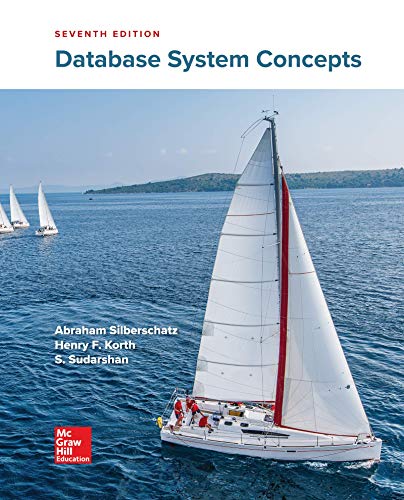
I need help coding in MATLAB. I want to create a function where I can input different values of n and e and I would get an output of gamma. Can you help me with that?
% Initial Conditions
rev = 0:0.001:4;
n1 = -1;
w1 = [n1; 0; 0];
g1 = deg2rad(8);
C1 = [cos(g1) -sin(g1) 0;
sin(g1) cos(g1) 0;
0 0 1];
[e1, norm_EP1] = DCMtoEP(C1);
% For n = -1 and e1
% Using ode45 to integrate the KDE and DDE
options = odeset('RelTol',1e-12,'AbsTol',1e-12);
result1 = ode45(@K_DDE, rev, [w1; e1; n1], options);
% Extracting information from the ode45 solver
v1 = result1.x;
w_ode1 = result1.y(1:3, :);
e_ode1 = result1.y(4:7, :);
% Finding C11
C11_1 = 1 - 2*e_ode1(2,:).^2 - 2*e_ode1(3,:).^2;
% Initializing arrays
gamma1 = zeros(1, length(v1));
% Finding the nutation angles
for i = 1:length(v1)
gamma1(i) = acosd(C11_1(i));
end
function dwedt = K_DDE(~, w_en)
% Extracting the initial condtions to a variable
w = w_en(1:3);
e = w_en(4:7);
n = w_en(8);
I = 400;
J = 150;
x = (J/I) - 1;
y = n - 1;
% Kinematic Differential Equations
dedt = zeros(4,1);
dedt(1) = pi*(e(4)*(w(1)-1-y) - e(3)*w(2) + e(2)*w(3));
dedt(2) = pi*(e(3)*(w(1)+1-y) + e(4)*w(2) - e(1)*w(3));
dedt(3) = pi*(-e(2)*(w(1)+1-y) + e(1)*w(2) + e(4)*w(3));
dedt(4) = pi*(-e(1)*(w(1)-1-y) - e(2)*w(2) - e(3)*w(3));
% Dynamical Differential Equations
dwdt = zeros(3,1);
dwdt(1) = 0;
dwdt(2) = -24*pi*(-x)*(e(1)*e(2)+e(3)*e(4))*(e(2)*e(3)-e(1)*e(4)) ...
+ 2*pi*(-x)*w(1)*w(3) - 2*pi*w(3)*y;
dwdt(3) = 12*pi*(-x)*(e(1)*e(2)+e(3)*e(4))*(1-2*e(3)^2-2*e(1)^2) ...
- 2*pi*(-x)*w(1)*w(2) + 2*pi*w(2)*y;
% Combining the w and C into one output matrix
dwedt = [dwdt; dedt; 0];
end

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- Question: The module timeit allows you to compute the time a function call takes. Verify your answer about the time complexity by computing the run time for randomly chosen arc diagrams of suitable sizes and producing an appropriate plot of the average run times against the lengths of the matchings. Can you help me to check my code and also can you provide an interpretation to my results(the graph).arrow_forwardPlot the following function for -5 ≤ x ≤ 5: y = 1 1+x² The x-values can be generated with x = np.linspace (-5, 5, n), where n refers to the number of x-values generated (you pick a n-value).arrow_forward2. Write the definition of a bool function named BooleanMode () whose header is bool BooleanMode (bool data[), int n) Given that n represents the size of data, the function returns the truth value that appears the most in data. If there is an equal number of both truth values in data, the function returns the truth value that appeared first. For instance, if data = [true, true,false, false,false, true,falsel, then the function will return false. Implarrow_forward
- I need help with programming in MATLAB. I want to know how to go from geodetic coordinates to ECI. Can you help me change this lat = 40.43094 and long = -86.915798 to geodetic coordinates. Please don't use an inbuilt MATLAB function.arrow_forwardCan you help me program this in MATLAB? Transformation from Keplerian orbital elements to cartesian coordinates. Write a function script in the form (x,y,z,vx,vy,vz) = orb2cart(a,ecc,inc,raan,argp,f). This is going from Keplerian orbital elements to the cartesian coordinates. Inputing orbital elements (a,ecc,inc,raan,argp,f) and output the state vector (x,y,z,vx,vy,vz).arrow_forwardAnswer the given question with a proper explanation and step-by-step solution. This code it good but I have to use the def pig7 function...arrow_forward
- Use C++ and don't forrget the struct functionarrow_forwardI need help with programming in MATLAB. The following code transforms cartesian coordinates to the kepler elements. Can you give me the code for transforming kepler orbital elements to cartesian coordinates. The following code gives the 6 kepler elements. Transform those elements into cartesian coordinate that match the values under the Example Usage part of the code. Can you send a screenshot so I know the output of your code matches the input of the following code? I have asked this question twice and I have gotten the exact same code as the answer and it did not match. Please make sure the results match. % Example usage:x = 1000; y = 2000; z = 3000; vx = 4; vy = -3; vz = 2; [a, ecc, inc, raan, argp, f] = cart2orb(x, y, z, vx, vy, vz); % Display the resultsdisp(['Semi-Major Axis (a): ', num2str(a), ' km']);disp(['Eccentricity (ecc): ', num2str(ecc)]);disp(['Inclination (inc): ', num2str(inc), ' degrees']);disp(['Right Ascension of Ascending Node (raan): ',…arrow_forwardConsider the following code: x = [1 2 3 4 5]; y = [4 1 2 7 9]; comp = x > y; added = [x + y, 2]; What types of variables will 'comp' and 'added' be? How many values might they contain? Explain why in a sentence or two. In MatLabarrow_forward
- In Kotlin, write a higher-order function with an expression body that takes an int n and a function f from int to int and returns the result of calling f(f(n)). For example if you call the function with n = -5 anda function that calculates absolute value, your function will return 5, and if you send the value 2 and a function that calculates the sqaure of an int, the function will return 16. in addition to the function, write the syntax to call it with:a. a function name as the function arguementb. some lambda expression as the function argumentarrow_forwardNeed help in Matlab. How do I modify this program to produce eye shapes like the pink picture using scaling, rotating and translating? Thank you! figure(1) x(1:2,1)=[1;0]; plot(x(1), x(2),'*'); grid on; hold on; axis([-15 15 -15 15]); k=1; Inc=1; for j=1:Inc:360; x(1:2,k+1)=[cosd(Inc) -sind(Inc);sind(Inc) cosd(Inc)]*x(1:2,k); plot(x(1,k+1), x(2,k+1),'^'); k=k+1; %for j=1:Inc:360; %x(1:2,k+1)=[cosd(Inc) -sind(Inc);sind(Inc) cosd(Inc)]*x(1:2,k); %plot(x(1,k+1), x(2,k+1),'^'); %k=k+1; %pause(); end for k=1:Inc:360; y1(1:2,k)=[1.5 0;0 3]*x(1:2,k); plot(y1(1,k),y1(2,k),'^') %for k=1:Inc:360; %y1(1:2,k)=[1.5 0;0 3]*x(1:2,k); %plot(y1(1,k),y1(2,k),'^') %pause(0.1); end for k=1:Inc:360; y2(1:2,k)=[3 0;0 1.5]*x(1:2,k)+[7;5]; plot(y2(1,k),y2(2,k),'^') %for k=1:Inc:360; %y2(1:2,k)=[3 0;0 1.5]*x(1:2,k)+[7;5]; %plot(y2(1,k),y2(2,k),'^') %pause(0.1); end for k=1:Inc:360; y3(1:2,k)=[3 0;0 1.5]*x(1:2,k)+[-7;5]; plot(y3(1,k),y3(2,k),'^') %for k=1:Inc:360; %y3(1:2,k)=[3 0;0 1.5]*x(1:2,k)+[-7;5];…arrow_forwardPlease use MATLAB to solve the problem.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
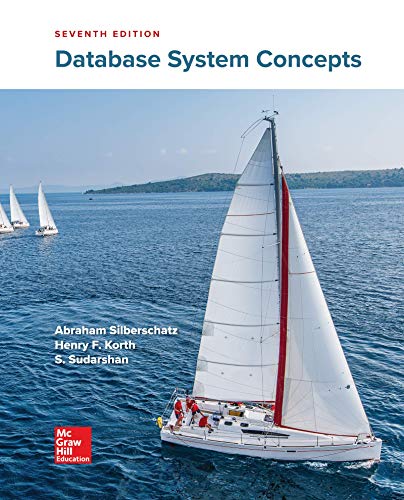
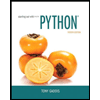
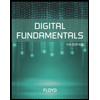
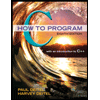
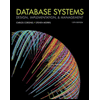
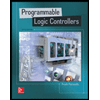