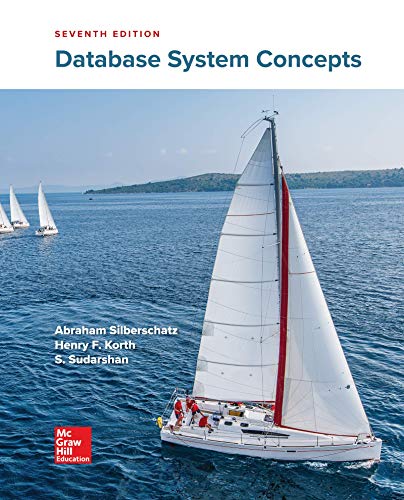
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
In python
The Nim game. Write a program for a two-player version of the game Nim: a human player will play against the computer. The game is simple: players take turns removing from 1 to 4 sticks from a pile of 13 sticks; the player who picks up the last stick wins the game.
Here’s some pseudocode for the Nim game.
Start by initializing some variables that will allow you to keep track of the state of the game:
- One variable should record how many sticks there are left in the pile; initially, this variable must be initialized to 13.
- There are two players in the game, who take turns. To remember who’s turn is next, use a variable with two states (= values). Say the variable is 1 if the human player is next, and it is 2, if the computer’s turn is next. Pick randomly the player who should start the game.
Then, start the main loop of the game: the game should continue for as long as there still are sticks (at least one) to pick. In each repetition of the game loop, one player will play their turn:
- First, print the current “board” – in this version, the whole game will be played in the console. The “board” will simply be a representation of the sticks that are left to play; you should also state textually how many sticks there are left. Here’s the “board” at the beginning of the game:
| | | | | | | | | | | | | 13 sticks remaining
- Before you let the next player play, determine what is the maximum number of sticks that can be removed in this turn: that is 4, if there are at least 4 sticks left, or the number of remaining sticks, if less than 4 sticks remain. The minimum number of sticks to remove is always 1.
- Next, one player plays their turn. If it’s the human player’s turn, show them a message asking how many sticks they want to pick (from 1 to the maximum that you determined before), and get their input. Validate the input; this means you must check if the player input a correct number, in the allowed range. If they didn’t input correctly, then keep asking for their input until it is correct.
- If it’s the computer’s turn, then have your program pick a random number of sticks to play if the number of sticks is greater than 4 on the board. If it is less than 4 have the computer pick them up and win the game. This is a very rudimentary strategy and the human should always win. Show a message in the console to inform the human player of how the computer plays its turn.
- Once the current player – human or computer – has picked the number of sticks to remove, those sticks will be removed from the remaining stack.
- If there still are sticks to play, switch who’s turn it is next. This means that you will need to switch between the two possible values for the variable that keeps track of the current player; for example, if it was 1 in this turn, it should be changed to 2 for the next turn. You need to do this so that you know whose turn it is the next time through the game loop.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- The game of Nim starts with a random number of stones between 15 and 30. Two players alternate turns and on each turn may take either 1, 2, or 3 stones from the pile. The player forced to take the last stone loses. Create a nim application in python that allows the user to play against the computer. In this version of the game, the application generates the number of stones to begin with, the number of stones the computer takes, and the user goes first. The Nim application code should: prevent the user and the computer from taking an illegal number of stones. For example, neither should be allowed to take three stones when there are only 1 or 2 left. include an is_valid_entry() function to check user input. include a draw_stones() function that generates a random number from 1 to 3 for the number of stones the computer draws. include separate functions to handle the user’s turn and the computer’s turn. My problem is that the code is not outputting like it's supposed to.…arrow_forwardValidating User Input Summary In this lab, you will make additions to a Java program that is provided. The program is a guessing game. A random number between 1 and 10 is generated in the program. The user enters a number between 1 and 10, trying to guess the correct number. If the user guesses correctly, the program congratulates the user, and then the loop that controls guessing numbers exits; otherwise, the program asks the user if he or she wants to guess again. If the user enters a "Y", he or she can guess again. If the user enters "N", the loop exits. You can see that the "Y" or an "N" is the sentinel value that controls the loop. Note that the entire program has been written for you. You need to add code that validates correct input, which is "Y" or "N", when the user is asked if he or she wants to guess a number, and a number in the range of 1 through 10 when the user is asked to guess a number. Instructions Ensure the file named GuessNumber.java is open. Write loops…arrow_forwardin Java Tasks Write a program that lets the user play the game of Rock, Paper, Scissors against the computer. The program should work as follows. 1. When the program begins, a random number in the range of 1 through 3 is generated. If the number is 1, then the computer has chosen rock. If the number is 2, then the computer has chosen paper. If the number is 3, then the computer has chosen scissors. (Don't display the computer's choice yet.) 2. The user enters his or her choice of "rock", "paper", or "scissors" at the keyboard. (You can use a menu if you prefer.) 3. The computer's choice is displayed. Tasks 4. A winner is selected according to the following rules: a. If one player chooses rock and the other player chooses scissors, then rock wins. (The rock smashes the scissors.) b. If one player chooses scissors and the other player chooses paper, then scissors wins. (Scissors cuts paper.) C. If one player chooses paper and the other player chooses rock, then paper wins. (Paper wraps…arrow_forward
- In a C# Console App do the following Write a method that takes three integers and returns true if their sum is divisible by 3, false otherwise. Write a method that takes two strings and displays the string that has fewer characters. Write a method that takes two bool variables and returns true if they have the same value, false otherwise. Write a method that takes an int and a double and returns their product.arrow_forwardHow do you make a username password generator in pythonarrow_forwardIn JAVA pleasearrow_forward
- In Java Golf scores record the number of strokes used to get the ball in the hole. The expected number of strokes varies from hole to hole and is called par (possible values: 3, 4, or 5). Each score's name is based on the actual strokes taken compared to par: "Eagle": number of strokes is two less than par "Birdie": number of strokes is one less than par "Par": number of strokes equals par "Bogey": number of strokes is one more than par Given two integers that represent the number of strokes used and par, write a program that prints the appropriate score name. Print "Error" at the end of the output if par or score is not in the expected range. If the input is: 3 4 the output is: Par 4 in 3 strokes is Birdiearrow_forwardIn java, create a program that will use a do while loop with a "yes/no" prompt. The program should include user input and output with getters and setters. Once the end of the loop is reached, ask the user if they'd like to do the program again. Answering "Yes" will start the program over again. Answering "No" will end the program. Answering with anything else will be an invalid input. Once the loop begins again, the program should also discard all previous inputs so that there won't be any overlapping. Use Scanner.nextLine();, it will help.arrow_forwardProgram in C++ & Visual Studio not Studio Code Everyone has played Yahtzee... Right? There are so many better dice games, but my family likes this one the best. A YouTube video that describes Yahtzee can be found here. The basic rules are as follows: At the start of your turn you roll five normal everyday six sided dice. In the course of your turn you would choose to reroll any of the dice up to two more times. We are more concerned in this problem with the dice at the completion of your turn. This website gives a great rundown of the probabilities of Yahtzee that you will need. NOTE: You may ONLY use a set and map data structure to complete the solution to this problem Let’s see what results the dice give us using the following data structures and process: Prompt the user to enter five valid numbers (Range: One to six inclusive on both ends) Each time the user enters a valid number, “place” it into a map of integersCreate and use a set of integers with your data to…arrow_forward
- Design and implement an application that plays the Hi-Lo guessing game with numbers. The program should pick a random number between 1 and 100 (inclusive) and then repeatedly prompt the user to guess the number. On each guess, report to the user that he or she is correct or that the guess is high or low. Continue accepting guesses until the user guesses correctly or chooses to quit. Use a sentinel value to determine whether the user wants to quit. Count the number of guesses, and report that value when the user guesses correctly. At the end of each game (by quitting or a correct guess), prompt to determine whether the user wants to play again. Continue playing games until the user chooses to stop.arrow_forwardA retail company assigns a $5000 store bonus if monthly sales are $100,000 or more. Additionally, if their sales exceed 125% or more of their monthly goal of $90,000, then all employees will receive a message stating that they will get a day off. Step 3: Complete the pseudocode by writing the missing lines. When writing your modules and making calls, be sure to pass necessary variables as arguments and accept them as reference parameters if they need to be modified in the module. (Reference: Writing a Decision Structure in Pseudocode, page 118). Module main () //Declare local variables Declare Real monthlySales //Function calls Call getSales(monthlySales) ______________________________________________________ ______________________________________________________ End Module //this module takes in the required user input Module getSales(Real Ref monthlySales) Display “Enter the total sales for…arrow_forwardUnderstanding ifStatements Summary In this lab, you complete a prewritten Java program for a carpenter who creates personalized house signs. The program is supposed to compute the price of any sign a customer orders, based on the following facts: The charge for all signs is a minimum of $35.00. The first five letters or numbers are included in the minimum charge; there is a $4 charge for each additional character. If the sign is made of oak, add $20.00. No charge is added for pine. Black or white characters are included in the minimum charge; there is an additional $15 charge for gold-leaf lettering. Instructions 1. Ensure the file named HouseSign.java is open. 2. You need to declare variables for the following, and initialize them where specified: A variable for the cost of the sign initialized to 0.00 (charge). A variable for the number of characters initialized to 8 (numChars). A variable for the color of the characters initialized to "gold" (color). A variable for the…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
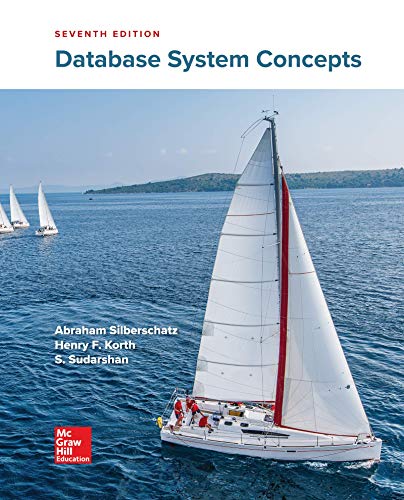
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
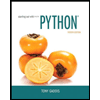
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
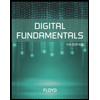
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
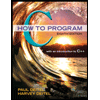
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
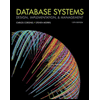
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
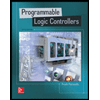
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education