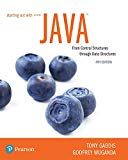
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
4th Edition
ISBN: 9780134787961
Author: Tony Gaddis, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 16, Problem 3PC
Program Plan Intro
Searching for Objects with the Binary search
Program plan:
- Create the class “ObjectBinarySearcher”,
- Define the “search()” method,
- Declare the required variables.
- Use the while loop to check whether the searching value is found in the array or searching reaches end of the array.
- Calculate the middle value.
- Check whether the middle value is at index “0”. If yes, then set the Boolean value for “found” to be “true”.
- Assign the middle value index to “position” variable.
- Check whether the searching value when compared to middle is greater than 0.
- If yes, search the left half of the array otherwise search the right half of the array.
- Return the position of the element.
- Create the class “ObjectBinarySearchTest”,
- Define the “main()” function,
- Assign the string values.
- Read the input from the user through scanner to search the value in the array.
- Call the “quicksort()” method to sort the values.
- Execute the do…while loop to search the value in the array until it iterates all the elements in the array.
- Call the “search()” method to find the searching element in the array.
- If the searching value is found, then print the corresponding message otherwise print the message “element not found in the array”.
- If the user needs to continue the choice to search the element. Press yes or “y” to continue otherwise exit the program.
- Define the “main()” function,
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Functions with 2D Arrays in Java
Write a function named displayElements that takes a two-dimensional array, the size of its rows and columns, then prints every element of a two-dimensional array. Separate every row by a new line and every column by a space.
In the main function, call the displayElements function and pass in the required parameters.
Output
1 2 3
4 5 6
7 8 9
JAVA PLEASE
FAAST
Write a java method that receives a string containing letters of the English alphabet and then prints the Letters whose frequencies are not unique.
Notes:
1) Ignore letter case; Uppercase and lowercase letters are considered the same.
2) Do not use methods from the Arrays class.
Using jGRASP please de-bug this
/**This program demonstrates an array of String objects.It should print out the days and the number of hours spent at work each day.It should print out the day of the week with the most hours workedIt should print out the average number of hours worked each dayThis is what should display when the program runs as it shouldSunday has 12 hours worked.Monday has 9 hours worked.Tuesday has 8 hours worked.Wednesday has 13 hours worked.Thursday has 6 hours worked.Friday has 4 hours worked.Saturday has 0 hours worked.Highest Day is Wednesday with 13 hours workedAverage hours worked in the week is 7.43 hours*/{public class WorkDays{public static void main(String[] args){String[] days = { "Sunday", "Monday", "Tuesday","Wednesday", "Thursday", "Friday", "Saturday"};
int[] hours = { 12, 9, 8, 13, 6, 4};
int average = 0.0;int highest = 0;String highestDay = " ";int sum = 0;
for (int index = 0; index < days.length; index++){System.out.println(days[index] + " has "…
Chapter 16 Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Ch. 16.1 - Prob. 16.1CPCh. 16.1 - Prob. 16.2CPCh. 16.1 - Prob. 16.3CPCh. 16.1 - Prob. 16.4CPCh. 16.2 - Prob. 16.5CPCh. 16.2 - Prob. 16.6CPCh. 16.2 - Prob. 16.7CPCh. 16.2 - If a sequential search is performed on an array,...Ch. 16.3 - Prob. 16.9CPCh. 16.3 - Prob. 16.10CP
Ch. 16.3 - Prob. 16.11CPCh. 16.3 - Prob. 16.12CPCh. 16.3 - Prob. 16.13CPCh. 16.3 - Prob. 16.14CPCh. 16.3 - Let a[ ] and b[ ] be two integer arrays of size n....Ch. 16.3 - Prob. 16.16CPCh. 16.3 - Prob. 16.17CPCh. 16.3 - Prob. 16.18CPCh. 16 - Prob. 1MCCh. 16 - Prob. 2MCCh. 16 - Prob. 3MCCh. 16 - Prob. 4MCCh. 16 - Prob. 5MCCh. 16 - Prob. 6MCCh. 16 - Prob. 7MCCh. 16 - Prob. 8MCCh. 16 - Prob. 9MCCh. 16 - Prob. 10MCCh. 16 - True or False: If data is sorted in ascending...Ch. 16 - True or False: If data is sorted in descending...Ch. 16 - Prob. 13TFCh. 16 - Prob. 14TFCh. 16 - Assume this code is using the IntBinarySearcher...Ch. 16 - Prob. 1AWCh. 16 - Prob. 1SACh. 16 - Prob. 2SACh. 16 - Prob. 3SACh. 16 - Prob. 4SACh. 16 - Prob. 5SACh. 16 - Prob. 6SACh. 16 - Prob. 7SACh. 16 - Prob. 8SACh. 16 - Prob. 1PCCh. 16 - Sorting Objects with the Quicksort Algorithm The...Ch. 16 - Prob. 3PCCh. 16 - Charge Account Validation Create a class with a...Ch. 16 - Charge Account Validation Modification Modify the...Ch. 16 - Search Benchmarks Write an application that has an...Ch. 16 - Prob. 8PCCh. 16 - Efficient Computation of Fibonacci Numbers Modify...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- DNA Max Write code to find which of the strands representing DNA in an array String[] strands representing strands of DNA has the most occurrences of the nucleotide represented by parameter nuc. Complete the definition of the class DnaMax and method definition maxStrand shown below. If more than one strand has the same maximal number of the specified nucleotide you should return the longest strand with the maximal number. All DNA strands have different lengths in this problem so the maximal strand will be unique when length is accounted for. Return this uniquely maximal strand. Each String representing a DNA strand will contain only cytosine, guanine, thymine, and adenine, represented by the characters 'c', 'g', 't', and 'a', respectively. If no strand in the array contains the specified nucleotide return the empty string "". public class DnaMax { public String maxStrand(String[] strands, String nuc) { // fill in code here } } Constraints strands will contain no more than 50 elements,…arrow_forwardRecipe Program - Java ONLYI am looking to create a program that is a recipe holder. It needs to include at a minimum: At least 1 loop An Array or ArrayList At least 3 Java classes Use of methods The program would allow the user to (as part of a menu selection): Add a recipe Include ingredient list Instructions List all recipes that are in the program (array) Display a single recipe Search Recipes (option to view recipe selected) For example, searching for peanut butter cookie would bring up the recipe or multiple if the same name. The user could then select one of them to view the recipe. Search Recipes with a single ingredient (option to view recipe selected) For example: search for coconut and it would bring up coconut cream pie and German chocolate cake. The user could then select one of them to view the recipe. Close application Also need the program in a UML diagram.arrow_forward20- $footboll=array(); is an example of: a. boolean b. None c. function d. empty arrayarrow_forward
- 1. An array is a container that holds a group of values of a 2. Each item in an array is called an 3. Each element is accessed by a numerical 4. The index to the first element of an array is 0 and the index to the last element of the array is the length of the 5. Given the following array declaration, determine which of the three statements below it are true. int [] autoMobile = new int [13]; i. autoMobile[0] is the reference to the first element in the array. ii. autoMobile[13] is the reference to the last element in the array. ii. There are 13 integers in the autoMobile array. 6. In java new is a which is used to allocate memory 7. Declare a one-dimensional array named score of type int that can hold9 values. 8. Declare and initialize a one-dimensional byte array named values of size 10 so that all entries contain 1. 9. Declare and initialize a one-dimensional array to store name of any 3 students. 10. Declare a two-dimensional array named mark of type double that can hold 5x3…arrow_forwardNumber each step please! Create a program that 1. Initializes a game object. 2. Reads a file of signs and stores each sign in an ArrayList<String> in the game object. 3. Reads a file of outcomes, storing each outcome twice in a two-dimensional array in the game object (diagonals should be empty). 4. Prints out the list of signs and the two-dimensional array of outcomes to the screen.arrow_forwardprogramming: C# without using array, while and loops we havent yet tackle those i just need the normal code.arrow_forward
- 1. Given a string of at least 3 characters as input, if the length of the string is odd return the character in the middle as a string. If the string is even return the two characters at the midpoint. public class Class1 { public static String midString(String str) { //Enter code here } } ----------- 2. Given an array of integers return the sum of the values stored in the first and last index of the array. The array will have at least 2 elements in it public class Class1 { public static int endSum(int[] values) { //Enter code here } } ----------- 3. The method takes a string as a parameter. The method prints treat if the string is candy or chocolate (of any case, such as CaNdY) Otherwise print trick import java.util.Scanner; public class Class1 { public static void trickOrTreat(String str) { //Enter code here } public static void main(String[] args) { Scanner s = new Scanner(System.in); trickOrTreat(s.nextLine()); } }arrow_forwardBubble Sorting an Array of ObjectsCreate a Student class and you will need to do the following: Enter the number of students (must be greater than 5). Enter the student’s names and grades Make sure that you enter the names and grades in random order. Iterate through the array of students and using the bubble sort, order the array by grade.arrow_forwardArrays: create an array of a given type and populate its values. Use of for loop to traverse through an array to do the following : to print the elements one by one, to search the array for a given value. ArrayList: create an ArrayList containing elements of a given type . Use some of the common ArrayList methods to manipulate contents of the ArrayList. Write methods that will take an ArrayList as its parameter/argument ; and/or return an ArrayList reference variable as its return type. Searching for an object in an Array: Loop through the ArrayList to extract each object and to check if this object’s attribute has a given value. Explain how and why interfaces are used in Java Collection Framework. Explain the major differences between a Stack and a Queue. Be able to use stack and queue methods. What is meant by O(N) notation? Express the complexity of a given code using the O(N) notation.arrow_forward
- How do I code: public class PromptBank { String [] questions; String [] statements; public PromptBank(){ //questions = new String[ ]; //initialize your array to the correct length to match your number of questions you populate it with //statements = //initialize your array to the correct length to match your number of questions you populate it with } public void populateStatementsArray(){ questions[0] = "Tell me more about BLANK1 and BLANK2"; questions[1] = "BLANK1 seems important to you, so does BLANK2. Please tell me more."; questions[2] = "BLANK1 and BLANK2 seem to be on your mind. Let's talk about it."; /*complete this method with your other statements using BLANK1 for word1 * and BLANK2 for word2 place holder */ } public void populateQuestionsArray(){ questions[0] = "Is there anything else about BLANK1 and BLANK2?"; questions[1] = "Does BLANK1 bother you? How about…arrow_forward8. Grade Book A teacher has five students who have taken four tests. The teacher uses the following grading scale to assign a letter grade to a student, based on the average of his or her four test scores: Test Score Letter Grade 90–100 A 80-89 B 70-79 C 60-69 D 0-59 Write a class that uses a String array or an ArrayList object to hold the five students' names, an array of five characters to hold the five students' letter grades, and five arrays of four doubles each to hold each student's set of test scores. The class should have methods that return a specific student's name, the average test score, and a letter grade based on the average. Demonstrate the class in a program that allows the user to enter each student's name and his or her four test scores. It should then display each student's average test score and letter grade. Input Validation: Do not accept test scores less than zero or greater than 100.arrow_forwardWrite the pseudocode that will populate an array of type string with data.The array is called dogBreeds and the following values must be loaded: Labrador, Poodle, Terrier, Spanniel, Dobermanarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
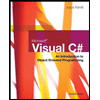
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,