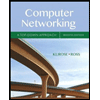
JAVA
Task
Write a JAVA class named Account to represent an account that you may find at a bank. Accounts have a balance and an annual interest rate. Include attributes in the Account class to reflect these.
Write getters for both attributes and a setter for only the annual interest rate. Think about how real bank accounts work, you change your balance by withdrawing and depositing money, not just by telling the banker how much you want to change it to.
Include a constructor with parameters used to assign values to both attributes.
Users should be able to withdraw money from an Account, deposit money into an Account, find monthly interest rate, and find monthy interest. Include methods for each of these actions. More details on them below.
withdraw method
This method simulates withdrawing money from the Account. It should not return a value and take the amount to withdraw as a parameter and reduce the balance by this amount.
deposit method
This method simulates depositing money into the Account. It should not return a value and take the amount to deposit as a parameter and increase the balance by this amount.
getMonthlyInterestRate method
This method calculates and returns the monthly interest rate. The interest rate that you have as an attribute is the annual interest rate. To "covert" the annual rate to the monthly rate, simply divide the annual rate by 12.
getMonthlyInterest method
This method calculates and returns the interest gained for the month. That is, take the balance and multiply it by the monthly interest rate.

The answer is given below.
Step by stepSolved in 3 steps with 1 images

- JAVA Practice Question for Midterm Write a class with a constructor that accepts a String object as its argument. The class should have a method that returns the number of vowels in the string, and another method that returns the number of consonants in the string. Demonstrate the class in a program by invoking the methods that return the number of vowels and consonants. Print the counts returned. Imagine you are developing a software package for an online shopping site that requires users to enter their own passwords. Your software requires that users’ passwords meet the following criteria: The password should be at least six characters long. The password should contain at least one uppercase and at least one lowercase letter. The password should have at least one digit. a program that asks the user to enter a password, then displays a message indicating whether it is valid or not.arrow_forwardQUICKLY please. Thank you. Please answer in python quickly Below is code that defines a Quadrilateral class (a shape with four sides), which has four floating point instance variables that correspond to the four side lengths: import math class Quadrilateral: def __init__(self,a,b,c,d): self.a = a self.b = b self.c = c self.d = d def perimeter(self): return self.a + self.b + self.c + self.d def area(self): print("Not enough information") def __lt__(self, other): return self.perimeter() < other.perimeter() Define a Rectangle class that inherits from Quadrilateral, with the following methods: __init__(self, length, width): Override the __init__ method so that the Rectangle constructor only takes in two floating point numbers. Your constructor for Rectangle should only consist of a call to Quadrilateral’s constructor: don’t define any new instance variables. Override the area method to…arrow_forwardImplement the inventory manager class. This class must have methods that do the following: Add a new item to the inventory manager. Remove an item from the inventory manager. Re-stock an item in the inventory manager. Display the items in the inventory manager. Search for an item in the inventory manager by at least two criteria (name, price, quantity, etc.). Part 3: Test the inventory manager class with a driver program or unit tests. This may be a console application.arrow_forward
- Java programarrow_forwardUse the scenario below for help answering 1(A), (B) and (C). This is using Java. The Scenario: You have a class named HeartsPlayer A round of Hearts starts with every player having 13 cards Players then choose 3 cards to “trade” with a player (1st you pass left, 2nd you pass right, 3rd you pass across, 4th you keep) Players then strategically play cards in order to have the lowest score At the end of the round, points are cumulatively totaled for each player. If one player’s total is greater than 100, the game ends and the player with the lowest score wins. Guide: Static & Not Final Field: Accessed by every object, Changing Non-Static & Final Field: Accessed by object itself, Non-Changing Static & Final: Accessed by every object, Non-Changing Non-Static & Not Final Field: Accessed by object itself, Changing 1. How should the following data fields be defined (with respect to final and static)? (a) playerPosition (These have values of North, South, East, or West)- Is…arrow_forwardDraw the UML diagram for the class. Implement the class. Write a test program that creates an Account object with an account ID of 1122, a balance of $20,000, and an annual interest rate of 4.5%. Use the withdraw method to withdraw $2,500, use the deposit method to deposit $3,000, and print the balance, the monthly interest, and the date when this account was created.arrow_forward
- MUST BE IN JAVA. PLEASE USE COMMENTS AND WRITE THE CODE IN SIMPLEST FORM.arrow_forwardPlease teach me how to fix an error from my JAVA source code. I have set up the source code with class name "ProductionWorker" and there were a error occured on second page of sceenshot where it is highlighted. //import the file import java.util.*; //create the class class Employee { //create the variable private String emp_num; //create the date variable private Date hiring_date; // default constructor public Employee() { //assign the emp num. this.emp_num = "000-A"; //assign the Date this.hiring_date = new Date(); } // constructor parameter public Employee(String emp_num, Date hiring_date) { this.emp_num = emp_num; this.hiring_date = hiring_date; } // getter method public String getEmployeeNumber() { return this.emp_num; } // call the gethiringdate function public Date gethiringDate() { // return the date return this.hiring_date; } // setter method public void setEmployeeNumber(String emp_num) { //set the emp num. this.emp_num = emp_num; } //set the hiring date public void…arrow_forwardJava Help Please This is real please stop wasting time. Real Answer Please This questioned was answered in a wrong way several times someone else to answer it please Write a new class called DistanceFitnessTracker that extends FitnessTracker. DistanceFitnessTracker will represent a fitness tracker able torecord only distance. Here, you will not be asked to convert between distance units(Kilometers is the unit) Distance.java public class Distance {// Enum of distance unitspublic enum DistanceUnit {KILOMETRES,MILES}// Constantsprivate static final double KMS_PER_MILE = 1.609;private static final double MILES_PER_KM = 0.621;// Instance variablesprivate double value;private DistanceUnit distanceUnit;// Constructorspublic Distance(double value) {this.value = value;// Default unit is kmthis.distanceUnit = DistanceUnit.KILOMETRES;} public Distance(double value, DistanceUnit distanceUnit) {this.value = value;this.distanceUnit = distanceUnit;}// Methods: You should remove the comments and…arrow_forward
- JAVA Write an application to simulate the rolling of two dice. The application should use an object of class Random once to roll the first die and again to roll the second die. The sum of the two values should then be calculated. Each die can show an integer value from 1 to 6, so the sum of the values will vary from 2 to 12, with 7 being the most frequent sum, and 2 and 12 the least frequent.arrow_forwardWrite a program which controls a metro train and that keeps track of stations and who gets on and off. Design and implement a class called train, which has at least the following: At least the following attributes (you can include others): metroID stationNum which keeps track of the station that the train is at. iii. direction which keeps track of the direction the train is travelling in. passTotal which keeps track of the number of passengers currently on the train. A default constructor which sets the metroID to a random number between 1 and 1000, the station number to 0, the direction (int), and the passTotal to 0 (train is empty). A constructor with one parameter; the parameter is the metro id. The constructor assigns the passed integer to the metroID attribute; the rest of the attributes are set as described in the default constructor. Accessor methods for each attributes. Mutator methods for each attributes. nextStation(int lastStation) which determines the next…arrow_forwardDesign and implement the class Day that implements the day of the week in a program. The program should be able to perform the following operations on an object of the type Day: Set the day. Print the day. Return the day. Return the next day. Return the previous day. Add a comment with your full name in it in any part of the program.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
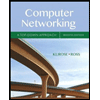
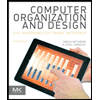
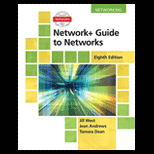
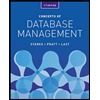
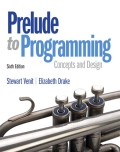
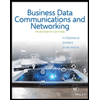