Your task is to implement a variation of the classic word game Hangman, which involves players guessing the letters in a word chosen at random with a finite number of guesses. While there are alternate versions such as category Hangman and Wheel of Fortune, which involve players guessing idioms, places, names and so on, we will be sticking with the traditional version. Requirements: You will implement a function called main that allows users to play an interactive hangman game against the computer. The computer should pick a word, and players should then try to guess letters in the word until they win or run out of guesses. Here is the overarching behaviour we expect: 1. The program should load a list of available words from the text file provided. Note that the file you have been given contains words in lowercase. 2. The computer should then select a word at random from the list at random. 3. The user is given a certain number of guesses at the beginning. 4. The game is interactive; the user inputs their guess and the computer either: a. reveals the letter if it exists in the secret word b. penalizes the user and updates the number of guesses remaining 5. The game ends when the user guesses the word, or runs out of available guesses. You are going to make use of a common approach to computational problem solving, which involves breaking the problem down into several logical subtasks to make things more manageable. The file hangman.py contains several existing helper variables and functions which you should use to help you complete the task. For this challenge, you will extend the existing program so that players scores are saved to and read from a text file e.g. scores.txt. You should ask for the player’s name at the start of each new game and use it to load their previous high score. If they manage to beat their personal best while playing, ask them if they would like to update the leaderboard and do so if prompted. To do this, you should create two new functions: • A function to retrieve the existing high score for the current player called: get_score. This should take a single argument, the players name and return their high score. If they don’t have a high score return 0 or None. Players without a previous high score should always be asked whether they would like to store their score. • A function to save a new high score for the current player called: save_score. This should take two arguments (the players name and score). The function does not need to have a return value, but should handle writing the latest score to the text file. If a player already has a lower score saved in the file, update it instead. Your game will also need a more robust menu, it should contain options to play the game, load the leaderboard or quit. Players should return here after playing or viewing the leaderboard. Selecting the option to quit should close the program after printing a goodbye message. The logic for this should be contained in your main function, update it as required. format : import random import string WORDLIST_FILENAME = "words.txt" # Responses to in-game events # Use the format function to fill in the spaces responses = [ "I am thinking of a word that is {0} letters long", "Congratulations, you won!", "Your total score for this game is: {0}", "Sorry, you ran out of guesses. The word was: {0}", "You have {0} guesses left.", "Available letters: {0}", "Good guess: {0}", "Oops! That letter is not in my word: {0}", "Oops! You've already guessed that letter: {0}", ] def choose_random_word(all_words): return random.choice(all_words) # end of helper code # ----------------------------------- def load_words(): # TODO: Fill in your code here pass # Load the list of words into the variable wordlist # Accessible from anywhere in the program # TODO: uncomment the below line once # you have implemented the load_words() function # wordlist = load_words() def is_word_guessed(word, letters_guessed): # TODO: Fill in your the code here pass def get_guessed_word(word, letters_guessed): # TODO: Fill in your code here pass def get_remaining_letters(letters_guessed): # TODO: Fill in your code here pass def hangman(word): print("Welcome to Hangman Ultimate Edition") print("I am thinking of a word that is {0} letters long".format(len(word))) print("-------------") # TODO: Fill in your code here # ---------- Challenge Functions (Optional) ---------- def get_score(name): pass def save_score(name, score): pass # When you've completed your hangman function, scroll down to the bottom # of the file and uncomment the last lines to test # (hint: you might want to pick your own # word while you're doing your own t
Your task is to implement a variation of the classic word game Hangman, which involves players
guessing the letters in a word chosen at random with a finite number of guesses. While there are
alternate versions such as category Hangman and Wheel of Fortune, which involve players
guessing idioms, places, names and so on, we will be sticking with the traditional version.
Requirements:
You will implement a function called main that allows users to play an interactive hangman game
against the computer. The computer should pick a word, and players should then try to guess letters
in the word until they win or run out of guesses.
Here is the overarching behaviour we expect:
1. The program should load a list of available words from the text file provided. Note that
the file you have been given contains words in lowercase.
2. The computer should then select a word at random from the list at random.
3. The user is given a certain number of guesses at the beginning.
4. The game is interactive; the user inputs their guess and the computer either:
a. reveals the letter if it exists in the secret word
b. penalizes the user and updates the number of guesses remaining
5. The game ends when the user guesses the word, or runs out of available guesses.
You are going to make use of a common approach to computational problem solving, which
involves breaking the problem down into several logical subtasks to make things more
manageable. The file hangman.py contains several existing helper variables and functions
which you should use to help you complete the task.
For this challenge, you will extend the existing program so that players scores are saved to and
read from a text file e.g. scores.txt. You should ask for the player’s name at the start of each new
game and use it to load their previous high score. If they manage to beat their personal best while
playing, ask them if they would like to update the leaderboard and do so if prompted.
To do this, you should create two new functions:
• A function to retrieve the existing high score for the current player called: get_score.
This should take a single argument, the players name and return their high score. If they
don’t have a high score return 0 or None. Players without a previous high score should
always be asked whether they would like to store their score.
• A function to save a new high score for the current player called: save_score. This
should take two arguments (the players name and score). The function does not need to
have a return value, but should handle writing the latest score to the text file. If a player
already has a lower score saved in the file, update it instead.
Your game will also need a more robust menu, it should contain options to play the game, load the
leaderboard or quit. Players should return here after playing or viewing the leaderboard. Selecting
the option to quit should close the program after printing a goodbye message. The logic for this
should be contained in your main function, update it as required.
format :
import string
WORDLIST_FILENAME = "words.txt"
# Responses to in-game events
# Use the format function to fill in the spaces
responses = [
"I am thinking of a word that is {0} letters long",
"Congratulations, you won!",
"Your total score for this game is: {0}",
"Sorry, you ran out of guesses. The word was: {0}",
"You have {0} guesses left.",
"Available letters: {0}",
"Good guess: {0}",
"Oops! That letter is not in my word: {0}",
"Oops! You've already guessed that letter: {0}",
]
def choose_random_word(all_words):
return random.choice(all_words)
# end of helper code
# -----------------------------------
def load_words():
# TODO: Fill in your code here
pass
# Load the list of words into the variable wordlist
# Accessible from anywhere in the program
# TODO: uncomment the below line once
# you have implemented the load_words() function
# wordlist = load_words()
def is_word_guessed(word, letters_guessed):
# TODO: Fill in your the code here
pass
def get_guessed_word(word, letters_guessed):
# TODO: Fill in your code here
pass
# TODO: Fill in your code here
pass
def hangman(word):
print("Welcome to Hangman Ultimate Edition")
print("I am thinking of a word that is {0} letters long".format(len(word)))
print("-------------")
# TODO: Fill in your code here
# ---------- Challenge Functions (Optional) ----------
def get_score(name):
pass
def save_score(name, score):
pass
# When you've completed your hangman function, scroll down to the bottom
# of the file and uncomment the last lines to test
# (hint: you might want to pick your own
# word while you're doing your own testing)
# -----------------------------------
def main():
# Uncomment the line below once you have finished testing.
# word = choose_random_word(wordlist)
# Uncomment the line below once you have implemented the hangman function.
# hangman(word)
pass
# Driver function for the program
if __name__ == "__main__":
main()


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

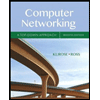
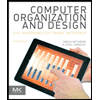
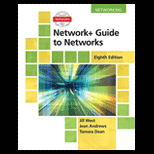
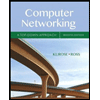
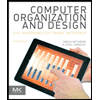
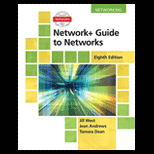
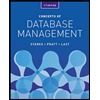
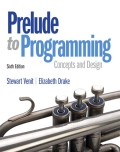
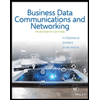