data member numCalories with a value read from input. Then, assign Ham and Cheese's data member numCalo
Assign Barbecue's data member numCalories with a value read from input. Then, assign Ham and Cheese's data member numCalories with another value read from input. Input will contain two integer numbers.
#include <stdio.h>
#include <string.h>
typedef struct Pizza_struct {
char pizzaName[75];
int numCalories;
} Pizza;
int main(void) {
Pizza pizzasList[2];
strcpy(pizzasList[0].pizzaName, "Barbecue");
strcpy(pizzasList[1].pizzaName, "Ham and Cheese");
/* Your code goes here */
printf("A %s slice contains %d calories.\n", pizzasList[0].pizzaName, pizzasList[0].numCalories);
printf("A %s slice contains %d calories.\n", pizzasList[1].pizzaName, pizzasList[1].numCalories);
return 0;
}
please USE C++
I tried 10 time but I cant solve it

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

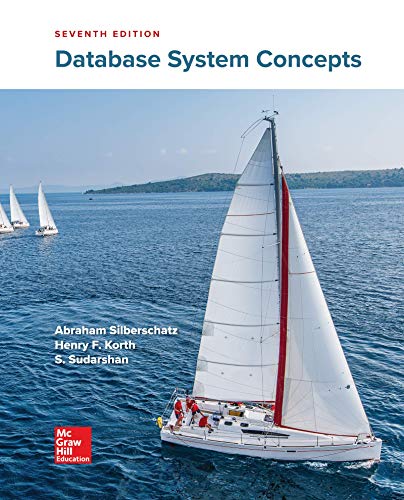
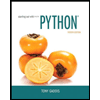
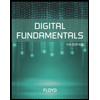
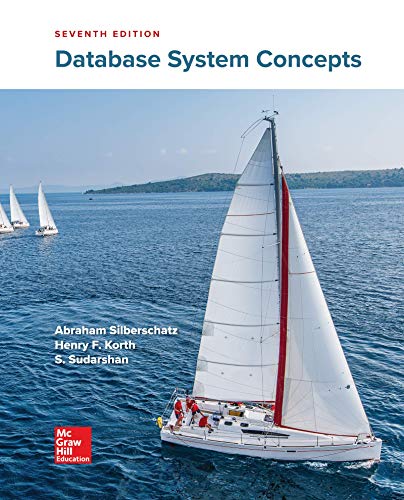
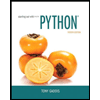
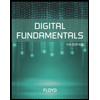
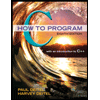
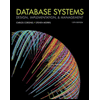
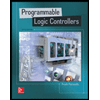