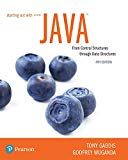
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
4th Edition
ISBN: 9780134787961
Author: Tony Gaddis, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 18.4, Problem 18.32CP
Program Plan Intro
“SortedMap” class:
- The “SortedMap” is inherited from the “Map” interface which ensures that the entries are maintained in an ascending key order.
- The main function of a “SortedMap” is order the key by their natural order or by a particular comparator.
- It does not permit null key or null value.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
At what point is it appropriate to build an index by hand?
You are writing an application for the 911 dispacher office for a city. You need a data structure that will allow you to store all the residents' phone numbers and addresses. The common operations performed on the set of objects are: lookup an address based on the calling phone number. The client wants the program to be as efficient as possible. Which of the following data structures should you use in your design?
Question 43 options:
Hash table
Binary search tree
Heap
Sorted linked List
shared ptr sPtr { make_unique () };
Should we expect an error? Why?
Chapter 18 Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Ch. 18.1 - Prob. 18.1CPCh. 18.1 - What are the three general types of collections?Ch. 18.1 - Prob. 18.3CPCh. 18.1 - Prob. 18.4CPCh. 18.1 - Prob. 18.5CPCh. 18.1 - Prob. 18.6CPCh. 18.1 - Prob. 18.7CPCh. 18.2 - Prob. 18.8CPCh. 18.2 - Prob. 18.9CPCh. 18.2 - Prob. 18.10CP
Ch. 18.2 - Prob. 18.11CPCh. 18.2 - Prob. 18.12CPCh. 18.2 - Prob. 18.13CPCh. 18.2 - Prob. 18.14CPCh. 18.2 - Prob. 18.16CPCh. 18.2 - Prob. 18.17CPCh. 18.2 - Prob. 18.18CPCh. 18.2 - Prob. 18.20CPCh. 18.3 - Prob. 18.21CPCh. 18.3 - Prob. 18.22CPCh. 18.3 - Prob. 18.23CPCh. 18.3 - Prob. 18.24CPCh. 18.3 - Any time you override the Object classs equals...Ch. 18.3 - Prob. 18.26CPCh. 18.3 - Prob. 18.27CPCh. 18.3 - Prob. 18.28CPCh. 18.4 - Prob. 18.29CPCh. 18.4 - Prob. 18.31CPCh. 18.4 - Prob. 18.32CPCh. 18.6 - How do you define a stream of elements?Ch. 18.6 - How does a stream intermediate operation differ...Ch. 18.6 - Prob. 18.35CPCh. 18.6 - Prob. 18.36CPCh. 18.6 - Prob. 18.37CPCh. 18.6 - Prob. 18.38CPCh. 18.6 - Prob. 18.39CPCh. 18 - Prob. 1MCCh. 18 - Prob. 2MCCh. 18 - This type of collection is optimized for...Ch. 18 - Prob. 4MCCh. 18 - A terminal operation in a stream pipeline is also...Ch. 18 - Prob. 6MCCh. 18 - Prob. 7MCCh. 18 - This List Iterator method replaces an existing...Ch. 18 - Prob. 9MCCh. 18 - Prob. 10MCCh. 18 - This is an object that can compare two other...Ch. 18 - This class provides numerous static methods that...Ch. 18 - Prob. 13MCCh. 18 - Prob. 14MCCh. 18 - Prob. 15TFCh. 18 - Prob. 16TFCh. 18 - Prob. 17TFCh. 18 - Prob. 18TFCh. 18 - Prob. 19TFCh. 18 - Prob. 20TFCh. 18 - Prob. 21TFCh. 18 - Prob. 22TFCh. 18 - Prob. 1FTECh. 18 - Prob. 2FTECh. 18 - Prob. 3FTECh. 18 - Prob. 4FTECh. 18 - Write a statement that declares a List reference...Ch. 18 - Prob. 2AWCh. 18 - Assume that it references a newly created iterator...Ch. 18 - Prob. 4AWCh. 18 - Prob. 2SACh. 18 - Prob. 4SACh. 18 - Prob. 5SACh. 18 - Prob. 6SACh. 18 - How does the Java compiler process an enhanced for...Ch. 18 - Prob. 8SACh. 18 - Prob. 9SACh. 18 - Prob. 10SACh. 18 - Prob. 11SACh. 18 - Prob. 12SACh. 18 - Prob. 13SACh. 18 - Prob. 14SACh. 18 - Word Set Write an application that reads a line of...Ch. 18 - Prob. 3PCCh. 18 - Prob. 5PCCh. 18 - Prob. 8PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Create an ordering iterator named OrderedIterator to sort the stream of values given by another iterator. Although you may assume that the basic Iterator would eventually run out of elements, you may not limit the number.arrow_forwardWhat are the different ways in naming sets? Give1 example each.arrow_forwardWhich of the following has its elements always sorted based on some criteria? PriorityQueue Queue O ArrayList Stackarrow_forward
- def city_dict(adict): """ Question 6 -Given a dictionary that maps a person to a list of countries they want to travel to, return a dictionary with the value being the list sorted by the last letter in each countries name. If two countries have the same last letter, sort by the first letter. -For an extra challenge, try doing this in one line (Optional). Args: adict (dict) Returns: dict >>> city_dict({"Pablo": ["Belgium", "Canada", "Germany"], "Athena": ["Italy", "France", "Egypt"], "Liv": ["Japan", "Bolivia", "Greece"]}) {'Pablo': ['Canada', 'Belgium', 'Germany'], 'Athena': ['France', 'Egypt', 'Italy'], 'Liv': ['Bolivia', 'Greece', 'Japan']} >>> city_dict({"Jacob": ["Bahamas", "Brazil", "Chile"], "Lexi": ["Colombia", "Finland", "Panama"], "Emily": ["Ireland", "Russia", "Kenya", "Jordan"]}) {'Jacob': ['Chile', 'Brazil', 'Bahamas'], 'Lexi': ['Colombia', 'Panama', 'Finland'], 'Emily': ['Kenya',…arrow_forward185e def find(self, key): 186e 187 188 Efficiency: 189 Finds and returns a copy of value in the set that matches key. Use: value = source.find(key) 190 191 192 193 Parameters: 194 key - a partial data element (?) 195 Returns: 196 value - a copy of the full value matching key, otherwise None (?) 197 198 199 200 # your code here 201arrow_forwardFrom the following code that collects the data entered by the user of the animals according to their type of food. Pastebin: https://pastebin.com/VuBfRp0s I have an error when deleting animals. It asks me for the code and when I enter it, it says that it deletes the animal. However, it does not disappear from the list and the same animal continues to be printed. What I can do?arrow_forward
- individual characters using their 0-based index. Hint: You will need to do this for checking all the rules. However, when you access a character .arrow_forwardstruct insert_into_hash_table { // Function takes a constant Book as a parameter, inserts that book indexed by // the book's ISBN into a hash table, and returns nothing. void operator()(const Book& book) { ///// /// TO-DO (8) ||||. // Write the lines of code to insert the key (book's ISBN) and value // ("book") pair into "my_hash_table". /// END-TO-DO (8) // } std::unordered_map& my_hash_table; };arrow_forwardYou are writing an application. You need a data structure that will allow you to insert new elements. In addition, you must sometimes remove the maximum element and other times simply report the value of the maximum element. The client wants the program to be as efficient as possible. Which of the following data structures should you use in your design? Question 24 options: Unsorted linked list Hash table Array Sorted linked list Heaparrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
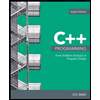
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning