A robot is initially located at position (0, 0) in a grid [−5, 5] × [−5, 5]. The robot can move randomly in any of the directions: up, down, left, right. The robot can only move one step at a time. For each move, print the direction of the move and the current position of the robot. If the robot makes a circle, which means it moves back to the original place, print “Back to the origin!” to the console and stop the program. If it reaches the boundary of the grid, print “Hit the boundary!” to the console and stop the program. A successful run of your code may look like: Down (0,-1) Down (0,-2) Up (0,-1) Left (-1,-1) Left (-2,-1) Up (-2,0) Left (-3,0) Left (-4,0) Left (-5,0) Hit the boundary! or Left (-1,0) Down (-1,-1) Right (0,-1) Up (0,0) Back to the origin! Instructions: This program is to give you practice using the control flow, the random number generator, and output formatting. You may not use stdafx.h. Include header comments. Include to format your output. Name your file randomWalk.cpp.
A robot is initially located at position (0, 0) in a grid [−5, 5] × [−5, 5]. The robot can move randomly in any of the directions: up, down, left, right. The robot can only move one step at a time. For each move, print the direction of the move and the current position of the robot. If the robot makes a circle, which means it moves back to the original place, print “Back to the origin!” to the console and stop the
A successful run of your code may look like:
Down (0,-1)
Down (0,-2)
Up (0,-1)
Left (-1,-1)
Left (-2,-1)
Up (-2,0)
Left (-3,0)
Left (-4,0)
Left (-5,0)
Hit the boundary!
or
Left (-1,0)
Down (-1,-1)
Right (0,-1)
Up (0,0)
Back to the origin!
Instructions: This program is to give you practice using the control flow, the random number generator, and output formatting. You may not use stdafx.h. Include header comments. Include <iomanip> to format your output. Name your file randomWalk.cpp.
This is the question and I'm supposed to build this program with while loop, string, random number generator. I'm not allowed to use switch for this assignment.
I'm having trouble assigning random direction with appropriate coordinate corresponding with that direction.
This is what I have so far:
#include<iostream>
#include<iomanip>
#include<ctime>
#include<cstdlib>
#include<string>
using namespace std; i
nt x1 = 5;
int x2 = -5;
int y1_ = 5;
int y2 = -5;
bool gameOver = false;
bool Origin(int x, int y)
{ if (x == 0 && y == 0) {
cout << "Back to Origin!" << endl;
gameOver = true;
return true;
}
return false;
}
bool boundary(int x, int y) { if (x<x1 || x>x2) {
cout << "Hit the boundary!" << endl;
gameOver = true; return true;
}
if (y<y1_ || y>y2) {
cout << "Hit the boundary!" << endl;
gameOver = true;
return true;
}
return false;
}
int main(int argc, char** argv)
{ srand(static_cast<int>(time(0)));
int robotx = 0;
int roboty = 0;
while (!gameOver) {
int r = rand() % 4+1;
int x = robotx;
int y = roboty;
if (r = 0)
cout << left<<setw(7) <<"Down"<<"(" << x << "," << y << ")" << endl;
y --;
if (r=1) cout << left<<setw(7)<<"Right"<<"(" << x << "," << y << ")" << endl;
x ++;
if (r=2) cout << left<<setw(7)<<"Up" << "(" << x << "," << y << ")" << endl; y ++;
if (r=3) cout << left<<setw(7)<<"Left" << "(" << x << "," << y << ")" << endl; x --;
}
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 5 images

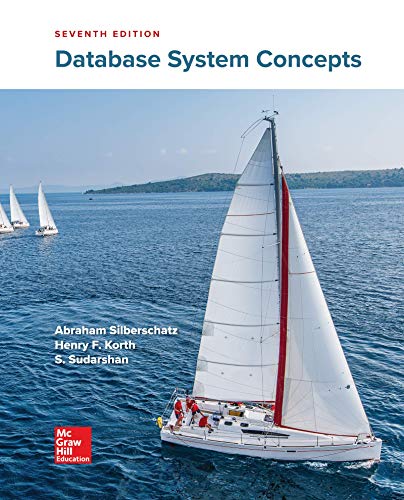
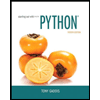
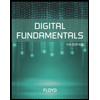
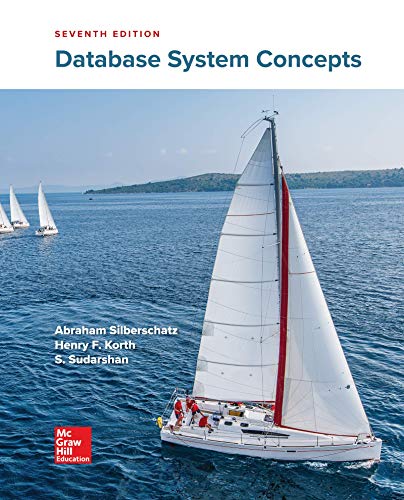
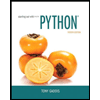
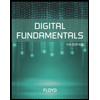
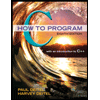
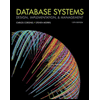
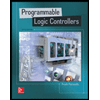