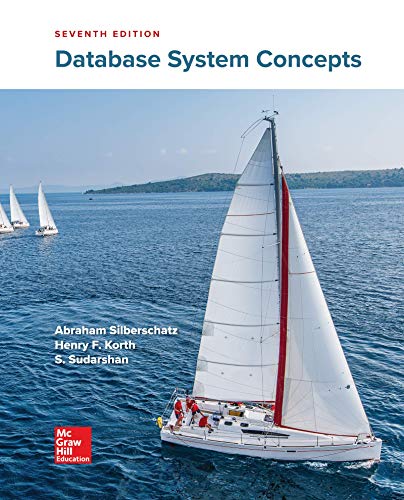
I am looking for the following in your
Python GUI-based MP3 Player
Project #3: Python GUI-based MP3 Player
1. Ability to select a folder / directory for MP3s. (HINT: There’s a function/method in filedialog of tkinter to do this. In other words, you'll need the following import:
from tkinter import messagebox, filedialog
2. Ability to play selected MP3s.
3. Ability to pause the current MP3.
4. Quit button/function to exit application.
5. Use a list to store and display the mp3 file names. You will need to demonstrate your mastery of lists, loops, and GUI elements within this program.
Submission will include:
1. Python program named myplayer.py
2. Data Flow Diagrams / Pseudocode
3. If choosing an alternate library to tkinter or pygame, provide instructions and download links for the alternate library.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- in java but don't write as a GUI (Graphical User Interface) Write a program that reads a file named input.txt and writes a file that contains the same contents, but is named output.txt. The input file will contain more than one line when I test this and so should your output file. Do not use a path name when opening these files. This means the files should be located in the top level folder of the project. This would be the folder that contains the src folder, probably named FileCopy depending on what name you gave the project. Do not use a copy method that is supplied by Java. Your program must read the file line by line and write the file itself. Class should be named FileCopy do not write to input.txt!arrow_forwardImplement a graphical application that displays a list of courses. Briefly, this GUI will take the inputs (i.e., course information), store them in an array list, and display course information in the output area. Figure 1 shows the layout ofthe CourseDisplay GUI. You should have three Java files: 1. CourseDisplayFrame class that extendsJFrame class (The main part of your program). 2.CourseDisplayViewer class, which contains the main method where a CourseDisplay object will be created, and the GUI will pop up (Suggested frame width of 450, and frame height of 400). 3.Course class, which is the class that models the Course objects (This file will be given to you). In your CourseDisplayFrame class file, you should have the followings: •Input area: –A text label and a text field (suggested width of 30) for course code; –A text label and a text field (suggested width of 30) for course name; –A text label and a text field (suggested width of 30) for course credit; –A text label and a text…arrow_forwardIn java plsarrow_forward
- Need guidance on how to use Tkiner in visual studio. The tkiner program doesn't run, it shows as a syntax error. Code: from tkinter import* import tkinter.messagebox class Library: def __init_(self,root): self.root = root self.root.title("Library Inventory System") self.root.geometry("1350x750+0+0") BookId = StringVar() AuthorFullName=StringVar() PublicationYear=StringVar() BookTitle=StringVar() num_copies=StringVar() #===================Frames==========================# MainFrame = Frame(self.root) MainFrame.grid() TitleFrame = Frame(MainFrame, bd=2, padx=40, pady=8, bg="Cadet blue", relief=RIDGE) TitleFrame.pack(side=TOP) self.libTitle =Label(TitleFrame, font=('arial',46,'bold'),text="Library Inventory System") self.libTitle.grid(sticky=W) ButtonFrame…arrow_forwardJAVA PPROGRAM Please Modify this program with further modifications as listed below: ALSO, take out and change the following in the program: System.out.println("Program terminated."); because the test case does not need it And change this in the program: Please enter the file name or type QUIT to exit:\n so it reperats once for every test case because it repeats twice in every case as shown in the screenshot. I only need it once. I have provided the failed the test cases and the inputs as a screenshot. The program must pass the test case when uploaded to Hypergrade. import java.io.*;import java.util.ArrayList;import java.util.Scanner;public class SymmetricalNameMatcher { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); String fileName; do { // Prompt the user to enter a file name or 'QUIT' to exit. System.out.print("Please enter the file name or type QUIT to exit:\n"); fileName =…arrow_forwardFor the following program in phyton : Pay CheckWindow CostWrite an interactive program to calculate the cost of glasswork and metalstripping for rectangular windows. All windows, no matter what size, use thesame quality of glass and aluminum strips. Prompt the user for his/her name,age, length, and width of a window, then calculate the costs shown based on theinformation read in from the windowinfo.txt file which contains the materialstypes and costs (you cannot change this file at all). There is a discount on thetotal cost of 10% if the total area is over 1000 square inches, and 15% if it isover 1500 square inches.*Be sure to include a try/except and any and all string methods you might need*No conditional loops can be used at all**This is the info you will see in the text file, remember you mustread this in and store it:Glass: 80 cents per square inch (unit area)Aluminum: $1.25 per inch (unit length) This is the sample output. Write it to both the screen and a text file…arrow_forward
- Can someone please help me with this project for my Java Programming class? If you could correct my mistakes for me that would be great.arrow_forwardIn java, without Arrays and using only String methods ( don't use String builder) // Question 17: In MS-DOS, a file name consists of up // to 8 characters (excluding '.', ':', backslask, '?', // and '*'), followed by an optional dot ('.' character) // and extension. The extension may contain zero to three // characters. For example, 1STFILE.TXT is a valid filename. // Filenames are case-blind. // // Write and test a method that takes // in a String, validates it as a valid MS-DOS file // name, appends the extensions ".TXT" if no extension // is given (that is, no '.' appears in FILENAME), converts // the name to uppercase, and returns the resulting string // to the calling method // If fileName ends with a dot, remove that dot and do not // append the default extension. If the name is invalid, // validFileName should return null. public String validFileName(String n) { }arrow_forwardHere are what to display on your Pokémon's show page: The pokemon's name The image of the pokemon An unordered list of the Pokemon's types (eg. water, poison, etc). The pokemon's stats for HP, ATTACK, and DEFENSE. module.exports = [ { id: "001", name: "Bulbasaur", img: "http://img.pokemondb.net/artwork/bulbasaur.jpg", type: [ "Grass", "Poison" ], stats: { hp: "45", attack: "49", defense: "49", spattack: "65", spdefense: "65", speed: "45" }, ]; Routes Your app should use RESTful routes: Index GET /pokemon Show GET /pokemon/:id New GET /pokemon/new Edit GET /pokemon/:id/edit Create POST /pokemon Update PUT /pokemon/:id Destroy DELETE /pokemon/:idarrow_forward
- In addition to the instructions on the screenshot, please ensure that your program is made in C++ with comments. Please also ensure that screenshots are taken for the source code and the executed version, including all possible cases. Finally, add your code in a manner so I can just copy and paste it for review. Thanks.arrow_forwardmy assignment wants me to Convert the MileConversions program to an interactive application. Instead of assigning a value to the miles variable, accept it from the user as input. Something is wrong with my code My code is : { public static void main(String[] args) { Scanner inputDevice = new Scanner(System.in); final double INCHES_IN_MILE = 63360; final double FEET_IN_MILE = 5280; final double YARDS_IN_MILE = 1760; double miles = 4; double in, ft, yds; in = miles * INCHES_IN_MILE; ft = miles * FEET_IN_MILE; yds = miles * YARDS_IN_MILE; System.out.println(miles + " miles is " + in + " inches, or " + ft + " feet, or " + yds + " yards"); } }arrow_forwardPlease help me with this questionarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
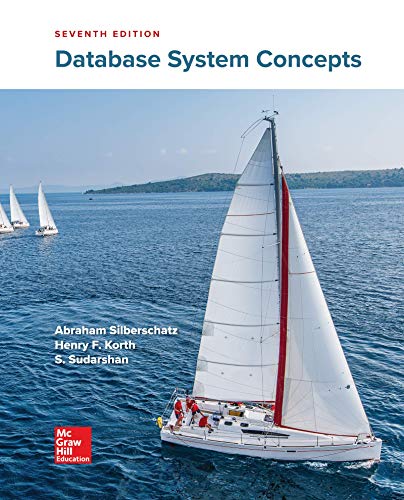
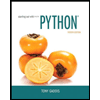
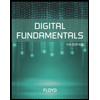
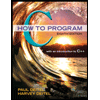
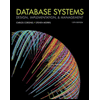
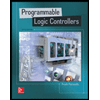