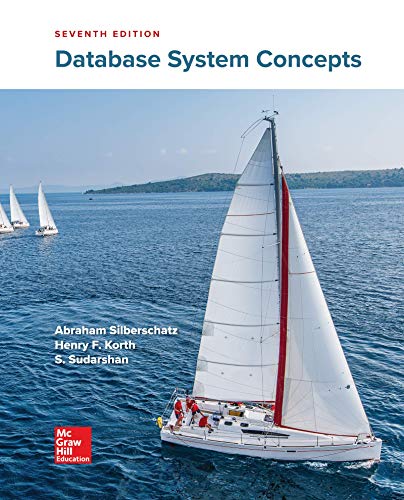
Concept explainers
Python program for this project:
Patient Charges
Write a class named Patient that has attributes for the following data:
- First name, middle name, and last name
- Address, city, state, and ZIP code
- Phone number
- Name and phone number of emergency contact
The Patient class’s _ _init_ _ method should accept an argument for each attribute. The Patient class should also have accessor and mutator methods for each attribute.
Next, write a class named Procedure that represents a medical procedure that has been performed on a patient. The Procedure class should have attributes for the following data:
- Name of the procedure
- Date of the procedure
- Name of the practitioner who performed the procedure
- Charges for the procedure
The Procedure class’s _ _init_ _ method should accept an argument for each attribute. The Procedure class should also have accessor and mutator methods for each attribute.
Next, write a program that creates an instance of the Patient class, initialized with sample data.
Then, create three instances of the Procedure class, initialized with the following data:
Procedure #1: Procedure #2: Procedure #3:
Procedure name: Physical ExamProcedure name: X-ray Procedure name: Blood test
Date: Today’s date Date: Today’s date Date: Today’s date
Practitioner: Dr. Irvine Practitioner: Dr. Jamison Practitioner: Dr. Smith
Charge: 250.00 Charge: 500.00 Charge: 200.00
The program should display
the patient’s information,
information about all three of the procedures,
and the total charges of the three procedures.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- Write a program that will contain an array of person class objects. The program should include two classes: 1) One class will be the person class. 2) The second class will be the team class, which contain the main method and the array or array list. The person class should include the following data fields; Name Phone number Birth Date Jersey Number Be sure to include get and set methods for each data field.The team class should contain the data fields for the team, such as: Team name Coach name Conference name The program should include the following functionality. Add person objects to the array; Find a specific person object in the array; (find a person using any data field you choose such as name or jersey number) Output the contents of the array, including all data fields of each person object. (display roster)arrow_forwardThere are two types of data members in a class: static and non-static. Give an example of real-world use for a static data member.arrow_forwardIn order for a derived class to override a method of the base class, you need to use the __ keyword in the method header of the base class. Question 7 options: extends override virtual basearrow_forward
- Written in Python It should have an init method that takes two values and uses them to initialize the data members. It should have a get_age method. Docstrings for modules, functions, classes, and methodsarrow_forwardThere are two types of data members in a class: static and non-static. Provide an example of when it might be useful to have a static data member in the actual world.arrow_forwardThis is Phython Programmingarrow_forward
- B. Pet Class Using Python Program, Write a class named Pet, which should have the following data attributes: • _ _name (for the name of a pet) •__animal_type (for the type of animal that a pet is. Example values are 'Dog', 'Cat', and `Bird')' __age (for the pet's age) init The Pet class should have an method that creates these attributes. It should also have the following methods: set_name() This method assigns a value to the __name field. set_animal_type() This method assigns a value to the __animal_type field. • set_age() This method assigns a value to the • get_name() This method returns the value of the get_animal_type() This method returns the value of the __animal_type field. • get_age() This method returns the value of the _age field. Once you have written the class, write a program that creates an object of the class and prompts the user to enter the name, type, and age of his or her pet. This data should be stored as the object's attributes. Use the object's accessor methods…arrow_forwardclass Widget: """A class representing a simple Widget === Instance Attributes (the attributes of this class and their types) === name: the name of this Widget (str) cost: the cost of this Widget (int); cost >= 0 === Sample Usage (to help you understand how this class would be used) === >>> my_widget = Widget('Puzzle', 15) >>> my_widget.name 'Puzzle' >>> my_widget.cost 15 >>> my_widget.is_cheap() False >>> your_widget = Widget("Rubik's Cube", 6) >>> your_widget.name "Rubik's Cube" >>> your_widget.cost 6 >>> your_widget.is_cheap() True """ # Add your methods here if __name__ == '__main__': import doctest # Uncomment the line below if you prefer to test your examples with doctest # doctest.testmod()arrow_forwardclass Student: def __init__(self, id, fn, ln, dob, m='undefined'): self.id = id self.firstName = fn self.lastName = ln self.dateOfBirth = dob self.Major = m def set_id(self, newid): #This is known as setter self.id = newid def get_id(self): #This is known as a getter return self.id def set_fn(self, newfirstName): self.fn = newfirstName def get_fn(self): return self.fn def set_ln(self, newlastName): self.ln = newlastName def get_ln(self): return self.ln def set_dob(self, newdob): self.dob = newdob def get_dob(self): return self.dob def set_m(self, newMajor): self.m = newMajor def get_m(self): return self.m def print_student_info(self): print(f'{self.id} {self.firstName} {self.lastName} {self.dateOfBirth} {self.Major}')all_students = []id=100user_input = int(input("How many students: "))for x in range(user_input): firstName = input('Enter…arrow_forward
- I need help with this PLEASE NO JAVA NO C++ ONLY PYTHON PLZ Create a class object with the following attributes and actions: Class Name Class number Classroom Semester Level Subject Actions: Store a class list Print the class list as follows: Class name Class Number Semester Level Subject Test your object: Ask the user for all the information and to enter at least 3 classes test using all the actions of the object print using the to string action Describe the numbers and text you print. Do not just print numbers or strings to the screen explain what each number represents.arrow_forwardExercise 1-Account class • Design a class named Account that contains : • A private int data field named id for the account • A private double data field named balance for the account • A privet Date data field named dateCreated that stores the date when the account was created • A no-arg constructor that creates a default account • A constructor that creates an account with the specified id and initial balance • The getters (i.e., accessors) and setters (i.e., mutators) methods for id and balance • The getter method for dateCreated • A method named withdraw that withdraws a specified amount from the account • A method named deposit that deposits a specified amount to the accountarrow_forwardCode should be in Pythonarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
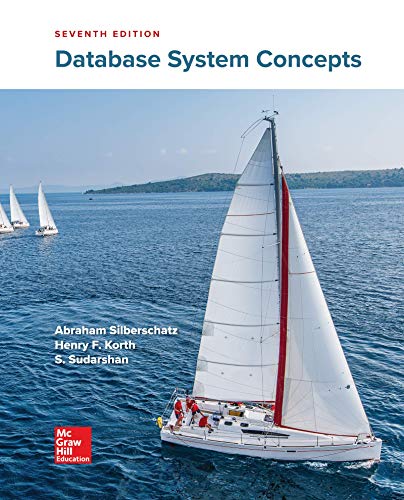
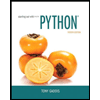
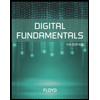
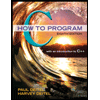
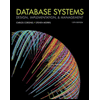
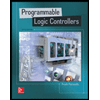