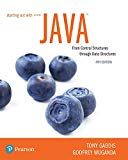
Efficient Computation of Fibonacci Numbers
Modify the application in Code Listing 16-18 by replacing the recursive method for computing Fibonacci terms with an iterative version and try to make it as efficient as possible. Modify the application so it outputs the elapsed time in both seconds and milliseconds. Finally, run the application and generate output showing the rime required to compute the terms of the sequence in positions 45, 46, 47, 48, 49, and 50. Comment on the results.

Want to see the full answer?
Check out a sample textbook solution
Chapter 16 Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Additional Engineering Textbook Solutions
C Programming Language
C How to Program (8th Edition)
Web Development and Design Foundations with HTML5 (8th Edition)
Web Development and Design Foundations with HTML5 (9th Edition) (What's New in Computer Science)
Database Concepts (8th Edition)
- Problem Description Given the following array as an example: int A[10]= {10,3,2,1,6,5,7,8,9,1}; A. Write a recursive function that will print the contents of a given array as shown in the following example Sample output 10 3 2 1 6 5 7 8 9 1 10 3 2 1 6 5 7 8 9 10 3 2 1 6 5 7 8 10 3 2 1 6 5 7 10 3 2 1 6 5 10 3 2 1 6 10 3 2 1 10 3 2 10 3 10 B. Modify the above recursive function so that it will print the contents of a given array as shown in the following example 10 3 2 1 6 5 7 8 9 1 3 2 1 6 5 7 8 9 2 1 6 5 7 8 1 6 5 7 6 5arrow_forwardWrite these in Pseudocode #1a – In pseudocode, write a call to a function that passes 1 Integer variable and 1 Integer array, and saves a Boolean value in return. #1b – In pseudocode, write the function that accepts 1 Integer and 1 Integer array and returns a Boolean. In the function, search the Integer array with a for-loop, and if the Integer parameter is found in the array, return false. If the Integer parameter is not found, return true. #2a – In pseudocode, write a call to a module that passes 1 Integer variable, 1 Real variable, 1 String constant, and 1 String literal as arguments. #2b – In pseudocode, write the module header that accepts 1 Integer, 1 Real, and 2 Strings as parameters. #3 – This pseudocode has multiple problems. Fix the calling statement and the definition below so that the routine accepts 3 grades as parameters and returns the average into a variable.…arrow_forwardCodeW For fun X C Solved https://codeworkou... 臺亂 CodeWorkout X272: Recursion Programming Exercise: Is Reverse For function isReverse, write the two missing base case conditions. Given two strings, this function returns true if the two strings are identical, but are in reverse order. Otherwise it returns false. For example, if the inputs are "tac" and "cat", then the function should return true. Examples: isReverse("tac", "cat") -> true Your Answer: 1 public boolean isReverse(String s1, String s2) { 2. if > 3. 4. else if > return true; return false; 5. 6. else { String s1first = String s2last return s1first.equals (s2last) && 51. substring(0, 1); s2, substring(s2.length() 1); 7. 8. 6. isReverse(s1.substring(1), s2.substring(0, s2.length() 1)); { 12} 1:11AM 50°F Clear 12/4/2021arrow_forward
- PYTHON RECURSIVE FUNCTION Write a python program that lists all ways people can line up for a photo (all permutations of a list of strings). The program will read a list of one word names, then use a recursive method to create and output all possible orderings of those names, one ordering per line. When the input is: Julia Lucas Mia then the output is (must match the below ordering): Julia Lucas Mia Julia Mia Lucas Lucas Julia Mia Lucas Mia Julia Mia Julia Lucas Mia Lucas Juliaarrow_forwardWrite a recursive function that returns the smallest integer in an array. Write a test program that prompts the user to enter a list of five integers and displays the smallest integer.arrow_forwardCreate a class Recursion. It will have two static methods: removeX and countSubstring and write each function recursively. Recursion class removeX The removeX function will take a String as a parameter. It will return a new String that is the same as the original String, but with all “x” characters removed. This method will be case insensitive. countSubstring The countSubstring function will take two strings as parameters and will return an integer that is the count of how many times the substring (the second parameter) appears in the first string without overlapping with itself. This method will be case insensitive. For example: countSubstring(“catwoman loves cats”, “cat”) would return 2 countSubstring(“aaa nice”, “aa”) would return 1 because “aa” only appears once without overlapping itself. Create a Main class to test and run your Recursion class.arrow_forward
- Create a function that returns the nth catalan number. In combinatorial mathematics, the Catalan numbers form a sequence of natural numbers that occur in various counting problems, often involving recursively-defined objects. They are named after the Belgian mathematician Eugène Charles Catalan (1814-1894). For more info, check out the resource tab. Examples getCatalanNumber (0) → 1 getCatalanNumber (6) → 132 getCatalanNumber (8) 1430 Notes Inputs are zero and positive integers.arrow_forwardGiven the sequence, S2 = 1, 2, 4, 5, 7, 8, 10, 11, 13, 14, … Write a RECURSIVE method called “sequence2” that takes a single int parameter (n) and returns the int value of the nth element of the sequence S2. You will need to determine any base cases and a recursive case that describes the listed sequence. Use the following code to test your answers to questions 3 and 4the output should print the two sequences given (S & S2): public class TestSequences { public static void main(String[] args) { for(int i = 0; i < 10; i++) { System.out.print(sequence(i) + " "); // 2, 4, 6, 12, 22, 40, 74, 136, 250, 460 } System.out.println(); for(int i = 0; i < 10; i++) { System.out.print(sequence2(i) + " "); // 1, 2, 4, 5, 7, 8, 10, 11, 13, 14 } } // *** Your method for sequence here *** // *** Your method for sequences2 here *** } // end of TestSequences classarrow_forward4. CodeW. X b For fun X Solved x b Answer x+ Ohttps://codeworko... CodeWorkout X264: Recursion Programming Exercise: Multiply For function multiply,write the missing base case condition and action. This function will multiply two numbers x and y.You can assume that both x and y are positive. Examples: multiply(2, 3) -> 6 Your Answer: 1 public int multiply(int x, int y) { 2. if > { > } else { return multiply(x 1, y) + y; 3. 5. { 7. 1:08 AM 50°F Clear 日arrow_forward
- Using JAVA Recursive Power Method Write a method called powCalthat uses recursion to raise a number to a power. The method should accept two arguments: The first argument is the exponentand the second argument is the number to be raised(example”powCal(10,2)means2^10). Assume that the exponent is anonnegative integer. Demonstrate the method in a program called Recursive (This means that you need to write a program that has at least two methods: mainand powCal. The powCal method is where you implement the requirements above and the main method is where you make a method call to demonstrate how your powCalmethod work).arrow_forwardThe following recursive method get Number Equal searches the array x of 'n integers for occurrences of the integer val. It returns the number of integers in x that are equal to val. For example, if x contains the 9 integers 1, 2, 4, 4, 5, 6, 7, 8, and 9, then getNumberEqual(x, 9, 4) returns the value 2 because 4 occurs twice in x. public static int getNumberEqual(int x[], int n, int val) { if (n< 0) ( return 0; } else { if (x[n-1) == val) { return getNumberEqual(x, n-1, val) +1; } else { return getNumber Equal(x, n-1, val); } // end if ) // end if } // end get Number Equal Demonstrate that this method is recursive by listing the criteria of a recursive solution and stating how the method meets each criterion.arrow_forwardDesign and implement a recursive program to determine and print the Nth line of Pascal's triangle, as shown below. Each interior value is the sum of the two values above it. Hint: Use an array to store the values on each line.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
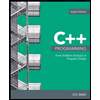