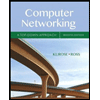
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
solve in python
Street Class
Step 1:
• Create a class to store information about a street:
• Instance variables should include:
• the street name
• the length of the street (in km)
• the number of cars that travel the street per day
• the condition of the street (“poor”, “fair”, or “good”).
• Write a constructor (__init__), that takes four arguments corresponding to
the instance variables and creates one Street instance.
Step 1:
• Create a class to store information about a street:
• Instance variables should include:
• the street name
• the length of the street (in km)
• the number of cars that travel the street per day
• the condition of the street (“poor”, “fair”, or “good”).
• Write a constructor (__init__), that takes four arguments corresponding to
the instance variables and creates one Street instance.
Street Class
Step 2:
• Add additional methods:
• __str__
• Should return a string with the Street information neatly-formatted, as in:
Elm is 4.10 km long, sees 3000 cars per day, and is in poor condition.
• compare:
• This method should compare one Street to another, with the Street the method is called
on being compared to a second Street passed to a parameter.
• Return True or False, indicating whether the first street needs repairs more urgently than
the second.
• Streets in “poor” condition need repairs more urgently than streets in “fair” condition, which
need repairs more urgently than streets in “good” condition.
• If the condition of two Streets is the same, the one with more traffic is given higher priority.
Step 2:
• Add additional methods:
• __str__
• Should return a string with the Street information neatly-formatted, as in:
Elm is 4.10 km long, sees 3000 cars per day, and is in poor condition.
• compare:
• This method should compare one Street to another, with the Street the method is called
on being compared to a second Street passed to a parameter.
• Return True or False, indicating whether the first street needs repairs more urgently than
the second.
• Streets in “poor” condition need repairs more urgently than streets in “fair” condition, which
need repairs more urgently than streets in “good” condition.
• If the condition of two Streets is the same, the one with more traffic is given higher priority.
Street Class
Step 3:
• Add a global constant COST_PER_KM = 1400000, to represent how
much it costs to repair each kilometer of road.
• Examine the format of the file streetData.txt.
• Add code to read streets from file, and create a list of Street objects.
• (Print a list of all streets, to verify that you have read them correctly.)
• Print a list of the streets in poor condition, and calculate the total cost
of repairing all of them.
Step 3:
• Add a global constant COST_PER_KM = 1400000, to represent how
much it costs to repair each kilometer of road.
• Examine the format of the file streetData.txt.
• Add code to read streets from file, and create a list of Street objects.
• (Print a list of all streets, to verify that you have read them correctly.)
• Print a list of the streets in poor condition, and calculate the total cost
of repairing all of them.
Step 4: Additional Practice
• Modify the code that constructs the Street list, so that the Streets are
ordered by priority in the list.
• Do not use a sort() function. Write your own sort, or preferably, perform an
ordered insert as you are reading streets from the txt file.
• (Make use of your compare method)
• If you have $40 million to spend on repairs, which streets could be
repaired?
• Modify the code that constructs the Street list, so that the Streets are
ordered by priority in the list.
• Do not use a sort() function. Write your own sort, or preferably, perform an
ordered insert as you are reading streets from the txt file.
• (Make use of your compare method)
• If you have $40 million to spend on repairs, which streets could be
repaired?
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 4 steps with 3 images

Knowledge Booster
Similar questions
- Use Java Programming Language Create a Loan Account Hierarchy consisting of the following classes: LoanAccount, CarLoan, PrimaryMortgage , UnsecuredLoan, and Address. Each class should be in it's own .java file. The LoanAccount class consists of the following properties: principal- the original amount of the loan. annualInterestRate - the annual interest rate for the loan. It is not static as each loan can have it's own interest rate. months - the number of months in the term of the loan, i.e. the length of the loan. and the following methods: a constructor that takes the three properties as parameters. calculateMonthlyPayment() - takes no parameters and calculates the monthly payment using the same formula as Assignment 1. getters for the three property variables. toString() - displays the information about the principle, annualInterestRate, and months as shown in the example output below. The CarLoan class which is a subclass of the LoanAccount class and consists of the…arrow_forwardCould you please help me with this chapter 6 number 15 Programming challenge from Starting out with Java Gaddis 7th edition book. Thank youarrow_forwardI need help with this PLEASE NO JAVA NO C++ ONLY PYTHON PLZ Create a class object with the following attributes and actions: Class Name Class number Classroom Semester Level Subject Actions: Store a class list Print the class list as follows: Class name Class Number Semester Level Subject Test your object: Ask the user for all the information and to enter at least 3 classes test using all the actions of the object print using the to string action Describe the numbers and text you print. Do not just print numbers or strings to the screen explain what each number represents.arrow_forward
- Chapter 15 Customer Employee Creator Create an object-oriented program that allows you to enter data for customers and employees. The person related classes will be stored in a module separate from the main function application module. Console Customer/Employee Data Entry Customer or employee? (c/e): c DATA ENTRY First name: Frank Last name: Wilson Email: frank44@gmail.com Number: M10293 CUSTOMER Full name: Frank Wilson Email: Number: Continue? (y/n): y Customer or employee? (c/e): e DATA ENTRY First name: Joel Last name: Murach Email: joel@murach.com SSN: 123-45-6789 EMPLOYEE Full name: Joel Murach Email: SSN: Bye! frank44@gmail.com Continue? (y/n): n ● M10293 ● Specifications Create a Person class that provides attributes for first name, last name, and email address. Include a parameter for each attribute in the constructor method. This class will provide a property or method that returns the person's full name. ● ● ● ● joel@murach.com 123-45-6789 Create a Customer class that…arrow_forwardRemaining Time: 35 minutes, 18 seconds, ¥ Question Completion Status: A class named Account is defined as the following. class Account } private: int id; double balance; public: //A constructor without parameter that creates a default account with id 0, balance 0 Account): /A constructor with the parameter that setting the id and balance Account(int, double), int getId); / return the ID double getBalance() //return the balance void withdraw(double amount) // the amount will be withdrawn void setlID(int), //set the ID void setBalance (double) //set the balance }: Assume that we will create a separate file of implementation of the function definition for the questions a) -b) a) Write a construction function definition with two parameters (ID and balance). b) Write a function definition of the function declaration (void withdraw(double amount):). c) Creates an Account object with an ID (1122) and balance ($20000). Then, write source codes to withdraw $2 MacBooarrow_forward6. Patient Charges Write a class named Patient that has attributes for the following data: • First name, middle name, and last name • Address, city, state, and ZIP code • Phone number ● The Patient class's init__ method should accept an argument for each attribute. The Name and phone number of emergency contact Patient class should also have accessor and mutator methods for each attribute. Next, write a class named Procedure that represents a medical procedure that has been performed on a patient. The Procedure class should have attributes for the following data: • Name of the procedure • Date of the procedure • Name of the practitioner who performed the procedure • Charges for the procedure 107 The Procedure class's __init__ method should accept an argument for each attribute. The Procedure class should also have accessor and mutator methods for each attribute Next, write a program that creates an instance of the Patient class, initialized with sample data. Then, create three…arrow_forward
- Javaarrow_forwardFocus on classes, objects, methods and good programming styleYour task is to create a BankAccount class. Class name BankAccount Attributes __balance float float __pin integer integer Methods __init_()get_pin()check_pin()deposit()withdraw()get_balance() The bank account will be protected by a 4-digit pin number (i.e. between 1000 and 9999). The pin should be generated randomly when the account object is created. The initial balance should be 0.get_pin()should return the pin.check_pin(pin) should check the argument against the saved pin and return True if it matches, False if it does not.deposit(amount) should receive the amount as the argument, add the amount to the account and return the new balance.withraw(amount) should check if the amount can be withdrawn (not more than is in the account), If so, remove the argument amount from the account and return the new balance if the transaction was successful. Return False if it was not.get_balance()…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
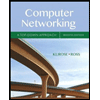
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
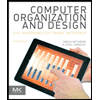
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
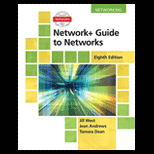
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
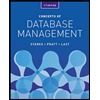
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
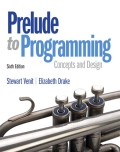
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
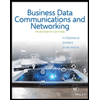
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY