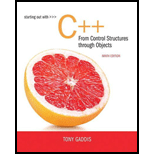
Date Class Modification
Modify the Date class in
++ Prefix and postfix increment operators. These operators should increment the object’s day member.
−− Prefix and postfix decrement operators. These operators should decrement the object’s day member.
− Subtraction operator. If one Date object is subtracted from another, the operator should give the number of days between the two dates. For example, if April 10, 2014 is subtracted from April 18, 2014, the result will be 8.
≪ cout’s stream insertion operator. This operator should cause the date to be displayed in the form April 18, 2018
≫ cin’s stream extraction operator. This operator should prompt the user for a date to be stored in a Date object.
The class should detect the following conditions and handle them accordingly:
• When a date is set to the last day of the month and incremented, it should become the first day of the following month.
• When a date is set to December 31 and incremented, it should become January 1 of the following year.
• When a day is set to the first day of the month and decremented, it should become the last day of the previous month.
• When a date is set to January 1 and decremented, it should become December 31 of the previous year.
Demonstrate the class’s capabilities in a simple program.
Input Validation: The overloaded ≫ operator should not accept invalid dates. For example, the date 13/45/2018 should not he accepted.

Want to see the full answer?
Check out a sample textbook solution
Chapter 14 Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Additional Engineering Textbook Solutions
Web Development and Design Foundations with HTML5 (8th Edition)
Concepts of Programming Languages (11th Edition)
Digital Fundamentals (11th Edition)
Web Development and Design Foundations with HTML5 (9th Edition) (What's New in Computer Science)
Objects First with Java: A Practical Introduction Using BlueJ (6th Edition)
Computer Systems: A Programmer's Perspective (3rd Edition)
- Double Bubble For this exercise you need to create a Bubble class and construct two instances of the Bubble object. You will then take the two Bubble objects and combine them to create a new, larger combined Bubble object. This will be done using functions that take in these Bubble objects as parameters. The Bubble class contains one data member, radius_, and the corresponding accessor and mutator methods for radius_, GetRadius and SetRadius. Create a member function called CalculateVolume that computes for the volume of a bubble (sphere). Use the value 3.1415 for PI. Your main function has some skeleton code that asks the user for the radius of two bubbles. You will use this to create the two Bubble objects. You will create a CombineBubbles function that receives two references (two Bubble objects) and returns a Bubble object. Combining bubbles simply means creating a new Bubble object whose radius is the sum of the two Bubble objects' radii. Take note that the CombineBubbles function…arrow_forwardDynamic Games Instructions: Write a class called Game that contains a video game’s name, genre, and difficultyLevel. Include a default constructor and destructor for the class. The constructor should print out the following message: “Creating a new game”. The destructor should print out the following message: “In the Game destructor.” Include appropriate get/set functions for the class. In main(), prompt the user to enter the number of games he or she has played in the past year. Dynamically create a built-in array based on this number (not a vector or object of the array class) to hold pointers to Game objects. Construct a loop in main() that executes once for each of the number of games that the user indicated. Within this loop, ask the user to enter the name and genre of each game. Using a random number generator, generate a difficultyLevel between 1-10 (inclusive). Seed this random number generator with 100. Next, dynamically create a Game object (remember that this…arrow_forwardasaparrow_forward
- Problem: Employee and ProductionWorker Classes Write a python class named ProductionWorker that is a subclass of the Employee class. The ProductionWorker class should keep data attributes for the following information: • Shift number (an integer, such as 1, 2, or 3)• Hourly pay rateThe workday is divided into two shifts: day and night. The shift attribute will hold an integer value representing the shift that the employee works. The day shift is shift 1 and the night shift is shift 2. Write the appropriate accessor and mutator methods for this class. Once you have written the class, write a program that creates an object of the ProductionWorker class, and prompts the user to enter data for each of the object’s data attributes. Store the data in the object, then use the object’s accessor methods to retrieve it and display it on the screen Note: The program should be written in python. Sample Input/Output: Enter the name: Ahmed Al-AliEnter the ID number: 12345Enter the department:…arrow_forwardTrue or False: 1. Data items in a class may be public. 2. Class members are public by default. 3. Friend functions have access only to public members of the class.arrow_forwardRetailItem Class Write a class named RetailItem that holds data about an item in a retail store. The class should have the following fields: description. The description field references a string object that holds a brief description of the item. • unitsonHand. The unitsOnHand field is an int variable that holds che number of units currently in inventory. price. The price field is a double that holds the item's retail price. Write a constructor that accepts arguments for each field, appropriate mutator methods that store values in these fields, and accessor methods that return the values in these fields. Once you have written the class, write a separate program that creates three RetaillItem objects and stores the following data in them: Description Units on Hand Price Item #1 Jacket 12 59.95 Item #2 Designer Jeans 40 34.95 Irem #3 Shirt 20 24.95arrow_forward
- True/False: a member function in a class can access all of its class's member variables, but not if the variables are protected.arrow_forwardEmployee Class (Medium) Write a class named Employee that has the following member variables: name. A string that holds the employee’s name. idNumber. An int variable that holds the employee’s ID number. department. A string that holds the name of the department where the employee works. position. A string that holds the employee’s job title. The class should have the following constructors: A constructor that accepts the following values as arguments and assigns them to the appropriate member variables: employee’s name, employee’s ID number, department, and position. A constructor that accepts the following values as arguments and assigns them to the appropriate member variables: employee’s name and ID number. The department and position fields should be assigned an empty string (""). A default constructor that assigns empty strings ("") to the name, department, and position member variables, and 0 to the idNumber member variable. Write appropriate mutator functions that…arrow_forwardThe enum class construct: Select one: a. does not require one to write anything in front of an enumeration value-name b. requires one to use the name of the enumeration, followed by "::" (colon-colon), before each name whenever the enumeration's value-name is used c. requires one to use the name of the enumeration, followed by "." (period), before each name whenever an enumeration's value-name is usedarrow_forward
- 6. Patient Charges Write a class named Patient that has attributes for the following data: • First name, middle name, and last name • Address, city, state, and ZIP code • Phone number ● The Patient class's init__ method should accept an argument for each attribute. The Name and phone number of emergency contact Patient class should also have accessor and mutator methods for each attribute. Next, write a class named Procedure that represents a medical procedure that has been performed on a patient. The Procedure class should have attributes for the following data: • Name of the procedure • Date of the procedure • Name of the practitioner who performed the procedure • Charges for the procedure 107 The Procedure class's __init__ method should accept an argument for each attribute. The Procedure class should also have accessor and mutator methods for each attribute Next, write a program that creates an instance of the Patient class, initialized with sample data. Then, create three…arrow_forwardMonth Class Exceptions Write a class named Month. The class should have an int field named monthNumber that holds the number of the month. For example, Janauary would be 1, February would be 2, and so forth. In addition, provide the following methods: A no-arg constructor that sets the monthNumber field to 1. A constructor that accepts the number of the month as an argument. It should set the monthNumber value to the value passed as the argument. If a value less than 1 or greater than 12 is passed, the constructor should throw an InvalidMonthNumberException. A constructor that accepts the name of the month, such as “January” or “February”, as an argument. It should set the monthNumber field to the correct corresponding value. If an invalid name is passed, the constructor should throw an InvalidMonthNameException. A setMonthNumber method that accepts an int argument, which is assigned to the monthNumber field. If a value less than 1 or greater than 12 is passed, throw an…arrow_forwardC++ oop using classes only You are going to make an employee management system. In this lab, you have to create anEmployee class only. Employee class fields are: Employee Name, Employee ID, andEmployee Gender. The member functions include the getters and setters for all the membervariables and display () function to display the data. In the main function, first create twoEmployee objects and allow the user to enter data in these objects. Secondly, display thedata from these objects.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
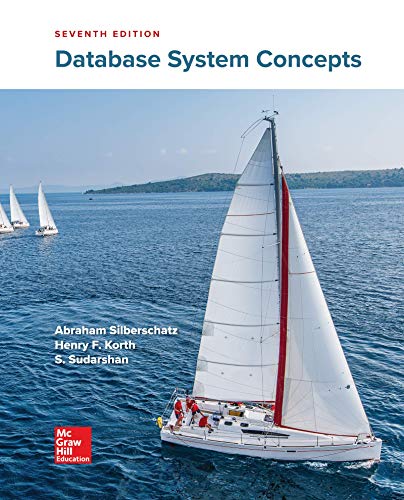
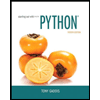
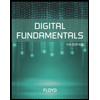
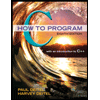
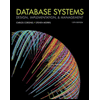
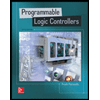