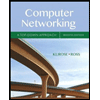
Question 3
The program below reads two integers. Then a function with the following
prototype is called:
void div_rem(int a, int b, int *divides, int *remains);
This function is passed the two integers. It divides them (using integer division),
and writes the answer over wherever “divides” points. Then it finds the
remainder and writes it into where “remains” points. Thus for 20 and 3, 20
divided by 3 is 6, remainder 2. Implement the div_rem function.
#include <stdio.h>
void div_rem(int a, int b, int *divides, int *remains);
int main(void)
{
int a, b;
int div = 0;
int rem = 0;
printf("enter two integers ");
scanf("%i %i", &a, &b);
div_rem(a, b, &div, &rem);
printf("%i divided by %i = %i "
"remainder %i\n", a, b, div, rem);
return 0;
}

Step by stepSolved in 2 steps

- Write a program that generates random numbers between -25 and 25 by using the randint function. The program will add up the numbers and terminate when the sum of the values becomes zero. The program should contain the following functions: add() : add up the values main(): the function where the program execution should begin and end. randomgen(): a function that returns a number between -25 and 25 A global variable subsetsum that keeps track of the sum The program should output the count of the number of values generated before exiting saying that the subset sum was zero.arrow_forwardAlert dont submit AI generated answer.arrow_forwardPython programming also screenshotarrow_forward
- Function scale multiples its first argument (a real number) by 10 raised to the power indicated by its second argument (an integer). For example, the function call scale (2.5, 2) double scale (double x, int n) double scale_factor; scale factor - pow (10, n); return x*scale_factor; Returns the value 250.0 (2.5 x 102). The function call scale (2.5, -2) Return the value 0.025 (2.5 x 10²) Write only the main function, create and initialize a double array and then call the function scale for each elements of the array. At the end, the contents of the array must consist of scaled values. Create and use a separate integer array as the powers of each corresponding value. Eg. If double array has the following contents: 1.0 2.0 3.0 4.0 and the Integer array of powers have the following: 4 3. 2 -2, After function calls, the new contents of the double array must be updated as in seen in the following: 1.0x104 2.0x10³ 3.0x10² 4.0x10²arrow_forwardJava A jiffy is the scientific name for 1/100th of a secondarrow_forwardPYTHON Question 3: Rain or Shine Part 1: Alfonso will only wear a jacket outside if it is below 60 degrees or it is raining. Write a function that takes in the current temperature and a boolean value telling if it is raining and it should return True if Alfonso will wear a jacket and False otherwise. Try solving this problem with a single line of code. def wears_jacket(temp, raining): """ >>> wears_jacket(90, False) False >>> wears_jacket(40, False) True >>> wears_jacket(100, True) True """ *** YOUR CODE HERE *** Note that it should either return True or False based on a single condition, whose truthiness value will also be either True or False. Part 2: Rewrite the above function with a lambda expression.arrow_forward
- Toll roads have different fees at different times of the day and on weekends. Write a function CalcToll() that has three arguments: the current hour of time (int), whether the time is morning (bool), and whether the day is a weekend (bool). The function returns the correct toll fee (double), based on the chart below. Weekday Tolls Before 7:00 am ($1.15) 7:00 am to 9:59 am ($2.95) 10:00 am to 2:59 pm ($1.90) 3:00 pm to 7:59 pm ($3.95) Starting 8:00 pm ($1.40) Weekend Tolls Before 7:00 am ($1.05) 7:00 am to 7:59 pm ($2.15) Starting 8:00 pm ($1.10) Ex: The function calls below, with the given arguments, will return the following toll fees: CalcToll(8, true, false) returns 2.95CalcToll(1, false, false) returns 1.90CalcToll(3, false, true) returns 2.15CalcToll(5, true, true) returns 1.05 need help with code in c++arrow_forward***in python only*** use turtle function Define the concentricCircles function such that: It draws a series of concentric circles, where the first parameter specifies the radius of the outermost circle, and the second parameter specifies the number of circles to draw. The third and fourth parameters specify an outer color and an other color, respectively. The outer color is used for the largest (i.e., outermost) circle, and then every other circle out to the edge alternates between that color and the 'other' color. The difference between the radii of subsequent circles is always the same, and this difference is also equal to the radius of the smallest circle. Put another way: the distance between the inside and outside of each ring is the same. Define concentricCircles with 4 parameters Use def to define concentricCircles with 4 parameters Use any kind of loop Within the definition of concentricCircles with 4 parameters, use any kind of loop in at least one place. Call…arrow_forward//Write a function that loops through and console.log's the numbers from 1 to 100, except multiples of three, log (without quotes) "VERY GOOD" instead of the number, for the multiples of five, log (without quotes) "SUPER AWESOME". For numbers which are multiples of both three and five, log (without quotes) "VERY GOOD SUPER AWESOME" Console log out: 12VERY GOOD4SUPER AWESOMEVERY GOOD78VERY GOODSUPER AWESOME11VERY GOOD1314VERY GOOD SUPER AWESOME1617VERY GOOD19SUPER AWESOMEVERY GOOD2223VERY GOODSUPER AWESOME26VERY GOOD2829VERY GOOD SUPER AWESOMEarrow_forward
- Ex. 8.4) The following functions are all intended to check whether a string contains any lowercase letters, but at least some of them are wrong. For each function, describe what the function actually does (assuming that the parameter is a string).arrow_forwardPart (a) Write a python function that computes the binomial coefficient ("). The function should return the correct answer for any positive integer n and k where k=m pass Part (c) Suppose that the number of people in the trial is 100. Then: • Plot a curve that shows how the probability of type 1 error changes with the choice of m, for m = 1,...n assuming that the null hypothesis holds (in red), • On the same picture, plot the probability of type 2 error vs the value of m in the case in which the new drug is effective with proability 0.68 (in blue). You can plot the two curves using matplotlib.pyplot. You can select the color by passing color='r' or color='b' to the plt.plot() function. [4]: n - 100 # your code here def plot_curve (): pass [5]: plot_curve() Part (d) Based on the picture above, what value of m do you think would be suitable to keep both type 1 and type 2 error small at the same time? (You may assume that the company claims the new drug has 68% accuracy) [6]: # your…arrow_forwardCreate a function that determines whether each seat can "see" the front-stage. A number can "see" the front-stage if it is strictly greater than the number before it. Everyone can see the front-stage in the example below: # FRONT STAGE [[1, 2, 3, 2, 1, 1], [2, 4, 4, 3, 2, 2], [5, 5, 5, 5, 4, 4], [6, 6, 7, 6, 5, 5]] # Starting from the left, the 6 > 5 > 2 > 1, so all numbe # 6 > 5 > 4 > 2 - so all numbers can see, etc. Not everyone can see the front-stage in the example below: # FRONT STAGE [[1, 2, 3, 2, 1, 1], [2, 4, 4, 3, 2, 2], [5, 5, 5, 10, 4, 4], [6, 6, 7, 6, 5, 5]] # The 10 is directly in front of the 6 and blocking its varrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
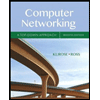
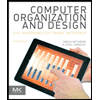
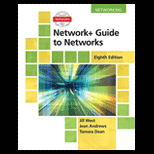
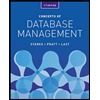
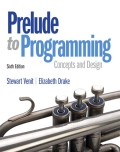
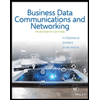