Write a python program to compute some statitistics for a class of students’ scores on an assignment. Please using loops and working with lists, so write the functions WITHOUT using min(), max(), and sum(). The method should be append(),pop() etc. The students’ scores should be stored in a list. The program should be able to compute the following: the average (mean) the median the lowest score the highest score Write separate functions for each statistic and, write Pytest tests to practice unit testing functions with list parameters. A few suggestions of things to test: a list with an even number of grades a list with an odd number of grades a sorted list an unsorted list an empty list
Write a python program to compute some statitistics for a class of students’ scores on an assignment. Please using loops and working with lists, so write the functions WITHOUT using min(), max(), and sum(). The method should be append(),pop() etc.
The students’ scores should be stored in a list. The program should be able to compute the following:
-
the average (mean)
-
the median
-
the lowest score
-
the highest score
Write separate functions for each statistic and, write Pytest tests to practice unit testing functions with list parameters. A few suggestions of things to test:
-
a list with an even number of grades
-
a list with an odd number of grades
-
a sorted list
-
an unsorted list
-
an empty list

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

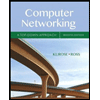
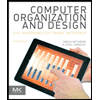
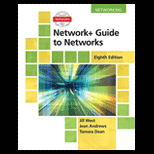
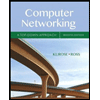
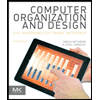
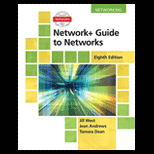
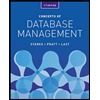
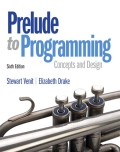
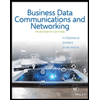