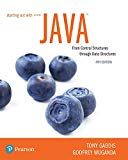
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
4th Edition
ISBN: 9780134787961
Author: Tony Gaddis, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Expert Solution & Answer
Chapter 18, Problem 3FTE
Explanation of Solution
Given code:
//implement the "Set" interface
Set <String> nameSet = new HashSet<>(); //Line 1
//add the values into the "Set"
nameSet.add("Chris"); //Line 2
nameSet.add("Kenny"); //Line 3
//declare the "ListIterator"
ListIterator it = nameSet.listIterator();//Line 4
Error in the code:
Error #1:
The error is in “Line 4”. The user can’t get a “ListIterator” from the object, which implements the “Set” interface...
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
solve this questions for me .
a) first player is the minimizing player. What move should be chosen?b) What nodes would not need to be examined using the alpha-beta pruning procedure?
Consider the problem of finding a path in the grid shown below from the position S to theposition G. The agent can move on the grid horizontally and vertically, one square at atime (each step has a cost of one). No step may be made into a forbidden crossed area. Inthe case of ties, break it using up, left, right, and down.(a) Draw the search tree in a greedy search. Manhattan distance should be used as theheuristic function. That is, h(n) for any node n is the Manhattan distance from nto G. The Manhattan distance between two points is the distance in the x-directionplus the distance in the y-direction. It corresponds to the distance traveled along citystreets arranged in a grid. For example, the Manhattan distance between G and S is4. What is the path that is found by the greedy search?(b) Draw the search tree in an A∗search. Manhattan distance should be used as the
Chapter 18 Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Ch. 18.1 - Prob. 18.1CPCh. 18.1 - What are the three general types of collections?Ch. 18.1 - Prob. 18.3CPCh. 18.1 - Prob. 18.4CPCh. 18.1 - Prob. 18.5CPCh. 18.1 - Prob. 18.6CPCh. 18.1 - Prob. 18.7CPCh. 18.2 - Prob. 18.8CPCh. 18.2 - Prob. 18.9CPCh. 18.2 - Prob. 18.10CP
Ch. 18.2 - Prob. 18.11CPCh. 18.2 - Prob. 18.12CPCh. 18.2 - Prob. 18.13CPCh. 18.2 - Prob. 18.14CPCh. 18.2 - Prob. 18.16CPCh. 18.2 - Prob. 18.17CPCh. 18.2 - Prob. 18.18CPCh. 18.2 - Prob. 18.20CPCh. 18.3 - Prob. 18.21CPCh. 18.3 - Prob. 18.22CPCh. 18.3 - Prob. 18.23CPCh. 18.3 - Prob. 18.24CPCh. 18.3 - Any time you override the Object classs equals...Ch. 18.3 - Prob. 18.26CPCh. 18.3 - Prob. 18.27CPCh. 18.3 - Prob. 18.28CPCh. 18.4 - Prob. 18.29CPCh. 18.4 - Prob. 18.31CPCh. 18.4 - Prob. 18.32CPCh. 18.6 - How do you define a stream of elements?Ch. 18.6 - How does a stream intermediate operation differ...Ch. 18.6 - Prob. 18.35CPCh. 18.6 - Prob. 18.36CPCh. 18.6 - Prob. 18.37CPCh. 18.6 - Prob. 18.38CPCh. 18.6 - Prob. 18.39CPCh. 18 - Prob. 1MCCh. 18 - Prob. 2MCCh. 18 - This type of collection is optimized for...Ch. 18 - Prob. 4MCCh. 18 - A terminal operation in a stream pipeline is also...Ch. 18 - Prob. 6MCCh. 18 - Prob. 7MCCh. 18 - This List Iterator method replaces an existing...Ch. 18 - Prob. 9MCCh. 18 - Prob. 10MCCh. 18 - This is an object that can compare two other...Ch. 18 - This class provides numerous static methods that...Ch. 18 - Prob. 13MCCh. 18 - Prob. 14MCCh. 18 - Prob. 15TFCh. 18 - Prob. 16TFCh. 18 - Prob. 17TFCh. 18 - Prob. 18TFCh. 18 - Prob. 19TFCh. 18 - Prob. 20TFCh. 18 - Prob. 21TFCh. 18 - Prob. 22TFCh. 18 - Prob. 1FTECh. 18 - Prob. 2FTECh. 18 - Prob. 3FTECh. 18 - Prob. 4FTECh. 18 - Write a statement that declares a List reference...Ch. 18 - Prob. 2AWCh. 18 - Assume that it references a newly created iterator...Ch. 18 - Prob. 4AWCh. 18 - Prob. 2SACh. 18 - Prob. 4SACh. 18 - Prob. 5SACh. 18 - Prob. 6SACh. 18 - How does the Java compiler process an enhanced for...Ch. 18 - Prob. 8SACh. 18 - Prob. 9SACh. 18 - Prob. 10SACh. 18 - Prob. 11SACh. 18 - Prob. 12SACh. 18 - Prob. 13SACh. 18 - Prob. 14SACh. 18 - Word Set Write an application that reads a line of...Ch. 18 - Prob. 3PCCh. 18 - Prob. 5PCCh. 18 - Prob. 8PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- whats for dinner? pleasearrow_forwardConsider the follow program that prints a page number on the left or right side of a page. Define and use a new function, isEven, that returns a Boolean to make the condition in the if statement easier to understand. ef main() : page = int(input("Enter page number: ")) if page % 2 == 0 : print(page) else : print("%60d" % page) main()arrow_forwardWhat is the correct python code for the function def countWords(string) that will return a count of all the words in the string string of workds that are separated by spaces.arrow_forward
- Consider the following program that counts the number of spaces in a user-supplied string. Modify the program to define and use a function, countSpaces, instead. def main() : userInput = input("Enter a string: ") spaces = 0 for char in userInput : if char == " " : spaces = spaces + 1 print(spaces) main()arrow_forwardWhat is the python code for the function def readFloat(prompt) that displays the prompt string, followed by a space, reads a floating-point number in, and returns it. Here is a typical usage: salary = readFloat("Please enter your salary:") percentageRaise = readFloat("What percentage raise would you like?")arrow_forwardassume python does not define count method that can be applied to a string to determine the number of occurances of a character within a string. Implement the function numChars that takes a string and a character as arguments and determined and returns how many occurances of the given character occur withing the given stringarrow_forward
- Consider the ER diagram of online sales system above. Based on the diagram answer the questions below, a) Based on the ER Diagram, determine the Foreign Key in the Product Table. Just mention the name of the attribute that could be the Foreign Key. b) Mention the relationship between the Order and Customer Entities. You can use the following: 1:1, 1:M, M:1, 0:1, 1:0, M:0, 0:M c) Is there a direct relationship that exists between Store and Customer entities? Answer Yes/No? d) Which of the 4 Entities mention in the diagram can have a recursive relationship? e) If a new entity Order_Details is introduced, will it be a strong entity or weak entity? If it is a weak entity, then mention its type?arrow_forwardNo aiarrow_forwardGiven the dependency diagram of attributes {C1,C2,C3,C4,C5) in a table shown in the following figure, (the primary key attributes are underlined)arrow_forward
- What are 3 design techniques that enable data representations to be effective and engaging? What are some usability considerations when designing data representations? Provide examples or use cases from your professional experience.arrow_forward2D array, Passing Arrays to Methods, Returning an Array from a Method (Ch8) 2. Read-And-Analyze: Given the code below, answer the following questions. 2 1 import java.util.Scanner; 3 public class Array2DPractice { 4 5 6 7 8 9 10 11 12 13 14 15 16 public static void main(String args[]) { 17 } 18 // Get an array from the user int[][] m = getArray(); // Display array elements System.out.println("You provided the following array "+ java.util.Arrays.deepToString(m)); // Display array characteristics int[] r = findCharacteristics(m); System.out.println("The minimum value is: " + r[0]); System.out.println("The maximum value is: " + r[1]); System.out.println("The average is: " + r[2] * 1.0/(m.length * m[0].length)); 19 // Create an array from user input public static int[][] getArray() { 20 21 PASSTR2222322222222222 222323 F F F F 44 // Create a Scanner to read user input Scanner input = new Scanner(System.in); // Ask user to input a number, and grab that number with the Scanner…arrow_forwardGiven the dependency diagram of attributes C1,C2,C3,C4,C5 in a table shown in the following figure, the primary key attributes are underlined Make a database with multiple tables from attributes as shown above that are in 3NF, showing PK, non-key attributes, and FK for each table? Assume the tables are already in 1NF. Hint: 3 tables will result after deducing 1NF -> 2NF -> 3NFarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
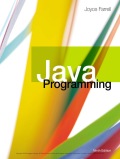
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
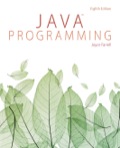
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
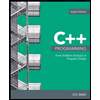
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
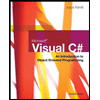
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
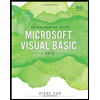
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning