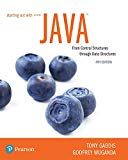
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
4th Edition
ISBN: 9780134787961
Author: Tony Gaddis, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 18, Problem 3AW
Assume that it references a newly created iterator for a list of String objects. Write code that uses the iterator to display each of the String objects in the list.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
public List<String> getLikes(String user)
This will take a String representing a user (like “Mike”) and return a unique List containing all of the users that have liked the user “Mike.”
public List<String> getLikedBy(String user)
This will take a String representing a user (like “Tony”) and return a unique List containing each user that “Tony” has liked.
create a Main to test your work.
import java.io.BufferedReader;import java.io.FileReader;import java.io.IOException;import java.util.ArrayList;import java.util.Arrays;import java.util.HashMap;import java.util.HashSet;import java.util.List;import java.util.Map;import java.util.Set;
public class FacebookLikeManager { public List<String> facebookMap; private Set<String> likesSets;
public FacebookLikeManager() { facebookMap = new ArrayList<>(); likesSets = new HashSet<>(Arrays.asList("Mike","Kristen","Bill","Sara")); }
public void buildMap(String filePath) {…
Assume the names variable references a list of strings. Write code that determines whether 'Ruby' is in the names list. If it is, display the message 'Hello Ruby'. Otherwise, display the message 'No Ruby'.
def swap_text(text):
Backstory:
Luffy wants to organize a surprise party for his friend Zoro and he wants to send a message to his friends, but he wants to encrypt the message so that Zoro cannot easily read it. The message is encrypted by exchanging pairs of characters.
Description: This function gets a text (string) and creates a new text by swapping each pair of characters, and returns a string with the modified text. For example, suppose the text has 6 characters, then it swaps the first with the second, the third with the fourth and the fifth with the sixth character.
Parameters: text is a string (its length could be 0)Return value: A string that is generated by swapping pairs of characters. Note that if the
Examples:
swap_text ("hello") swap_text ("Party for Zoro!") swap_text ("") def which_day(numbers):
→ 'ehllo'→ 'aPtr yof roZor!' → ''
length of the text is odd, the last character remains in the same position.
Chapter 18 Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Ch. 18.1 - Prob. 18.1CPCh. 18.1 - What are the three general types of collections?Ch. 18.1 - Prob. 18.3CPCh. 18.1 - Prob. 18.4CPCh. 18.1 - Prob. 18.5CPCh. 18.1 - Prob. 18.6CPCh. 18.1 - Prob. 18.7CPCh. 18.2 - Prob. 18.8CPCh. 18.2 - Prob. 18.9CPCh. 18.2 - Prob. 18.10CP
Ch. 18.2 - Prob. 18.11CPCh. 18.2 - Prob. 18.12CPCh. 18.2 - Prob. 18.13CPCh. 18.2 - Prob. 18.14CPCh. 18.2 - Prob. 18.16CPCh. 18.2 - Prob. 18.17CPCh. 18.2 - Prob. 18.18CPCh. 18.2 - Prob. 18.20CPCh. 18.3 - Prob. 18.21CPCh. 18.3 - Prob. 18.22CPCh. 18.3 - Prob. 18.23CPCh. 18.3 - Prob. 18.24CPCh. 18.3 - Any time you override the Object classs equals...Ch. 18.3 - Prob. 18.26CPCh. 18.3 - Prob. 18.27CPCh. 18.3 - Prob. 18.28CPCh. 18.4 - Prob. 18.29CPCh. 18.4 - Prob. 18.31CPCh. 18.4 - Prob. 18.32CPCh. 18.6 - How do you define a stream of elements?Ch. 18.6 - How does a stream intermediate operation differ...Ch. 18.6 - Prob. 18.35CPCh. 18.6 - Prob. 18.36CPCh. 18.6 - Prob. 18.37CPCh. 18.6 - Prob. 18.38CPCh. 18.6 - Prob. 18.39CPCh. 18 - Prob. 1MCCh. 18 - Prob. 2MCCh. 18 - This type of collection is optimized for...Ch. 18 - Prob. 4MCCh. 18 - A terminal operation in a stream pipeline is also...Ch. 18 - Prob. 6MCCh. 18 - Prob. 7MCCh. 18 - This List Iterator method replaces an existing...Ch. 18 - Prob. 9MCCh. 18 - Prob. 10MCCh. 18 - This is an object that can compare two other...Ch. 18 - This class provides numerous static methods that...Ch. 18 - Prob. 13MCCh. 18 - Prob. 14MCCh. 18 - Prob. 15TFCh. 18 - Prob. 16TFCh. 18 - Prob. 17TFCh. 18 - Prob. 18TFCh. 18 - Prob. 19TFCh. 18 - Prob. 20TFCh. 18 - Prob. 21TFCh. 18 - Prob. 22TFCh. 18 - Prob. 1FTECh. 18 - Prob. 2FTECh. 18 - Prob. 3FTECh. 18 - Prob. 4FTECh. 18 - Write a statement that declares a List reference...Ch. 18 - Prob. 2AWCh. 18 - Assume that it references a newly created iterator...Ch. 18 - Prob. 4AWCh. 18 - Prob. 2SACh. 18 - Prob. 4SACh. 18 - Prob. 5SACh. 18 - Prob. 6SACh. 18 - How does the Java compiler process an enhanced for...Ch. 18 - Prob. 8SACh. 18 - Prob. 9SACh. 18 - Prob. 10SACh. 18 - Prob. 11SACh. 18 - Prob. 12SACh. 18 - Prob. 13SACh. 18 - Prob. 14SACh. 18 - Word Set Write an application that reads a line of...Ch. 18 - Prob. 3PCCh. 18 - Prob. 5PCCh. 18 - Prob. 8PC
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
What two questions should you ask to determine a classs responsibilities?
Starting Out with C++: Early Objects
Explain why software testing should always be an incremental, staged activity. Are programmers the best people ...
Software Engineering (10th Edition)
Describe the primary differences between the conceptual and logical data models.
Modern Database Management (12th Edition)
Explain how entities are transformed into tables.
Database Concepts (7th Edition)
T F When a function terminates, it always branches back to main, regardless of where it was called from.
Starting Out with C++ from Control Structures to Objects (9th Edition)
Name the steps in the programming process.
Digital Fundamentals (11th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Write in Java pleasearrow_forwarddetemine if the following statement are true or false The iterator operation is required by the Iterable interface. A list allows retrieval of information based on the contents of the information.arrow_forwardLists: Create a list of student names. These are strings representing the names of students in your class (e.g., ["John", "Mary", "Peter", "Sam"]). 2. Tuples: For each student, create a tuple that stores their grades in different subjects. The subjects are Mathematics, English, and Science (e.g., (85, 90, 88)). 3. Dictionaries: Now, create a dictionary where the keys are the names of the students (from your list), and the values are the tuples storing their grades. This way, you can easily look up a student's grades in different subjects.arrow_forward
- Python write a program in python that plays the game of Hangman. When the user plays Hangman, the computer first selects a secret word at random from a list built into the program. The program then prints out a row of dashes asks the user to guess a letter. If the user guesses a letter that is in the word, the word is redisplayed with all instances of that letter shown in the correct positions, along with any letters correctly guessed on previous turns. If the letter does not appear in the word, the user is charged with an incorrect guess. The user keeps guessing letters until either: * the user has correctly guessed all the letters in the word or * the user has made eight incorrect guesses. one for each letter in the secret word and Hangman comes from the fact that incorrect guesses are recorded by drawing an evolving picture of the user being hanged at a scaffold. For each incorrect guess, a new part of a stick-figure body the head, then the body, then each arm, each leg, and finally…arrow_forward#include <iostream> #include "ContactNode.h" int main() { ContactNode* contactList = new ContactNode(); ContactNode* currNode; std::string name, phoneNum; int i = 1; while (i <= 3) { std::cout << "Person " << i++ << std::endl; std::cout << "Enter name:" << std::endl; getline(std::cin, name); std::cout << "Enter phone number:" << std::endl; std::cin >> phoneNum; std::cin.ignore(); contactList->InsertAfter(new ContactNode(name, phoneNum)); std::cout << "You entered: " << name << ", " << phoneNum << std::endl << std::endl; } currNode = contactList; std::cout << "CONTACT LIST" << std::endl; while (contactList != nullptr) { currNode->PrintContactNode(); currNode = currNode->GetNext(); } delete contactList, currNode; } // ContactNode.h #ifndef CONTACTNODE_H #define CONTACTNODE_H #include <string> class ContactNode { public: ContactNode(std::string…arrow_forwardWord Separator Write a program that accepts as input a sentence in which all of the words are run together but the first character of each word is uppercase. Convert the sentence to a string in which the words are separated by spaces and only the first word starts with an uppercase letter. For example the string “StopAndSmellTheRoses.” would be converted to “Stop and smell the roses.” LINUX !#/bin/basharrow_forward
- string txt = "Noor"; cout 1: . "The ;. .length of the txt string is: " >arrow_forwardStatic Length, Limited Dynamic Length, and Dynamic Length String implementations have what advantages and disadvantages?arrow_forwardYahtzee! Yahtzee is a dice game that uses five die. There are multiple scoring abilities with the highest being a Yahtzee where all five die are the same. You will simulate rolling five die 777 times while looking for a yahtzee. Program Specifications : Create a list that holds the values of your five die. Populate the list with five random numbers between 1 & 6, the values on a die. Create a function to see if all five values in the list are the same and IF they are, print the phrase "You rolled ##### and its a Yahtzee!" (note: ##### will be replaced with the values in the list) Create a loop that completes the process 777 times, simulating you rolling the 5 die 777 times, checking for Yahtzee, and printing the statement above when a Yahtzee is rolled. When you complete the project please upload your .py file to the Project 2 folder by the due date.arrow_forward
- What characters are utilised as delimiters when you supply null as a parameter to the Split method of a string object?arrow_forwardWAP c# program creates a string, s1, which deliberately leaves space for a name, much like you’d do with a letter you plan to run through a mail merge. We add two to the position where we find the comma to make sure there is a space between the comma and the name.arrow_forwardC++arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
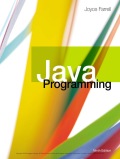
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Introduction to Linked List; Author: Neso Academy;https://www.youtube.com/watch?v=R9PTBwOzceo;License: Standard YouTube License, CC-BY
Linked list | Single, Double & Circular | Data Structures | Lec-23 | Bhanu Priya; Author: Education 4u;https://www.youtube.com/watch?v=IiL_wwFIuaA;License: Standard Youtube License