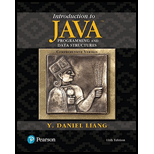
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 25, Problem 25.17PE
Program Plan Intro
Program Plan:
- Include the required import statement.
- Define the main class.
- Declare the necessary variables
- Using start initialize the required.
- Create border pane, tree view, text field and button.
- Set the tree view as center, alignment.
- Add an action event to the button.
- Create a scene and place the pane in the stage.
- Set the title.
- Place the scene in the stage.
- Display the stage.
- Define the main method using public static main.
- Initialize the call.
- Define “decode” method.
- Declare the variable
- Check the length of the “bits”.
- If the “bits” value is “0” set that value into left subtree, if the “bits” value is “1” set the value into right subtree.
- If the left subtree is null, leaf is detected and restart from the root.
- Return the “result”.
- Define “encode” method.
- Declare and set the value.
- Check the length of the “text”.
- Calculate the result.
- Return the “result”.
- Define “getCode” method.
- Check the “root” value is null
- The variable “codes” is declared and allocates memory for that variable.
- Call the “assignCode” method.
- Return the “codes” value.
- Define “assignCode” method.
- Check if root of left subtree value is not null.
- If the condition is true, calculate the left and right subtree value and call the “assignCode” method with the respective parameters.
- Otherwise calculate the “root” element value.
- Check if root of left subtree value is not null.
- Define “getHuffmanTree” method.
- Create a heap to hold the trees.
- Add the values into the heap tree.
- If the heap tree is greater than 1, remove the smallest and next smallest weight from the tree and combine the two trees.
- Finally return the tree.
- Define “getCharacterFrequency” method.
- Declare the variable.
- The try-catch block is used to check the input file is present or not and count the number of characters in that file.
- Return the total counts.
- Define “TreeView” class.
- Declare the required variables.
- Define the constructor.
- Define the “setTree” method.
- Add the value.
- Call the “repaint” method.
- Define the “repaint” method.
- Clear the pane
- Display the tree recursively.
- Define the “displayTree” method.
- Check if the left node value is not equal to null.
- Draw a line to the up node.
- Draw the left subtree recursively.
- Check if the right node value is not equal to null.
- Draw a line to the down node.
- Draw the left subtree recursively.
- Display the node.
- Check if the left node value is not equal to null.
- Define “Tree” class.
- Declare the variable.
- Create a tree with two subtrees,
- Create a tree containing a leaf node.
- Compare the trees based on their weights.
- Define “Node” class.
- Declare the required variables.
- Create a default constructor.
- Create a node with the particular weight and character.
- Define “Heap” class.
- Create the object for the ArrayList
- Create the default constructor.
- Create a heap from an array of objects.
- Define “add” method.
- Add the new object into the heap.
- Check the “currentIndex” value is greater than 0.
- Swap if the current object is greater than its parents.
- Assign “parentIndex” value into “currentIndex” variable.
- Define “remove” method.
- Check the condition and remove the root from the heap.
- Declare and compute the left and right child index.
- Then find the maximum value between two children.
- Swap if the current node is less than maximum value.
- Finally return the value.
Expert Solution & Answer

Trending nowThis is a popular solution!

Students have asked these similar questions
a) Write a program that asks user to enter number of vertices in an undirected graph and
then the adjacency matrix representing the undirected graph. The program, then, must
display whether the given graph is connected or not.
You will find two sample runs of the program below.
Sample 1
Sample 2
Enter number of vertices: 3
Enter number of vertices: 3
Enter adjacency matrix:
0 1 1
1 0 0
1 0 0
Enter adjacency matrix:
0 1 0
1 0 0
0 0 0
The graph is connected.
The graph is not connected.
Data structure/ C language / Graph / Dijkstra’s algorithm
implement a solution of a very common issue: how to get from one town to another using the shortest route.* design a solution that will let you find the shortest paths between two input points in a graph, representing cities and towns, using Dijkstra’s algorithm. Your program should allow the user to enter the input file containing information of roads connecting cities/towns. The program should then construct a graph based on the information provided from the file. The user should then be able to enter pairs of cities/towns and the algorithm should compute the shortest path between the two cities/towns entered.Attached a file containing a list of cities/towns with the following data:Field 1: Vertex ID of the 1st end of the segmentField 2: Vertex ID of the 2nd of the segmentField 3: Name of the townField 4: Distance in Kilometer Please note that all roads are two-ways. Meaning, a record may represent both the roads from…
a) Write a program that asks user to enter number of vertices in a directed graph and thenthe adjacency matrix representing the directed graph. The program, then, must display thenode with the highest outdegree. Assume that nodes are named as 0, 1, 2 and so on.
Chapter 25 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 25.2 - Prob. 25.2.1CPCh. 25.2 - Prob. 25.2.2CPCh. 25.2 - Prob. 25.2.3CPCh. 25.2 - Prob. 25.2.4CPCh. 25.2 - Prob. 25.2.5CPCh. 25.3 - Prob. 25.3.1CPCh. 25.3 - Prob. 25.3.2CPCh. 25.3 - Prob. 25.3.3CPCh. 25.3 - Prob. 25.3.4CPCh. 25.4 - Prob. 25.4.1CP
Ch. 25.4 - Prob. 25.4.2CPCh. 25.4 - Prob. 25.4.3CPCh. 25.4 - Prob. 25.4.4CPCh. 25.4 - Prob. 25.4.5CPCh. 25.5 - Prob. 25.5.1CPCh. 25.5 - Prob. 25.5.2CPCh. 25.5 - Prob. 25.5.3CPCh. 25.5 - Prob. 25.5.4CPCh. 25.5 - Prob. 25.5.5CPCh. 25.6 - Prob. 25.6.1CPCh. 25.6 - Prob. 25.6.2CPCh. 25.6 - Prob. 25.6.3CPCh. 25.6 - How do you replace lines 9499 in Listing 25.11...Ch. 25 - Prob. 25.1PECh. 25 - (Implement inorder traversal without using...Ch. 25 - (Implement preorder traversal without using...Ch. 25 - (Implement postorder traversal without using...Ch. 25 - Prob. 25.6PECh. 25 - Prob. 25.7PECh. 25 - (Implement bidirectional iterator) The...Ch. 25 - Prob. 25.9PECh. 25 - Prob. 25.10PECh. 25 - Prob. 25.11PECh. 25 - (Test BST) Design and write a complete test...Ch. 25 - (Modify BST using Comparator) Revise BST in...Ch. 25 - Prob. 25.15PECh. 25 - (Data compression: Huffman coding) Write a program...Ch. 25 - Prob. 25.17PECh. 25 - (Compress a file) Write a program that compresses...Ch. 25 - (Decompress a file) The preceding exercise...
Knowledge Booster
Similar questions
- PYTHON PORGRAM - Write the syntax for defining a for loop (loop index j) that counts backwards from 10 to -10 in steps of 2 and prints the current loop index to screen.arrow_forwardD. If the values of A, B , C and D are 2, 3, 4 and 5 respectively, manually calculate the value of the following postfix expressions using stack. a. AB*C-D+ b. ABC +*D- ||arrow_forwardString Pair // Problem Description // One person hands over the list of digits to Mr. String, But Mr. String understands only strings. Within strings also he understands only vowels. Mr. String needs your help to find the total number of pairs which add up to a certain digit D. // The rules to calculate digit D are as follow // Take all digits and convert them into their textual representation // Next, sum up the number of vowels i.e. {a, e, i, o, u} from all textual representation // This sum is digit D // Now, once digit D is known find out all unordered pairs of numbers in input whose sum is equal to D. Refer example section for better understanding. // Constraints // 1 <= N <= 100 // 1 <= value of each element in second line of input <= 100 // Number 100, if and when it appears in input should be converted to textual representation as hundred and not as one hundred. Hence number…arrow_forward
- Part 3 plase skip it if you dont know the correct answer i need it urgent. Will doewnvote in case of wrong or copied answers from chegg or bartleby! A regular expression (shortened as regex or regexp; also referred to as rational expression) is a sequence of characters that specifies a search pattern. Usually such patterns are used by string-searching algorithms for "find" or "find and replace" operations on strings, or for input validation. using regex create a python program to verify the string having each word start from 'f'? It should return True otherwise false.arrow_forwardExercise Objectives Problem Description Write a program that reads a string and mirrors it around the middle character. Examples: abcd becomes cdab. abcde becomes deCab AhmadAlami becomes AlamiAhmad Page 1 of 2 Your program must: • Implement function void reflect (char* str) which receives a string (array of characters) and mirrors it. This function does not print anything. • Read from the user (in main()) a string and then print the string after calling function reflect(). • Use pointers and pointer arithmetic only. The use of array notation and/or functions from the string.h library is not allowed.arrow_forwardWhen the * operator’s left operand is a list and its right operand is an integer, the operator becomes this. a. The multiplication operator b. The repetition operator c. The initialization operator d. Nothing—the operator does not support those types of operands.arrow_forward
- def swap_text(text): Backstory: Luffy wants to organize a surprise party for his friend Zoro and he wants to send a message to his friends, but he wants to encrypt the message so that Zoro cannot easily read it. The message is encrypted by exchanging pairs of characters. Description: This function gets a text (string) and creates a new text by swapping each pair of characters, and returns a string with the modified text. For example, suppose the text has 6 characters, then it swaps the first with the second, the third with the fourth and the fifth with the sixth character. Parameters: text is a string (its length could be 0)Return value: A string that is generated by swapping pairs of characters. Note that if the Examples: swap_text ("hello") swap_text ("Party for Zoro!") swap_text ("") def which_day(numbers): → 'ehllo'→ 'aPtr yof roZor!' → '' length of the text is odd, the last character remains in the same position.arrow_forwardii) WAP to demonstrate the use of foreach loop on an array of strings, declare an array of strings and print all strings in output using foreach loop only. In Perl programming language.arrow_forward7. The following function converts a postfix expression to an infix expression assuming the expression can only contain unsigned integer numbers and +' and -' operators. 1. public static String convert (String postfix) { 2. String operand1, operand2, infix; 3. Stack s = new AStack; 4. int i = 0; 5. while (i < postfix.length) { char nextc = postfix.charAt (i); if ((nextc 6. 7. '+')|| (nextc == '-') { == operand2 = s.pop (); operandl = s.pop () ; infix = '(' + operandl + nextc + operand2 + ')'; s.push (infix); i++; 8. 9. 10. 11. } 12. else if (Character.isDigit (nextc)){ int start = i; while (Character.isDigit (postfix.charAt (i)) i++; infix = postfix.substring (start, i); s.push (infix); 13. 14. 15. 16. 17. } else i++; 18. 19. } 20 return s.pop () ; 21. } (a) Rewrite only the lines of code that need to be changed so that the above function converts a postfix expression to a prefix expression. (b) Describe in words what changes are needed on the above given convert () function so that it…arrow_forward
- flip_matrix(mat:list)->list You will be given a single parameter a 2D list (A list with lists within it) this will look like a 2D matrix when printed out, see examples below. Your job is to flip the matrix on its horizontal axis. In other words, flip the matrix horizontally so that the bottom is at top and the top is at the bottom. Return the flipped matrix. To print the matrix to the console: print('\n'.join([''.join(['{:4}'.format(item) for item in row]) for row in mat])) Example: Matrix: W R I T X H D R L G L K F M V G I S T C W N M N F Expected: W N M N F G I S T C L K F M V H D R L G W R I T X Matrix: L C S P Expected: S P L C Matrix: A D J A Q H J C I Expected: J C I A Q H A D Jarrow_forwardData structure & Algorithum Write code that accomplishes the following tasks: Consider two bags that can hold strings. One bag is name letters and contain several one-letter strings. The other bag is empty and is named vowels. One at a time, remove a string from letters. If the strings contain a vowel, place it into the bag vowels; otherwise, discard the string. After you have checked all of the strings in letters, report the number of vowels in the bag vowels and the number of times each vowel appears in the bag. 1. Declare and initialize Bag object named letters.2. Add several one-letter strings to letter object3. Declare and initialize another Bag object vowels.4. Removed a atring from the letters object5. If the removed string is a vowel, add that vowel to the vowel Bag object.6. Repeat removed and vowel check for entire Bag.7. Display the number of vowels in the Bag.8. Display the frequency of occurrences each vowel appears.arrow_forwardsloc) 1. # Given an encoded string, return it's decoded string. # The encoding rule is: k[encoded_string], where the encoded_string# inside the square brackets is being repeated exactly k times.# Note that k is guaranteed to be a positive integer. # You may assume that the input string is always valid; No extra white spaces,# square brackets are well-formed, etc. # Furthermore, you may assume that the original data does not contain any# digits and that digits are only for those repeat numbers, k.# For example, there won't be input like 3a or 2[4]. # Examples: # s = "3[a]2[bc]", return "aaabcbc".# s = "3[a2[c]]", return "accaccacc".# s = "2[abc]3[cd]ef", return "abcabccdcdcdef".arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
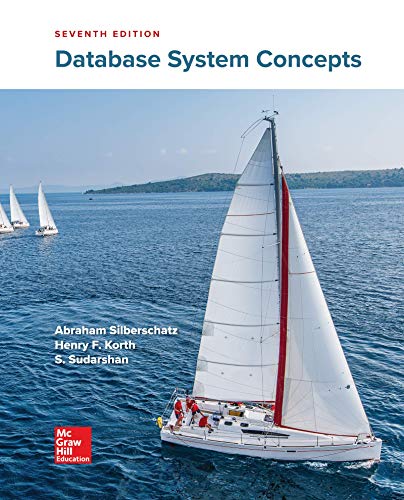
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
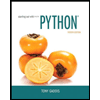
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
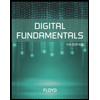
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
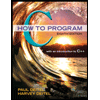
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
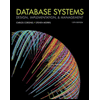
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
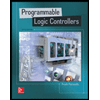
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education