Coding hints: def lapstomiles(user_laps): return user_laps / 4.00 #Please note that one mile is equal to four laps … print(f'{num_miles:.2f}') #We are formatting the laps into two decimal places. One lap around a standard high-school running track is exactly 0.25 miles. Define a function named laps_to_miles that takes a number of laps as a parameter, and returns the number of miles. Then, write a main program that takes a number of laps as an input, calls function laps_to_miles() to calculate the number of miles, and outputs the number of miles. Output each floating-point value with two digits after the decimal point, which can be achieved as follows: print(f'{your_value:.2f}')
This is the second lab for the Unit 4 zyBooks materials.
Coding hints:
def lapstomiles(user_laps):
return user_laps / 4.00 #Please note that one mile is equal to four laps …
print(f'{num_miles:.2f}') #We are formatting the laps into two decimal places.
One lap around a standard high-school running track is exactly 0.25 miles. Define a function named laps_to_miles that takes a number of laps as a parameter, and returns the number of miles. Then, write a main program that takes a number of laps as an input, calls function laps_to_miles() to calculate the number of miles, and outputs the number of miles.
Output each floating-point value with two digits after the decimal point, which can be achieved as follows:
print(f'{your_value:.2f}')
Ex: If the input is:
7.6the output is:
1.90Ex: If the input is:
2.2the output is:
0.55The program must define and call the following function:
def laps_to_miles(user_laps)
# Define your function here
if __name__ == '__main__':
user_laps = float(input());
num_miles = laps_to_miles(user_laps)
print(f'{num_miles:.2f}')

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

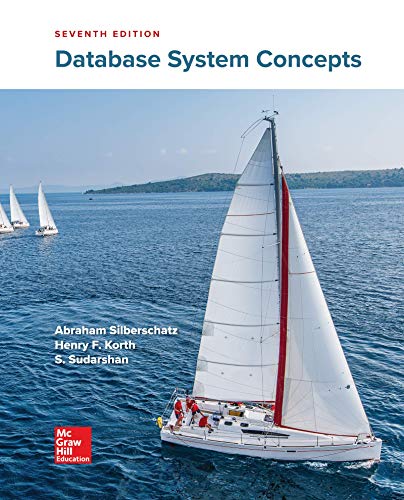
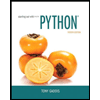
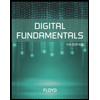
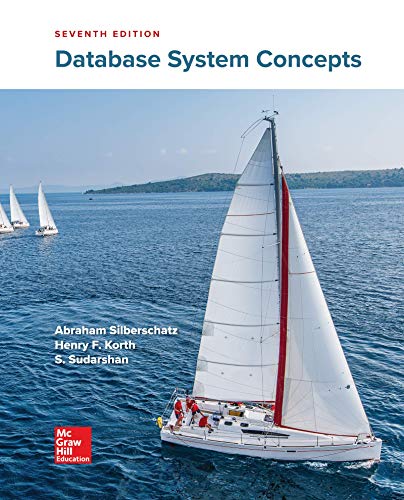
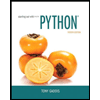
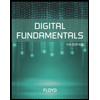
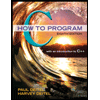
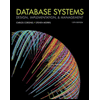
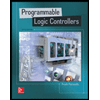