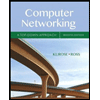
Java programming
please type the code
For this problem set, you will submit a single java file named Homework.java. You are supposed to add the functions described below to your Homework class. The functional signatures of the functions you create must exactly match the signatures in the problem titles. You are not allowed to use any 3rd party libraries in the homework assignment nor are you allowed to use any dependencies from the java.util package besides the Pattern Class. When you hava completed your homework, upload the Homework.java file to Grader Than. All tests will timeout and fail if your code takes longer than 10 seconds to complete.
ProtectedFunction(string, int)
This is a protected non-static function that takes a String and an int as its arguments and returns nothing.
You don't need to put any code in this function, you may leave the function's code empty. Don't overthink this problem. This is to test your knowledge on how to create a non-static protected function.
Arguments:
String text - An string
String int - An integer

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- In this project you will implement a student database using JavaFX. A GUI should be used as the program's interface. This program must consist of two classes. (1) The first class should define the GUI and handle the database interactions: a. Combo box should allow the user to select one of the four database actions (Insert, Delete, Find, and Update). b. The database should be implemented as a HashMap, with the ID field as the key and a student record consisting of a name and major as the value. c. The operation should be performed when the user clicks the Process Request button. d. If the user attempts to insert a key that is already in the database an error message should be displayed using a JOptionPane message dialog box. e. If the user attempts to delete, find or update a record that is not in the database, a message should also be displayed. f. After each successful operation is completed a JOptionPane window should be…arrow_forwardGDB and Getopt Class Activity activity [-r] -b bval value Required Modify the activity program from last week with the usage shown. The value for bval is required to be an integer as is the value at the end. In addition, there should only be one value. Since everything on the command line is read in as a string, these now need to be converted to numbers. You can use the function atoi() to do that conversion. You can do the conversion in the switch or you can do it at the end of the program. The number coming in as bval and the value should be added together to get a total. That should be the only value printed out at the end. Total = x should be the only output from the program upon success. Remove all the other print statements after testing is complete. Take a screenshot of the output to paste into a Word document and submit. Practice Compile the program with the -g option to load the symbol table. Run the program using gdb and use watch on the result so the program stops when the…arrow_forwardI have a text file that have a list of employee's Id and name correspond to it. All the IDs are unique. What I want to do is I want to read from the text file and then sort the Id in order then put it back into the text file. How can I do this in java? Can you write a code for it in java. For example: the text file text.txt contains Id Name 3 Kark 1 Mia 5 Tyrus 2 John After sort, the text.txt will be 1 Mia 2 John 3 Karl 5 Tyrusarrow_forward
- JAVA Problem – CycleFileOutput Revisit the Cycle class. Modify your application such that the properties will be written to a text file called “Cycle.txt” instead of to the screen. Directions Examine your application for the class called Cycle. Add an appropriate throws statement in the main method. Create a reference to a File class with the appropriate name of a text file (Cycle.txt). Use appropriate code to ensure that the text file exist. Output the values of the variables to the text file. Close the file. Note: Verify the contents were written to the text file using notepad (or any word processor). public class Cycle { // Declear integer instance variable private int numberOfWheels; private int weight; // Constructer declear class public Cycle(int numberOfWheels, int weight ) { this.numberOfWheels = numberOfWheels; this.weight = weight; } // String method for output public String toString() { String wheel = String.valueOf(this.numberOfWheels); String load =…arrow_forwardYou are the owner of a Bagel Shop. You want to display your menu that has 3 categories, Bagel Type, Bagel Toppings, and Coffee. Using Java create 1 new Java class containing 3 separate methods, each method responsible for writing/creating the data file. The end result should be 3 TXT files, each containing the product names and prices for each category. Modify each JPanel class constructor to call a separate method to read this input file and display the product names and the prices by adding them to JRadioButton, JLabel and/or JTextBox objects.arrow_forwardThe code given below represents a saveTransaction() method which is used to save data to a database from the Java program. Given the classes in the image as well as an image of the screen which will call the function, modify the given code so that it loops through the items again, this time as it loops through you are to insert the data into the salesdetails table, note that the SalesNumber from the AUTO-INCREMENT field from above is to be inserted here with each record being placed into the salesdetails table. Finally, as you loop through the items the product table must be update because as products are sold the onhand field in the products table must be updated. When multiple tables are to be updated with related data, you should wrap it into a DMBS transaction. The schema for the database is also depicted. public class PosDAO { private Connection connection; public PosDAO(Connection connection) { this.connection = connection; } public void…arrow_forward
- In this task, you are required to write a Java program in NetBeans that implements Parallel programming using the Fork/Join framework. Your program should initially generate 15 million random numbers. This should be read into an array of doubles. Then it should make use of a method that uses this array to calculate the sum of these doubles. Your program should make use of the subclasses in the Fork/Join Framework. Your program should also output the number of processors available.arrow_forwardYou have been hired at an open-air mine. You are to write a program to control a digger. For your task, you have been given a `map' of all the underground resources in the mine. This map comes as a file. The file has n rows. Each row has n space-separated integers. Each integer is between zero and one hundred (inclusive). The file should be understood to represent a grid, n wide by n deep. Each square in the grid can contain valuable resources. The value of these resources can go from zero (no resources) to 100 (max resources). The grid maps the resources just below the surface, and down to the lowest diggable depths. The digger starts at the surface (so, just on top of the topmost row in the grid)—at any horizontal position between 1 and n. The digger cannot dig directly downwards, but it can dig diagonally in either direction, left-down, or right-down. In its first time-step, it will dig onto the first row of the grid. In its second time-step, it'll hit the second row, and so on.…arrow_forward5. Complete the class code below and include a method named partition which takes an integer array and a partition integer as parameters and then partitions the original array into two new arrays where elements of the first array are all less than or equal to the partition integer and the second array are all greater than the partition integer. The method should print the original array, followed by the lower partition and finally the upper partition. Nothing should be returned by the method. For example, given int[ ] arr = {1, 45, 16, 7, 39, 6, 5, 11, 72, 31, 15} a method call to partition(arr, 11) should output: %3D [1, 45, 16, 7, 39, 6, 5, 11, 72, 31, 15] [1, 5, 6, 7, 11] [15, 16, 31, 39, 45, 72] public class Partition { public static void main(String[] args) { int[] arrayToPartition = {1, 45, 16, 7, 39, 6, 5, 11, 72, 31, 15}; %3D int partitionNumber = 11; partition(arrayToPartition, partitionNumber); } /l your method code here } // end of Partition classarrow_forward
- what are the proper steps for this Create a client class named Client with the main( ) method. Inside the main method do the following: Create an instance of BiGBirdcalled Little Squadto store players’ information of U.S Swimm team team using the overload constructor to make the initial length of the list array equal to 2. Add eight players to Little Squad by hardcoding eight calls to the add method (one for each player). Display the contents of Little Squad Remove a random player from Little Squad Display the contents of Little Squad. Get but do not remove a reference to the 3rditem in the bag. Display the contents of the reference you “got” it step 5. Add another Player with another hardcoded add method call. Display the contents of Little Squad. Remove the Player that you “got” in step 5 using a call to the remove(E e)method. Display the contents of Little Squad.arrow_forwardWhere can you find MIN_VALUE and MAX_VALUE constants? Java doesn't have constants In StringTokenizer class In primitive data types In wrapper classesarrow_forwardPlease read the instructions carefully and keep in mind of the bolded phrases. You are NOT ALLOWED to use HashSet Create a project in NetBeans and name the project Hw06. The class will contain the following static methods: reverseS – A method that displays a string reversely on the console using the following signature: publicstaticvoidreverseS(Strings) printSub1 – print all substrings of a string (duplicated substrings are allowed, but loops are not allowed). The method signature: public static void printSub1(String s) printSub2 – print all substrings of a string (duplicated substrings are not allowed, but loops are allowed). The method signature: public static void printSub2(String s) Note: All methods should be RECURSIVE. Any predefined classes that are based on Set are not allowed. In the main method, read a string from the user and output the reversed string and substrings to the screen: Sample Run: Please input a string: abcdThe reversed string: dcbaThe substrings…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
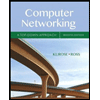
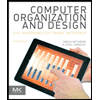
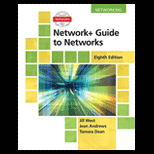
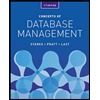
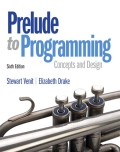
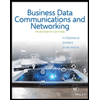