
- Include the required import statement.
- Define the main class.
- Define the main method using public static main.
- Allocate memory to the class “Test”.
- Define the “Test” class.
- Declare the object for the BST.
- Insert the values into the tree.
- Call the “postorder” method.
- The try-catch block is used to get the input from the user and do the postorder traversal.
- Display the output.
- Define the “BST” class.
- Declare the required variables.
- Create a default BST class.
- Define the “clone” method.
- Create an object for the “BST” class.
- Call the “copy” method with the argument.
- Return the tree.
- Define the “copy” method.
- If the “s” is not null, insert the element into the tree.
- Copy the “t” value into left and right subtrees.
- If the “s” is not null, insert the element into the tree.
- Define the “equals” method.
- Create the object for the array list.
- Call the “toArray” method.
- Return the two array.
- Define the ArrayList of “getElementInorder” method
- Create the object for the array list.
- Call the “getElementInorder” method.
- Return the list.
- Define the “getElementInorder” method.
- If the “root” is equal to null, return the value.
- Call the “getElementInorder” method.
- Add the element into the list.
- Call the “getElementInorder” method.
- Create a binary tree from an array of objects.
- Define the “search” method.
- Start the traverse from the root of the tree.
- If the search element is in the left subtree set that value in “current” variable otherwise set the “current” variable as right subtree value.
- Define the “insert” method.
- If the root is null create the tree otherwise insert the value into left or right subtree.
- Define the “createNewNode”
- Return the result of new node creations.
- Define the “inorder”
- Inorder traverse from the root.
- Define the protected “inorder” method
- Traverse the tree according to the inorder traversal concept.
- Define the “postorder”
- Postorder traverse from the root.
- Define the protected “postorder” method
- Traverse the tree according to the postorder traversal concept.
- Define the “preorder”
- Preorder traverse from the root.
- Define the protected “preorder” method
- Traverse the tree according to the preorder traversal concept.
- Define the “TreeNode” class
- Declare the required variables.
- Define the constructor.
- Define the “getSize” method.
- Return the size.
- Define the “getRoot” method
- Return the root.
- Define the “java.util.ArrayList” method.
- Create an object for the array list.
- If the “current” is not equal to null, add the value to the list.
- If the “current” is less than 0, set the “current” as left subtree element otherwise set the “current” as right subtree element.
- Return the list.
- Define the “delete” method.
- If the “current” is not equal to null, add the value to the list.
- If the “current” is less than 0, delete the “current” as left subtree element otherwise delete the “current” as right subtree element.
- Return the list.
- Define the “iterator” method.
- Call the “inorderIterator” and return the value.
- Define the “inorderIterator”
- Create an object for that method and return the value
- Define the “inorderIterator” class.
- Declare the variables.
- Define the constructor.
- Call the “inorder” method.
- Define the “inorder” method.
- Call the inner “inorder” method with the argument.
- Define the TreeNode “inorder” method.
- If the root value is null return the value, otherwise add the value into the list.
- Define the “hasNext” method
- If the “current” value is less than size of the list return true otherwise return false.
- Define the “next” method
- Return the list.
- Define the “remove” method.
- Call the delete method.
- Clear the list then call the “inorder” method.
- Define the “clear” method
- Set the values to the variables
- Define the interface.
- Declare the required methods.
- Define the required methods.
- Define the main method using public static main.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
(Test perfect binary tree)
A perfect binary tree is a complete binary tree with all levels fully filled. Define a
new class named BSTWithTestPerfect that extends BST with the
following methods: (Hint: The number of nodes in a perfect binary tree is
2^(height+1) - 1.)
/** Returns true if the tree is a perfect binary tree
*/
public boolean isPerfectBST()
Use https://liveexample.pearsoncmg.com/test/Exercise25_03.txt to test your
code.
Class Name: Exercise25 03
(Test perfect binary tree) JAVA
A perfect binary tree is a complete binary tree with all levels fully filled. Define a new class named BSTWithTestPerfect that extends BST with the following methods: (Hint: The number of nodes in a perfect binary tree is 2^(height+1) - 1.)
/** Returns true if the tree is a perfect binary tree */public boolean isPerfectBST()
Use https://liveexample.pearsoncmg.com/test/Exercise25_03.txt to test your code.
Class Name: Exercise25_03
( as) Write a Java method named displayTree. The main method will pass it the Tree
created above and the way to display the Tree as a string, such as “postOrder" and "inOrder".
Then displayTree will display the Tree as instructed by the main method;
Chapter 25 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 25.2 - Prob. 25.2.1CPCh. 25.2 - Prob. 25.2.2CPCh. 25.2 - Prob. 25.2.3CPCh. 25.2 - Prob. 25.2.4CPCh. 25.2 - Prob. 25.2.5CPCh. 25.3 - Prob. 25.3.1CPCh. 25.3 - Prob. 25.3.2CPCh. 25.3 - Prob. 25.3.3CPCh. 25.3 - Prob. 25.3.4CPCh. 25.4 - Prob. 25.4.1CP
Ch. 25.4 - Prob. 25.4.2CPCh. 25.4 - Prob. 25.4.3CPCh. 25.4 - Prob. 25.4.4CPCh. 25.4 - Prob. 25.4.5CPCh. 25.5 - Prob. 25.5.1CPCh. 25.5 - Prob. 25.5.2CPCh. 25.5 - Prob. 25.5.3CPCh. 25.5 - Prob. 25.5.4CPCh. 25.5 - Prob. 25.5.5CPCh. 25.6 - Prob. 25.6.1CPCh. 25.6 - Prob. 25.6.2CPCh. 25.6 - Prob. 25.6.3CPCh. 25.6 - How do you replace lines 9499 in Listing 25.11...Ch. 25 - Prob. 25.1PECh. 25 - (Implement inorder traversal without using...Ch. 25 - (Implement preorder traversal without using...Ch. 25 - (Implement postorder traversal without using...Ch. 25 - Prob. 25.6PECh. 25 - Prob. 25.7PECh. 25 - (Implement bidirectional iterator) The...Ch. 25 - Prob. 25.9PECh. 25 - Prob. 25.10PECh. 25 - Prob. 25.11PECh. 25 - (Test BST) Design and write a complete test...Ch. 25 - (Modify BST using Comparator) Revise BST in...Ch. 25 - Prob. 25.15PECh. 25 - (Data compression: Huffman coding) Write a program...Ch. 25 - Prob. 25.17PECh. 25 - (Compress a file) Write a program that compresses...Ch. 25 - (Decompress a file) The preceding exercise...
Knowledge Booster
Similar questions
- (Java) How can I implement a binary search tree in a program to store data, and use the delete method to trim the tree? The program should have a 'populate' button that obtains a string from the user, creates a sorted binary search tree of characters, and prints the tree using the DisplayTree class. It should also print the characters on one line in sorted order by traversing the tree in inorder fashion. The program should also have a 'Trim Tree' button that obtains a second line of input to delete characters from the tree, trimming the tree accordingly. It should ignore characters that are not in the tree, and only delete one character for each occurrence in the second line of input. When all characters from the second line have been deleted from the tree, the program should print the remaining characters in the tree using the DisplayTree class. The output should be labeled appropriately, and no spaces or commas should be used between tree elements in the inorder traversal.Here is the…arrow_forwardTopic: Singly Linked ListImplement the following functions in C++ program. Read the question carefully. (See attached photo for reference) void isEmpty() This method will return true if the linked list is empty, otherwise return false. void clear() This method will empty your linked list. Effectively, this should and already has been called in your destructor (i.e., the ~LinkedList() method) so that it will deallocate the nodes created first before deallocating the linked list itself.arrow_forwardJAVA CODE Learning Objectives: Detailed understanding of the linked list and its implementation. Practice with inorder sorting. Practice with use of Java exceptions. Practice use of generics. You have been provided with java code for SomeList<T> class. This code is for a general linked list implementation where the elements are not ordered. For this assignment you will modify the code provided to create a SortedList<T> class that will maintain elements in a linked list in ascending order and allow the removal of objects from both the front and back. You will be required to add methods for inserting an object in order (InsertInorder) and removing an object from the front or back. You will write a test program, ListTest, that inserts 25 random integers, between 0 and 100, into the linked list resulting in an in-order list. Your code to remove an object must include the exception NoSuchElementException. Demonstrate your code by displaying the ordered linked list and…arrow_forward
- Computer Science JAVA Write a program that maintains the names of your friends and relatives and thus serves as a friends list. You should be able to enter, delete, modify, or search this data. You should assume that the names are unique. use a class to represent the names in the friends list and another class to represent the friends list itself. This class should contain a Binary Search Tree of names as a data field. (TreeNode Class BinarySearchTree Class FriendsList Class)arrow_forward- the constructor needs to initialize tailPtr to nullptr - insert(): modify it to update prev pointers as well as next pointers. - remove(): modify to update prev pointers as well as next pointers. Add a new public member function to the LinkedList class named reverse() which reverses the items in the list. swap each node’s prev/next pointers, and finally swap headPtr/tailPtr. Demonstrate your function works by creating a sample list of a few entries in main(), printing out the contents of the list, reversing the list, and then printing out the contents of the list again to show that the list has been reversed. Note: your function must actually reverse the items in the doubly-linked list, not just print them out in reverse order! we won't use the copy constructor in this assignment, and as such you aren't required to update the copy constructor to work with a doubly-linked list. @file LinkedList.cpp */ #include "LinkedList.h" // Header file#include <cassert>#include…arrow_forward(Please Help. My professor did not teach us any of this and will not answer my emails) Design a class named Queue for storing integers. Like a stack, a queue holds elements. In a stack, the elements are retreived in a last-in-first-out fashion. In a queue, the elements are retrieved in a first-in-first-out fashion. The class contains: An int[] data field named elements that stores the int values in the queue A data field named size that stores the number of elements in the queue A constructor that creates a Queue object with defult capacity 8 The method enqueue(int v) that adds v into the queue The method empty () that returns true if the queue is empty The method getSize() that returns the size of the queuearrow_forward
- (Implement a doubly linked list) The MyLinkedList class used in Listing 24.6 is a one-way directional linked list that enables one-way traversal of the list. Modify the Node class to add the new data field name previous to refer to the previous node in the list, as follows:public class Node<E> { E element; Node<E> next; Node<E> previous;public Node(E e) { element = e; } }Implement a new class named TwoWayLinkedList that uses a doubly linked list to store elements. The MyLinkedList class in the text extends MyAbstractList. Define TwoWayLinkedList to extend the java.util.AbstractSequentialList class. You need to implement all the methods defined in MyLinkedList as well as the methods listIterator() and listIterator(int index). Both return an instance of java.util. ListIterator<E>. The former sets the cursor to the head of the list and the latter to the element at the specified index.arrow_forwardTopic: Singly Linked ListImplement the following functions in C++ program. Read the question carefully. (See attached photo for reference) int count(int num) This will return the count of the instances of the element num in the list. In the linked list in removeAll method, having the method count(10) will return 3 as there are three 10's in the linked list. Additionally, you are to implement these methods: bool move(int pos1, int pos2) This method will move the element at position pos1 to the position specified as pos2 and will return true if the move is successful. This will return false if either the specified positions is invalid. In the example stated, operating the method move(1, 3) will move the 1st element and shall now become the 3rd element. The linked list shall now look like: 30 -> 40 -> 10 -> 50 int removeTail() This method will remove the last element in your linked list and return the said element. If the list is empty, return 0. You can use the…arrow_forwardUse java or python to solve Note: 1) Assume that the Node class and Linked Lists constructors are already written. You ONLY need to write the revinsert0 method/function 2) No need to write the Tester Class. 3) Use of Built in Method is not allowed except count(). You must take an inplace approach and modify the given list. 5) You cannot use arrays to solve this problemarrow_forward
- How nodes are defined (struct node (value count left right) #:mutable #:transparent) Write in Racket (traverse n) A traversal of a BST is an algorithm for “visiting” all node in the BST. The traversal must visit each node exactly once. In the case of a linked list, a traversal is trivial since the structure is linear: start at the head, move to the next node, and stop when you reach the tail. In the case of a BST, traversal must account for multiple child nodes and keep track of which subtrees have already been visited and which have not. There are three types of traversal: in-order, pre-order, and post-order. We will only implement in-order. The in-order traversal of a BST has the property that the node values will display in ascending or sorted order. The function can be defined either recursively or iteratively. Recursion is much simpler, so we’ll stick to that. Recursive Algorithm for In-Order Traversal of BST parameter: node n, the root of the tree…arrow_forward(Python)- Implement functions successor and predecessor. These functions will take two arguments: the root of a tree and an integer. The successor function returns the smallest item in the tree that is greater than the given item. The predecessorfunction returns the largest item in the tree that is smaller than the given item. Both functions will return -1 if not found. Note that the predecessor or successor may exist even if the given item is not present in the tree. Use this template: # Node definition provided, please don't modify it.class TreeNode:def __init__(self, val=None):self.val = valself.left = Noneself.right = None# TODO: Please implement the following functions that return an integer# Return the largest value in the tree that is smaller than given value. Return -1 if not found.def predecessor(root, value):pass# Return the smallest value in the tree that is bigger than given value. Return -1 if not found.def successor(root, value):passif __name__ == "__main__":# TODO:…arrow_forward*Please using JAVA only* Objective Program 3: Binary Search Tree Program The primary objective of this program is to learn to implement binary search trees and to combine their functionalities with linked lists. Program Description In a multiplayer game, players' avatars are placed in a large game scene, and each avatar has its information in the game. Write a program to manage players' information in a multiplayer game using a Binary Search (BS) tree for a multiplayer game. A node in the BS tree represents each player. Each player should have an ID number, avatar name, and stamina level. The players will be arranged in the BS tree based on their ID numbers. If there is only one player in the game scene, it is represented by one node (root) in the tree. Once another player enters the game scene, a new node will be created and inserted in the BS tree based on the player ID number. Players during the gameplay will receive hits that reduce their stamina. If the players lose…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
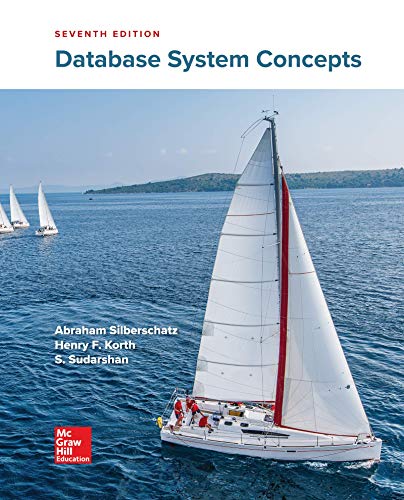
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
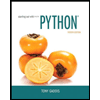
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
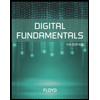
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
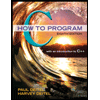
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
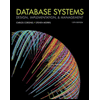
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
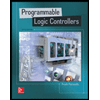
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education