#Testing Please test my code and see if it meet the follwing needs: The class should implement the Comparable interface. Circle one is less than Circle two if the radius of Circle one is less than the radius of Circle two. The two Circles are equal if they have the same radius. Circle one is larger than Circle two if its radius is larger. If circle One.compareTo circle Two <> 0 The Test Class displays a menu that allows the user to: Enter a Circle (the user only needs to enter the radius). Print all Circles (print the toString for each Circle in the ArrayList). Quit To adda a circle Cases: The ArrayList is empty The new circle is less than the first circle, add it at the beginning. The circle is greater than the last circle, add it at the end The new circle belongs somewhere in the middle. import java.lang.Math;public class Circle implements Comparable<Circle> {private double radius;public Circle(double radius) {this.radius = radius;}public double findArea() {return Math.PI * Math.pow(this.radius, 2);}public double findCircumference() {return 2 * Math.PI * this.radius;}@Overridepublic boolean equals(Object obj) {if (this == obj) return true;if (obj == null || getClass() != obj.getClass()) return false;Circle circle = (Circle) obj;return Double.compare(circle.radius, radius) == 0;}@Overridepublic String toString() {return "Radius: " + this.radius + " Area: " + this.findArea() + " Circumference: " + this.findCircumference();}@Overridepublic int compareTo(Circle c) {return Double.compare(this.radius, c.radius);}} #Test Class import java.util.ArrayList;import java.util.Scanner;public class CircleTest {private ArrayList<Circle> circles = new ArrayList<>();public void start() {Scanner scanner = new Scanner(System.in);int choice;do {System.out.println("\n1. Enter a Circle\n2. Print all Circles\n3. Quit");choice = scanner.nextInt();switch (choice) {case 1:System.out.println("Enter the radius of the Circle:");double radius = scanner.nextDouble();Circle circle = new Circle(radius);addCircle(circle);break;case 2:printCircles();break;case 3:System.out.println("Quitting...");break;default:System.out.println("Invalid choice. Please try again.");}} while (choice != 3);scanner.close();}private void addCircle(Circle circle) {if (circles.isEmpty()) {circles.add(circle);} else if (circle.compareTo(circles.get(0)) < 0) {circles.add(0, circle);} else if (circle.compareTo(circles.get(circles.size() - 1)) > 0) {circles.add(circle);} else {for (int i = 0; i < circles.size(); i++) {if (circle.compareTo(circles.get(i)) < 0) {circles.add(i, circle);break;}}}}private void printCircles() {for (Circle circle : circles) {System.out.println(circle);}}public static void main(String[] args) {CircleTest test = new CircleTest();test.start();}}
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
#Testing
Please test my code and see if it meet the follwing needs:
The class should implement the Comparable interface. Circle one is less than Circle two if the radius of Circle one is less than the radius of Circle two. The two Circles are equal if they have the same radius. Circle one is larger than Circle two if its radius is larger. If circle One.compareTo circle Two <> 0
The Test Class displays a menu that allows the user to:
- Enter a Circle (the user only needs to enter the radius).
- Print all Circles (print the toString for each Circle in the ArrayList).
- Quit
To adda a circle
Cases:
- The ArrayList is empty
- The new circle is less than the first circle, add it at the beginning.
- The circle is greater than the last circle, add it at the end
- The new circle belongs somewhere in the middle.
public class Circle implements Comparable<Circle> {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
public double findArea() {
return Math.PI * Math.pow(this.radius, 2);
}
public double findCircumference() {
return 2 * Math.PI * this.radius;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null || getClass() != obj.getClass()) return false;
Circle circle = (Circle) obj;
return Double.compare(circle.radius, radius) == 0;
}
@Override
public String toString() {
return "Radius: " + this.radius + " Area: " + this.findArea() + " Circumference: " + this.findCircumference();
}
@Override
public int compareTo(Circle c) {
return Double.compare(this.radius, c.radius);
}
}
import java.util.Scanner;
public class CircleTest {
private ArrayList<Circle> circles = new ArrayList<>();
public void start() {
Scanner scanner = new Scanner(System.in);
int choice;
do {
System.out.println("\n1. Enter a Circle\n2. Print all Circles\n3. Quit");
choice = scanner.nextInt();
switch (choice) {
case 1:
System.out.println("Enter the radius of the Circle:");
double radius = scanner.nextDouble();
Circle circle = new Circle(radius);
addCircle(circle);
break;
case 2:
printCircles();
break;
case 3:
System.out.println("Quitting...");
break;
default:
System.out.println("Invalid choice. Please try again.");
}
} while (choice != 3);
scanner.close();
}
private void addCircle(Circle circle) {
if (circles.isEmpty()) {
circles.add(circle);
} else if (circle.compareTo(circles.get(0)) < 0) {
circles.add(0, circle);
} else if (circle.compareTo(circles.get(circles.size() - 1)) > 0) {
circles.add(circle);
} else {
for (int i = 0; i < circles.size(); i++) {
if (circle.compareTo(circles.get(i)) < 0) {
circles.add(i, circle);
break;
}
}
}
}
private void printCircles() {
for (Circle circle : circles) {
System.out.println(circle);
}
}
public static void main(String[] args) {
CircleTest test = new CircleTest();
test.start();
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

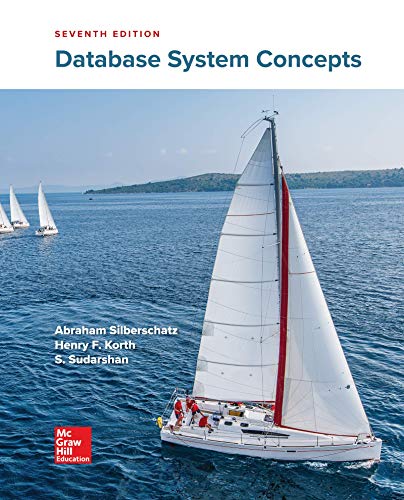
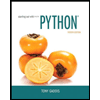
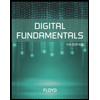
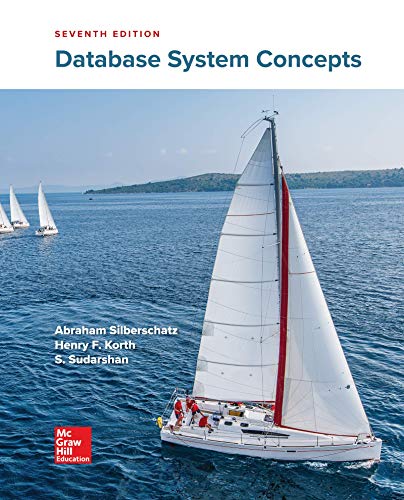
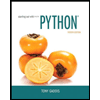
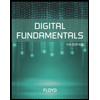
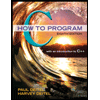
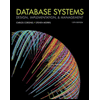
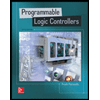