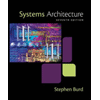
Concept explainers
A Barbershop Quartet is a group of four singers: a lead, a tenor, a bass, and a baritone. These four singers harmonize to produce a purely American form of music developed in the 19th century. Consider the incomplete class definitions below.
public class BarberShopQuartet
{
public BarberShopQuartet()
{
Tenor highSinger = new Tenor();
Baritone bari = new Baritone();
Lead melody = new Lead();
Bass lowSinger = new Bass();
System.out.println(“Quartet created.”);
}
}
______________________________________________________________________________
public class Singer
{
public Singer()
{
System.out.println(“Singing...”);
}
}
______________________________________________________________________________
public class Tenor extends Singer
{
public Tenor()
{
System.out.println(“Singing...the high notes”);
}
}
______________________________________________________________________________
public class Baritone extends Singer
{
public Baritone()
{
System.out.println(“Singing...harmonizing with the lead”);
}
}
______________________________________________________________________________
public class Lead extends Singer
{
public Lead()
{
System.out.println(“Singing...the melody and providing the
emotion”);
}
}
______________________________________________________________________________
public class Bass extends Singer
{
public Bass()
{
System.out.println(“Singing...the very low notes”);
}
}
Which of the following IS-A, HAS-A relationships is true?
A. |
A Baritone has-a BarberShopQuartet. |
|
B. |
A Singer has-a Lead. |
|
C. |
A BarberShopQuartet is-a Singer. |
|
D. |
A Singer has-a BarberShopQuartet.
|
|
E. |
A Tenor is-a Singer. |

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- A Barbershop Quartet is a group of four singers: a lead, a tenor, a bass, and a baritone. These four singers harmonize to produce a purely American form of music developed in the 19th century. Consider the incomplete class definitions below. public class BarberShopQuartet { public BarberShopQuartet() { Tenor highSinger = new Tenor(); Baritone bari = new Baritone(); Lead melody = new Lead(); Bass lowSinger = new Bass(); System.out.println(“Quartet created.”); } } ______________________________________________________________________________ public class Singer { public Singer() { System.out.println(“Singing...”); } } ______________________________________________________________________________ public class Tenor extends Singer { public Tenor() { System.out.println(“Singing...the high notes”); } } ______________________________________________________________________________ public class Baritone extends…arrow_forwardShape -width: double -length: double +allmutatorMethods Shapes2D Shapes3D -height:double *calSize():double +calVolume():double 1. Define class Shape, 2DShape and 3DShape as per UML class diagram given. 2. Define all mutator methods(set and get) for all classes. 3. Define methods calArea() with formula area - width * length for 2DShapes. 4. Define methods calArea() with formula calvolume, volume = width*length*height for 3DShapes. In the main program, declare and set data in 1 object of 3DShapes. Create an array OR ArrayList of 2DShapes, set and display the info of all objects as shown in the output. 5.arrow_forward49. Consider the following class definition. public class Cylinder } private double baseRadius; private double height; public Cylinder () baseRadius = 0; height = 0; public Cylinder (double 1, double h) } baseRadius - 1; height = h; { public void set (double r, double h) } baseRadius = r; height = h; { public String toString () } return (baseRadius + It n + height); { public double SurfaceArea () return 2 * 3.14 * baseRadius height; public double volume () } return 3.14 baseRadius * baseRadius * height; { Which of the following statements correctly instantiates the Cylinder object myCylinder? (i) Cylinder mycylinder = new Cylinder (2.5, 7.3) : (ii) class Cylinder myCylinder = new Cylinder (2.5, 7.3); (ii) myCylinder new Cylinder (2.5, 7.3); %3D a. Only (i) b. Only (i) c. Only (iüi) d. Both (ii) and (ii)arrow_forward
- public class Father { public String name; public int age; // customer name Father(){ name=”Hassan”; age=50;} public void display(){ System.out.println(“Name:”+name); System.out.println(“Age:”+age); } } 1. Based on Figure 3, create a class named Child that inherits the Father class. Declare an instance name location (String) for class Child. 2. Define a constructor in class Child and give an appropriate initial values for the instance (name, age, location). 3. Define a method display () in class Child, execute the display ( ) method in superclass using the keyword super. Method display ( ) in class Child should print the information of name, age and location 4. Create other class named Main for the main method and create 2 objects for the 2 classes (Father and Child). Then execute the display ( ) method for Father and Child. Example of the output as in Figure 4: Father's info: Name: Josh Age: 50 Child's info: Name: Christy Age: 20 Location:…arrow_forwardThe Temperature class is an abstract class with two abstract methods. Create the Conversion class which inherits from Temperature. Use the formulas below to help with the temperature conversions. CODE: abstract class Temperature { public abstract double celsius(double temp); public abstract double fahrenheit(double temp);} //add class definitions below this line //add class definitions above this line public class Exercise3 { public static void main(String[] args) { //add code below this line Conversion c = new Conversion(); //add code above this line }}arrow_forward48. Consider the following class definition. public class Rectangle { private double length; private double width; public Rectangle () length = 0; width = 0; public Rectangle (double 1, double w) { length = l; width = w; public void set (double 1, double w) { length = 1; width = w; public void print () } System.out.println (length " * + width) ; public double area() return length width; public double perimeter () return 2 * length + 2 width; } Which of the following statements correctly instantiates the Rectangle object myRectangle? (i) myRectangle = new Rectangle (12.5, 6); (ii) Rectangle myRectangle = new Rectangle (12.5, 6); (iii) class Rectangle myRectangle = new Rectangle (12.5, 6); a. Only (i) b. Only (ii) c. Only (ii) d. Both (ii) and (iii)arrow_forward
- Finish the constructor for the KickBall class. public class Ball { public double diameter; public Ball(double diam) { diameter = diam; public class KickBall extends Ball private Color ballColor; public KickBall(double diam, Color ballColor) {arrow_forwardclass Time { private: int hour; int minute; int second; public: Time(): Time(0, 0, 0) { Time(int s): Time(0, 0, s) {} I Time(int m, int s): Time(0, m, s) {} Time(int h, int m, int s) { m += s/60; s %= 60; h += m/60; m %= 60; hour = h; minute = m; second = s; } int getHour() {return hour;} int getMinute() {return minute;} int getSecond() {return second;} void setHour(int h) {hour = h;} void setMinute(int m) {minute = m;} void setSecond(int s) {second = s;} }; Q1: Implement a class called Time. a) Use the given Time class implement following. b) Overload friend insertion operator > to input Time objects.arrow_forwardA(n) ____________ is one instance or variable of a class.arrow_forward
- Chapter 10 defined the class circleType to implement the basic properties of a circle. (Add the function print to this class to output the radius, area, and circumference of a circle.) Now every cylinder has a base and height, where the base is a circle. Design a class cylinderType that can capture the properties of a cylinder and perform the usual operations on the cylinder. Derive this class from the class circleType designed in Chapter 10. Some of the operations that can be performed on a cylinder are as follows: calculate and print the volume, calculate and print the surface area, set the height, set the radius of the base, and set the center of the base. Also, write a program to test various operations on a cylinder.arrow_forwardpython programming Implement a constructor for the Person class Implement a constructor for the Student class Create a student with name; Albert Einstein, ramq: 14031879-1235, address: 112 Mercer Street, Princeton, courses: (1)Physics & (2)Relativity where the grades were b and a respectively and with enrollment date: 1895 partial solution attached in the imagearrow_forwardclass overload { int x; double y; void add(int a , int b) { x = a + b; } void add(double c , double d) { y = c + d; } overload() { this.x 0; %3D this.y = 0; } %3D } class Overload_methods { public static void main(String args[]) { overload obj int a = 2; double b - 3.2; obj.add(a, a); obj.add(b, b); System.out.println(obj.x + } = new overload(); + obj.y); } #3Run the codearrow_forward
- Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
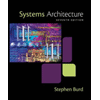
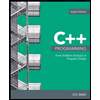