Use Python for this question: Develop a class Volume that stores the volume for a stereo that has a value between 0 and 11. Usage of the class is listed below the problem descriptions. Throughout the class you must guarantee that: The numeric value of the Volume is set to a number between 0 and 11. Any attempt to set the value to greater than 11 will set it to 11 instead, any attempt to set a negative value will instead set it to 0. This applies to the following methods below: __init__, set, up, down
Use Python for this question: Develop a class Volume that stores the volume for a stereo that has a value between 0 and 11. Usage of the class is listed below the problem descriptions. Throughout the class you must guarantee that: The numeric value of the Volume is set to a number between 0 and 11. Any attempt to set the value to greater than 11 will set it to 11 instead, any attempt to set a negative value will instead set it to 0. This applies to the following methods below: __init__, set, up, down
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Use Python for this question:
- Develop a class Volume that stores the volume for a stereo that has a value between 0 and 11. Usage of the class is listed below the problem descriptions. Throughout the class you must guarantee that:
- The numeric value of the Volume is set to a number between 0 and 11. Any attempt to set the value to greater than 11 will set it to 11 instead, any attempt to set a negative value will instead set it to 0.
- This applies to the following methods below: __init__, set, up, down
You must write the following methods:
- __init__ - constructor. Construct a Volume that is set to a given numeric value, or, if no number given, defaults the value to 0. (Subject to 0 <= vol <=11 constraint above.)
- __repr__ - converts Volume to a str for display, see runs below.
- set – sets the volume to the specified argument (Subject to 0 <= vol <=11 constraint above.)
- get – returns the numeric value of the Volume
- up – given a numeric amount, increases the Volume by that amount. (Subject to 0 <= vol <=11 constraint above.)
- down – given a numeric amount, decreases the Volume by that amount. (Subject to 0 <= vol <=11 constraint above.)
- __eq__ - implements the operator ==. Returns True if the two Volumes have the same value and False
Output is in the 2 attached images for this question:
Please run a doctest so the function returns the following output, which is attached in an image

Transcribed Image Text:# default arguments for init
-
-
>>> v = volume () # Volume defaults to 0
>>> v
Volume (0)
# can compare Volumes using
==
>>> # comparisons
>>> v = Volume (5)
>>> v.up (1.1)
>>> v == Volume (6.1)
True
>>> Volume (3.1)
== Volume (3.2)
False
# constructor cannot set the Volume greater
# than 11 or less than 0
>>> v = volume (20)
>>> v
Volume (11)
>>> v = Volume (-1)
>>> v
Volume (0)
>>>

Transcribed Image Text:##### Volume #####
# set and get
>>> v = volume ()
>>> v.set (5.3)
>>> v
Volume (5.3)
>>> v.get ()
5.3
>>> v.get () ==5.3 # return not print
True
>>>
init_,
_repr_, up, down
>>> v = volume (4.5) # set Volume with value
>>> v
Volume (4.5)
>>> v.up (1.4)
>>> v
Volume (5.9)
>>> v.up (6) # should max out at 11
>>> v
Volume (11)
>>> v.down (3.5)
>>> v
Volume (7.5)
>>> v.down (10) # minimum must be 0
>>> v
Volume (0)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
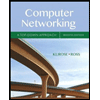
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
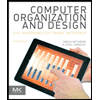
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
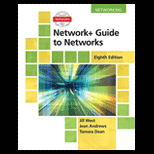
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
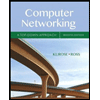
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
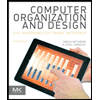
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
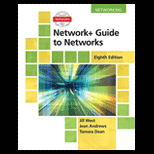
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
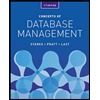
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
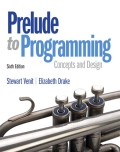
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
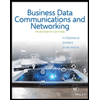
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY