The BankAccount class described below is used to represent a personal savings account with a yearly interest rate, (represented as a percentage, e.g., a 7% rate is represented by .07). The interest is applied monthly, (i.e., at the end of each month 1/12 of the interest is deposited into the account). public class BankAccount { public BankAccount(double rate) public BankAccount(double initBal, double rate) public double getBalance() public double getIntRate() public void setIntRate(double rate) public void deposit(double amount) public void withdraw(double amount) public void addMonthsInterest() private double balance; private double intRate; } Suppose a bank offers to its special customers a new type of savings account, called a “Credit Account”, that allows the customer to withdraw extra money if needed, so that the account balance is allowed to be negative at some times. These accounts award interest at the end of every month, as long as the balance is positive. If the balance is negative, then the account is charged the monthly interest for the negative amount, plus 0.5%. In addition, any withdrawals to an account with a non-positive balance are charged a fee of $2. Provide the code for this extension class. Be sure to include constructor methods and override th
The BankAccount class described below is used to represent a personal savings account with a yearly interest rate, (represented as a percentage, e.g., a 7% rate is represented by .07). The interest is applied monthly, (i.e., at the end of each month 1/12 of the interest is deposited into the account).
public class BankAccount
{ public BankAccount(double rate)
public BankAccount(double initBal, double rate)
public double getBalance()
public double getIntRate()
public void setIntRate(double rate)
public void deposit(double amount)
public void withdraw(double amount)
public void addMonthsInterest()
private double balance;
private double intRate;
}
Suppose a bank offers to its special customers a new type of savings account, called a “Credit Account”, that allows the customer to withdraw extra money if needed, so that the account balance is allowed to be negative at some times. These accounts award interest at the end of every month, as long as the balance is positive. If the balance is negative, then the account is charged the monthly interest for the negative amount, plus 0.5%.
In addition, any withdrawals to an account with a non-positive balance are charged a fee of $2. Provide the code for this extension class. Be sure to include constructor methods and override the appropriate methods.

Step by step
Solved in 3 steps with 3 images

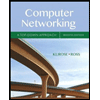
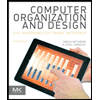
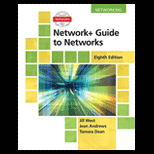
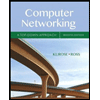
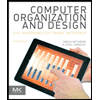
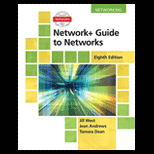
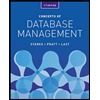
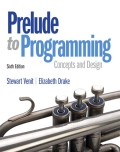
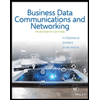