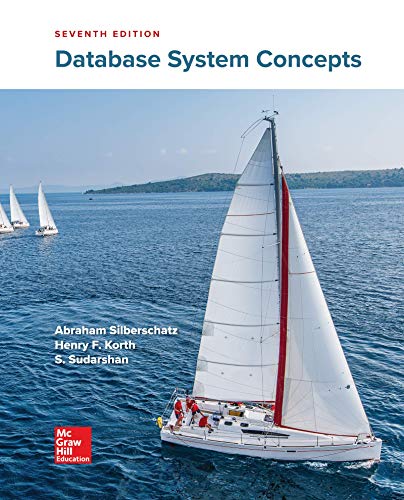
Concept explainers
my code is displayed below, just need help with this question in c++.
#include <iostream>
using namespace std;
class Vehicle{
private:
string *brand;
public:
Vehicle(){
brand = new string;
*brand = "TBD";
}
Vehicle(string b){
brand = new string;
*brand = b;
}
void output(){
cout << "Brand: " << *brand << endl;
}
void setBrand(string b){
*brand = b;
}
string getBrand(){return *brand;}
};
class Car:public Vehicle{
private:
int *weight;
public:
Car():Vehicle(){
weight = new int;
*weight = 0;
}
Car(string b, int w):Vehicle(b){
weight = new int;
*weight = w;
}
void output(){
cout << "Brand: " << getBrand() << " Weight: " << *weight << endl;
}
void setWeight(int w){
*weight = w;
}
int getWeight(){return *weight;}
};
class Boat:public Vehicle{
private:
int *hullLength;
public:
Boat():Vehicle(){
hullLength = new int;
*hullLength = 0;
}
Boat(string b, int h):Vehicle(b){
hullLength = new int;
*hullLength = h;
}
void output(){
cout << "Brand: " << getBrand() << " Hull Length: " << *hullLength << endl;
}
void sethullLength(int h){
*hullLength = h;
}
int gethullLength(){return *hullLength;}
};
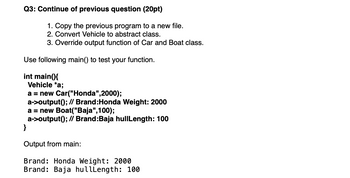

Step by stepSolved in 3 steps

- // CLASS PROVIDED: sequence (part of the namespace CS3358_FA2022)//// TYPEDEFS and MEMBER CONSTANTS for the sequence class:// typedef ____ value_type// sequence::value_type is the data type of the items in the sequence.// It may be any of the C++ built-in types (int, char, etc.), or a// class with a default constructor, an assignment operator, and a// copy constructor.//// typedef ____ size_type// sequence::size_type is the data type of any variable that keeps// track of how many items are in a sequence.//// static const size_type DEFAULT_CAPACITY = _____// sequence::DEFAULT_CAPACITY is the default initial capacity of a// sequence that is created by the default constructor.//// CONSTRUCTOR for the sequence class:// sequence(size_type initial_capacity = DEFAULT_CAPACITY)// Pre: initial_capacity > 0// Post: The sequence has been initialized as an empty sequence.// The insert/attach functions will work efficiently (without// allocating…arrow_forwardC++ #include <iostream>using namespace std; //creating struct to hold //student name,age and letter gradestruct student{ //data members string name; int age; char grade;};int main(){ //declaring object for the struct student *k = new student; //filling it with data k->name = "Kate"; k->age=24; k->grade='B'; ///then printing student data cout<<"Name:"<<k->name<<endl; cout<<"Age:"<<k->age<<endl; cout<<"Grade:"<<k->grade<<endl; return 0;}arrow_forwardC++ coding project just need some help getting the code thank you!arrow_forward
- The following struct types have been defined: typedef struct { int legs; char *sound; char *name; } pet_t; typedef struct { char *name; int age; pet_t *pet; } owner_t; A variable kid has been defined by: owner_t *kid; Functions get_owner and get_pet have the prototypes: owner_t *get_owner(char *,int,pet_t *); pet_t *get_pet (char *name, char *sound, int legs); Using kid, print a line like: Julie's pet parrot has 2 legs and says, "squawk".arrow_forwardbriefly explain this code #include<iostream>#include<string.h> using namespace std; class bank_account{int acno;char nm[100], acctype[100];float bal;public:bank_account(int acc_no, char *name, char *acc_type, float balance){acno=acc_no;strcpy(nm, name);strcpy(acctype, acc_type);bal=balance;}void deposit();void withdraw();void display();};void bank_account::deposit(){int damt1;cout<<"\n Enter Deposit Amount = ";cin>>damt1;bal+=damt1;}void bank_account::withdraw(){int wamt1;cout<<"\n Enter Withdraw Amount = ";cin>>wamt1;if(wamt1>bal)cout<<"\n Cannot Withdraw Amount";bal-=wamt1;}void bank_account::display(){cout<<"\n Name : "<<nm;cout<<"\n Account Type : "<<acctype;cout<<"\n Balance : "<<bal;}int main(){int acc_no;char name[100], acc_type[100];float balance;cout<<"\n Name : ";cin>>name;cout<<"\n Account Type : ";cin>>acc_type;cout<<"\n Balance : ";cin>>balance;bank_account…arrow_forwardPlease answer in C++ / Text book question / C++ for scientist and Engineers 3rd edition Construct a class named Fractions containing two integer data members named num and denom, used to store the numerator and denominator of a fraction having the form num/denom. Your class should include a default constructor that initializes num and denom to 1 and four operator functions for adding, subtracting, multiplying, and dividing the two fractions, as follows: Addition: a/b + c/d = (a * d + b * c) / (b * d)Subtraction: a/b - c/d = (a * d - b * c) / (b * d)Multiplication: a/b * c/d = (a* c) / (b * d)Division: (a/b) / (c/d) = (a * d) / (b * c) Finally, your class should have a member function that reduces each fraction to its terms (refer to Exercise 15 in Programming Projects for Chapter 6 for how to do this) as well as input and output functions for entering and displaying a fraction. thanksarrow_forward
- c++ programmingarrow_forwardIn C++ struct applianceType { string supplier; string modelNo; double cost; }; applianceType myNewAppliance; applianceType applianceList[25]; Write the C++ statements that prints out all the appliance info from applianceList whose modelNo is B1234.arrow_forwardC++ Language Create a UML Diagram for the Class Invoice with the provided code. Use <<constructor>> to define constructors, for each function's variable indicate whether it is a string or a integer. include <string> using namespace std; class Invoice{public:Invoice(string, string, int, int); void setPartNumber(string);string getPartNumber();void setPartDescription(string); string getPartDescription();void setQuantity(int); int getQuantity();void setPricePerItem(int); int getPricePerItem(); int getInvoiceAmount();private:string partNumber; string partDescription; int quantity; int pricePerItem; };arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
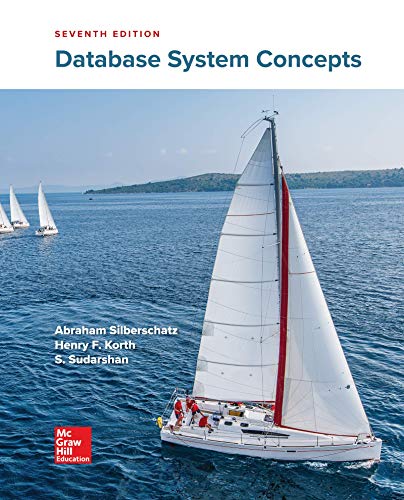
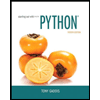
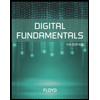
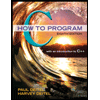
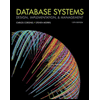
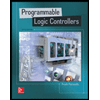