code should be in python: I need the user who's running the code to pick a game from 1 to 5 and the game they pick should work properly and let me know if any code errors can be fixed. I have made each game but i cant figure out how to make the user pick the game they want to try. here's my code: #game 1 you're supposed to input a sequence and the values are supposed to be shown how many times they are shown:
code should be in python:
I need the user who's running the code to pick a game from 1 to 5 and the game they pick should work properly and let me know if any code errors can be fixed. I have made each game but i cant figure out how to make the user pick the game they want to try.
here's my code:
#game 1 you're supposed to input a sequence and the values are supposed to be shown how many times they are shown:
ip = input("Please enter your sequence:")
print("In the sequence we saw:")
for i in range(10):
k = 0
k = sum(int(c) == i for c in ip)
if k > 0:
print("{}: {} time(s)".format(i, k))
#game 2 the user is supoosed to enter 2 values as a range and it shows the possible 3 multipication in that range:
num1 = int(input("Enter Number 1:"))
num2 = int(input("Enter Number 2:"))
if(num1>num2):
for i in range(num2+1,num1):
if(i%3 == 0):
print(i)
if(num1<num2):
for i in range(num1+1,num2):
if(i%3 == 0):
print(i)
#game 3 is a
def largestWord(s):
s = sorted(s, key = len)
print("Result : ",s[-1],len(s[-1]))
if __name__ == "__main__":
s = input("Enter the string: ")
l = list(s.split(" "))
largestWord(l)
# game 4 is a function that receives a list as its only parameter. Inside the body of the function and removes all the duplicate items from the list:
def remove_duplicates (Data):
new_Data = []
for item in Data:
if (item not in new_Data):
new_Data.append(item)
return(new_Data)
print(remove_duplicates(["hey","swim","day","night","swim"]))
#game 5 function that receives a list of strings as its only parameter. Inside the body of the function and it finds the first palindromic word and returns it:
def palinCheck(lst):
for i in lst:
if i==i[::-1]:
return i
return ""
lst=["abc","hello","car","level","mom"]
print(palinCheck(lst))

Step by step
Solved in 2 steps

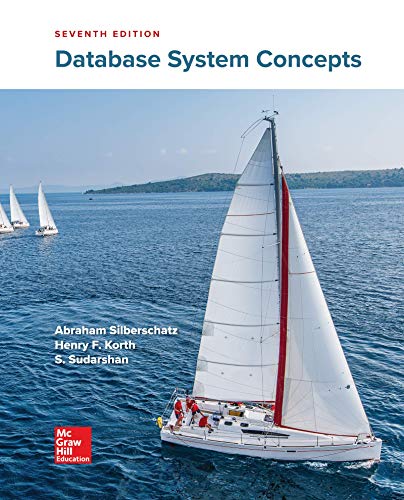
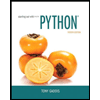
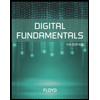
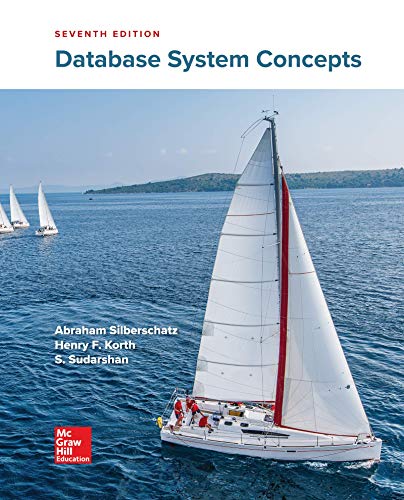
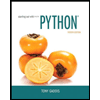
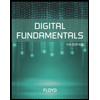
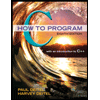
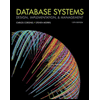
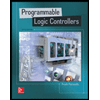