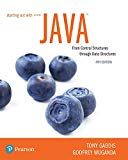
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
4th Edition
ISBN: 9780134787961
Author: Tony Gaddis, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Expert Solution & Answer
Chapter 16, Problem 3SA
Explanation of Solution
Binary search
The binary search algorithm is used to find an item in an array and the search array should be in the sorted order.
- The searching is started in the middle of the array.
- In the searching process, the algorithm finds an item or eliminates half of the array in each comparison.
- If the search item is less than the middle item, then it eliminates the second half of the array, and it continues to search in the first half of the array.
- If the search item is greater than the middle item, then it eliminates the first half of the array, and it continues to search in the second half of the array.
- Once again, it examines the middle and repeats the above steps to find an item.
- If the middle item is equal to search item, then the searching is stopped...
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Find the error, assume data is a string and all variables have been declared.
for ch in data:
if ch.isupper:
num_upper = num_upper + 1
if ch.islower:
num_lower = num_lower + 1
if ch.isdigit:
num_digits = num_digits + 1
if ch.isspace:
num_space = num_space + 1
Find the Error:
date_string = input('Enter a date in the format mm/dd/yyyy: ')
date_list = date_string.split('-')
month_num = int(date_list[0])
day = date_list[1]
year = date_list[2]
month_name = month_list[month_num - 1]
long_date = month_name + ' ' + day + ', ' + year
print(long_date)
Find the Error:
full_name = input ('Enter your full name: ')
name = split(full_name)
for string in name:
print(string[0].upper(), sep='', end='')
print('.', sep=' ', end='')
Chapter 16 Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Ch. 16.1 - Prob. 16.1CPCh. 16.1 - Prob. 16.2CPCh. 16.1 - Prob. 16.3CPCh. 16.1 - Prob. 16.4CPCh. 16.2 - Prob. 16.5CPCh. 16.2 - Prob. 16.6CPCh. 16.2 - Prob. 16.7CPCh. 16.2 - If a sequential search is performed on an array,...Ch. 16.3 - Prob. 16.9CPCh. 16.3 - Prob. 16.10CP
Ch. 16.3 - Prob. 16.11CPCh. 16.3 - Prob. 16.12CPCh. 16.3 - Prob. 16.13CPCh. 16.3 - Prob. 16.14CPCh. 16.3 - Let a[ ] and b[ ] be two integer arrays of size n....Ch. 16.3 - Prob. 16.16CPCh. 16.3 - Prob. 16.17CPCh. 16.3 - Prob. 16.18CPCh. 16 - Prob. 1MCCh. 16 - Prob. 2MCCh. 16 - Prob. 3MCCh. 16 - Prob. 4MCCh. 16 - Prob. 5MCCh. 16 - Prob. 6MCCh. 16 - Prob. 7MCCh. 16 - Prob. 8MCCh. 16 - Prob. 9MCCh. 16 - Prob. 10MCCh. 16 - True or False: If data is sorted in ascending...Ch. 16 - True or False: If data is sorted in descending...Ch. 16 - Prob. 13TFCh. 16 - Prob. 14TFCh. 16 - Assume this code is using the IntBinarySearcher...Ch. 16 - Prob. 1AWCh. 16 - Prob. 1SACh. 16 - Prob. 2SACh. 16 - Prob. 3SACh. 16 - Prob. 4SACh. 16 - Prob. 5SACh. 16 - Prob. 6SACh. 16 - Prob. 7SACh. 16 - Prob. 8SACh. 16 - Prob. 1PCCh. 16 - Sorting Objects with the Quicksort Algorithm The...Ch. 16 - Prob. 3PCCh. 16 - Charge Account Validation Create a class with a...Ch. 16 - Charge Account Validation Modification Modify the...Ch. 16 - Search Benchmarks Write an application that has an...Ch. 16 - Prob. 8PCCh. 16 - Efficient Computation of Fibonacci Numbers Modify...
Knowledge Booster
Similar questions
- Please show the code for the Tikz figure of the complex plane and the curve C. Also, mark all singularities of the integrand.arrow_forward11. Go to the Webinars worksheet. DeShawn wants to determine the number of webinars the company can hold on Tuesdays and Thursdays to make the highest weekly profit without interfering with consultations, which are also scheduled for Tuesdays and Thursdays and use the same resources. Use Solver to find this information as follows: a. Use Total weekly profit as the objective cell in the Solver model, with the goal of determining the maximum value for that cell. b. Use the number of Tuesday and Thursday sessions for the five programs as the changing variable cells. c. Determine and enter the constraints based on the information provided in Table 3. d. Use Simplex LP as the solving method to find a global optimal solution. e. Save the Solver model below the Maximum weekly profit model label. f. Solve the model, keeping the Solver solution. Table 3: Solver Constraints Constraint Cell or Range Each webinar is scheduled at least once on Tuesday and once on Thursday B4:F5 Each Tuesday and…arrow_forwardGo to the Webinars DeShawn wants to determine the number of webinars the company can hold on Tuesdays and Thursdays to make the highest weekly profit without interfering with consultations, which are also scheduled for Tuesdays and Thursdays and use the same resources. Use Solver to find this information as follows: Use Total weekly profit as the objective cell in the Solver model, with the goal of determining the maximum value for that cell. Use the number of Tuesday and Thursday sessions for the five programs as the changing variable cells. Determine and enter the constraints based on the information provided in Table 3. Use Simplex LP as the solving method to find a global optimal solution. Save the Solver model below the Maximum weekly profit model label. Solve the model, keeping the Solver solution. Table 3: Solver Constraints Constraint Cell or Range Each webinar is scheduled at least once on Tuesday and once on Thursday B4:F5 Each Tuesday and Thursday…arrow_forward
- I want to ask someone who has experiences in writing physics based simulation software. For context I am building a game engine, and want to implement physics simulation. There are a few approaches that I managed to find, but would like to know what are other approaches to doing physics simulation entry points from scenes, would you be able to visually draw me a few approaches (like 3 approaces)? When I say entry point to the actual physics simulation. An example of this is when the user presses the play button in the editor, it starts and initiates the physics system. Applying all of the global physics settings parameters that gets applied to that scene. Here is the use-case, I am looking for. If you have two scenes, and select scene 1. You press the play button. The physics simulation starts. When that physics simulation starts, you are also having to update the physics through some physics dedicated delta time because physics needs to happen faster update frequency. To elaborate,…arrow_forwardI want to ask someone who has experiences in writing physics based simulation software. For context I am building a game engine, and want to implement physics simulation. There are a few approaches that I managed to find, but would like to know what are other approaches to doing physics simulation entry points from scenes, would you be able to visually draw me a few approaches (like 3 approaces)?When I say entry point to the actual physics simulation. An example of this is when the user presses the play button in the editor, it starts and initiates the physics system. Applying all of the global physics settings parameters that gets applied to that scene.Here is the use-case, I am looking for. If you have two scenes, and select scene 1. You press the play button. The physics simulation starts. When that physics simulation starts, you are also having to update the physics through some physics dedicated delta time because physics needs to happen faster update frequency.To elaborate, what…arrow_forwardMale comedians were typically the main/dominant star of television sitcoms made during the FCC licensing freeze. Question 19 options: True False In the episode of The Honeymooners that you watched this week, why did Alice decide to get a job outside of the home? Question 1 options: to earn enough money to buy a mink coat to have something to do while the kids were at school to pay the bills after her husband got laid offarrow_forward
- After the FCC licensing freeze was lifted, sitcoms featuring urban settings and working class characters became far less common. Question 14 options: True Falsearrow_forwardsolve this questions for me .arrow_forwarda) first player is the minimizing player. What move should be chosen?b) What nodes would not need to be examined using the alpha-beta pruning procedure?arrow_forward
- Consider the problem of finding a path in the grid shown below from the position S to theposition G. The agent can move on the grid horizontally and vertically, one square at atime (each step has a cost of one). No step may be made into a forbidden crossed area. Inthe case of ties, break it using up, left, right, and down.(a) Draw the search tree in a greedy search. Manhattan distance should be used as theheuristic function. That is, h(n) for any node n is the Manhattan distance from nto G. The Manhattan distance between two points is the distance in the x-directionplus the distance in the y-direction. It corresponds to the distance traveled along citystreets arranged in a grid. For example, the Manhattan distance between G and S is4. What is the path that is found by the greedy search?(b) Draw the search tree in an A∗search. Manhattan distance should be used as thearrow_forwardwhats for dinner? pleasearrow_forwardConsider the follow program that prints a page number on the left or right side of a page. Define and use a new function, isEven, that returns a Boolean to make the condition in the if statement easier to understand. ef main() : page = int(input("Enter page number: ")) if page % 2 == 0 : print(page) else : print("%60d" % page) main()arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
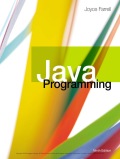
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
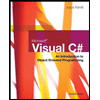
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
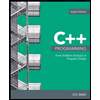
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
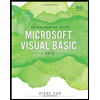
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
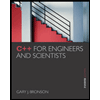
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr