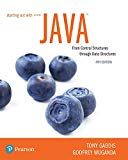
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
4th Edition
ISBN: 9780134787961
Author: Tony Gaddis, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Expert Solution & Answer
Chapter 16.1, Problem 16.3CP
Explanation of Solution
Selection sort:
- This is sorting
algorithm in which it finds out the smallest value in the array and moves that element to position “0”. - Then, it finds out the next smallest value and moves the element to position “1”.
- This process is continued until all the elements are placed in proper order.
Example:
Consider the elements to sort (8, 5, 1, 2, 7 )
- Find the smallest element in the array by comparing all the values and place it in the first position.
- Find the next smallest element in the array by comparing the values and place it in second position.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Mina always wants things to be sorted. She loves perfection in work
and doesn't worry about time. Her brother gifted her some boxes
with different sizes. Now she needs your help to sort it.
You're given n boxes with different sizes and your task is to sort
the boxes in ascending order of size. You should recursively
partition the array with a number p so that all the elements
greater than p are to its right and all the elements lower than p to
its left to sort the boxes. Make sure you are giving a solution that
doesn't exceed the time complexity of O(n^2), but on average has
complexity of O(nlogn)
a. Which algorithm will you suggest to Mina? Explain if your
algorithm is stable or unstable with appropriate example
b. Show the step by step simulation of your algorithm on the
boxes of size 5, 9, 10, 3, 15, 12, 6, 20, 9, 13, 3, 10, 1,
2.
c. Give an example of box sizes when it will be worst case and
when it will be the best case for this algorithm.
A sort algorithm that finds the smallest element of the array and interchanges it with the
element in the first position of the array. Then it finds the second smallest element from the
remaining elements in the array and places it in the second position of the array and so on
Compute the distance between the positions of the first and last zero element in the given array. For example, if the array is
3 0 1 0 4 9 5 0 6
your algorithm should yield 7 - 1 = 6.
If the array contains a single zero, return 0. If it doesn't contain any zeroes, return a negative value.
Chapter 16 Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Ch. 16.1 - Prob. 16.1CPCh. 16.1 - Prob. 16.2CPCh. 16.1 - Prob. 16.3CPCh. 16.1 - Prob. 16.4CPCh. 16.2 - Prob. 16.5CPCh. 16.2 - Prob. 16.6CPCh. 16.2 - Prob. 16.7CPCh. 16.2 - If a sequential search is performed on an array,...Ch. 16.3 - Prob. 16.9CPCh. 16.3 - Prob. 16.10CP
Ch. 16.3 - Prob. 16.11CPCh. 16.3 - Prob. 16.12CPCh. 16.3 - Prob. 16.13CPCh. 16.3 - Prob. 16.14CPCh. 16.3 - Let a[ ] and b[ ] be two integer arrays of size n....Ch. 16.3 - Prob. 16.16CPCh. 16.3 - Prob. 16.17CPCh. 16.3 - Prob. 16.18CPCh. 16 - Prob. 1MCCh. 16 - Prob. 2MCCh. 16 - Prob. 3MCCh. 16 - Prob. 4MCCh. 16 - Prob. 5MCCh. 16 - Prob. 6MCCh. 16 - Prob. 7MCCh. 16 - Prob. 8MCCh. 16 - Prob. 9MCCh. 16 - Prob. 10MCCh. 16 - True or False: If data is sorted in ascending...Ch. 16 - True or False: If data is sorted in descending...Ch. 16 - Prob. 13TFCh. 16 - Prob. 14TFCh. 16 - Assume this code is using the IntBinarySearcher...Ch. 16 - Prob. 1AWCh. 16 - Prob. 1SACh. 16 - Prob. 2SACh. 16 - Prob. 3SACh. 16 - Prob. 4SACh. 16 - Prob. 5SACh. 16 - Prob. 6SACh. 16 - Prob. 7SACh. 16 - Prob. 8SACh. 16 - Prob. 1PCCh. 16 - Sorting Objects with the Quicksort Algorithm The...Ch. 16 - Prob. 3PCCh. 16 - Charge Account Validation Create a class with a...Ch. 16 - Charge Account Validation Modification Modify the...Ch. 16 - Search Benchmarks Write an application that has an...Ch. 16 - Prob. 8PCCh. 16 - Efficient Computation of Fibonacci Numbers Modify...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Write a program that reads the numbers and sorts them by using the Counting Sort algorithm and finally search a number from that array using Linear Search Algorithm. Input: 3 6 5 4 789 Search Item: 7 Output: Sorted Array: 3 4 5 6 789 Search item 7 is found.arrow_forwardWrite a modified version of the selection sort algorithm that selects the largest element each time and moves it to the end of the array, rather than selecting the smallest element and moving it to the beginning. Will this algorithm be faster than the standard selection sort? What will its complexity class (big-Oh) be?arrow_forwardMake a programme that compares the four advanced sorting algorithms covered in this chapter. Create a 1,000-element randomly generated array for the tests. What is the algorithm's ranking? What happens when the array size is increased to 10,000, then 100,000 elements?arrow_forward
- Sort the array (D, G, J, F, A, C) using selection sort (show the array after each step).arrow_forwardDouble Insertion Sort is a variation on Insertion Sort that works from the middle of the array out. At each iteration, some middle portion of the array is sorted. On the next iteration, take the two adjacent elements to the sorted portion of the array. If they are out of order with respect to each other, then swap them. Now, push the left element toward the right in the array so long as it is greater than the element to its right. And push the right element toward the left in the array so long as it is less than the element to its left. The algorithm begins by processing the middle two elements of the array if the array is even. If the array is odd, then skip processing the middle item and begin with processing the elements to its immediate left and right. Implement Double Insertion Sort, being careful to properly handle both when the array is odd and when it is even. By using java, Implement the Double Insertion sort algorithm on a randomly generated list of N integer numbers. Your…arrow_forwardDouble Insertion Sort is a variation on Insertion Sort that works from the middle of the array out. At each iteration, some middle portion of the array is sorted. On the next iteration, take the two adjacent elements to the sorted portion of the array. If they are out of order with respect to each other, then swap them. Now, push the left element toward the right in the array so long as it is greater than the element to its right. And push the right element toward the left in the array so long as it is less than the element to its left. The algorithm begins by processing the middle two elements of the array if the array is even. If the array is odd, then skip processing the middle item and begin with processing the elements to its immediate left and right. Implement Double Insertion Sort, being careful to properly handle both when the array is odd and when it is even. Implement the Double Insertion sort algorithm by using JAVA on a randomly generated list of N integer Your program…arrow_forward
- Double Insertion Sort is a variation on Insertion Sort that works from the middle of the array out. At each iteration, some middle portion of the array is sorted. On the next iteration, take the two adjacent elements to the sorted portion of the array. If they are out of order with respect to each other, then swap them. Now, push the left element toward the right in the array so long as it is greater than the element to its right. And push the right element toward the left in the array so long as it is less than the element to its left. The algorithm begins by processing the middle two elements of the array if the array is even. If the array is odd, then skip processing the middle item and begin with processing the elements to its immediate left and right. Implement Double Insertion Sort, being careful to properly handle both when the array is odd and when it is even. Improved Bubble Sort: One possible improvement for Bubble Sort would be to add a flag variable and a test that…arrow_forwardUsing Javaarrow_forwardYou can see in the above display, we first sort each row of the 2D array; we then take the transpose of a two D array, i.e., all the row elements becoming the column elements; we then sort each row of the 2D again. If you read the final array, each row is sorted; each column is also sorted. The smallest element obviously is the 1st element of the two D array and the last element is the largest element of a two D array. Let us now look at the following UML diagram: (Note that additional methods are allowed; proposed methods and instance variable cannot be changed) Main method firstly constructs a 2D array of certain sizes and then construct a TwoD object and drive the task according to the above runtime interactions and displays. TwoD class has only one instance variable which is a two D array of numbers ( int or double). The constructor must do some “deep” copying. A copy constructor. The other three methods are obvious in definition: to sort each row, to rotate the 2D array (i.e.,…arrow_forward
- There are many algorithms that are used to solve a variety of problems. In this part, you should write an algorithm that shuffles a given array, the shuffled array should be completely different than the given array, explain your chosen algorithm, describe the algorithm step in pseudo code.arrow_forwardThe binary search algorithm that follows may be used to search an array when the elements are in order. This algorithm is analogous to the following approach to finding a name in a telephone book. a. Open the book in the middle and look at the middle name on the page. b. b. If the middle name isn't the one, you're looking for, decide whether it comes before or after the name you want. c. Take the appropriate half of the section of the book you were looking in and repeat these steps until you land on the name. 1. Let the bottom be the subscript of the initial array element. 2. Let the top be the subscript of the last array element. 3. Let found be false. 4. Repeat as long as the bottom isn't greater than the top and the target has not been found. 5. Let middle be the subscript of the element halfway between bottom and top. 6. If the element in the middle is the target 7. Set found to true and index to middle. else if the element in the middle is larger than the target 8. Let the top be…arrow_forwardSorting this array using java ... finds the smallest number in the list and places it first. It then finds the smallest number remaining and places it second, and so on until the list contains only a single number. The list is (2,9,5,4,8,1,6)arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
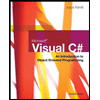
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage