Pseudocode for this Python code (I wrote one but it does not match): import random def rollDice(): num1 = random.randint(1, 6) num2 = random.randint(1, 6) return num1, num2 def determine_win_or_lose(num1, num2): total = num1 + num2 print(f"You rolled {num1} + {num2} = {total}") if total == 2 or total == 3 or total == 12: return 0 elif total == 7 or total == 11: return 1 else: print(f"Point is {total}") return determinePointValueResult(total) def determinePointValueResult(pointValue): total = 0 result = -1 while total != 7 and total != pointValue: num1, num2 = rollDice() total = num1 + num2 print(f"You rolled {num1} + {num2} = {total}") if total == pointValue: result = 1 elif total == 7: result = 0 return result def main(): n = int(input("Enter the number of games to play: ")) i = 0 winCounter = 0 loseCounter = 0 while i < n: num1, num2 = rollDice() result = determine_win_or_lose(num1, num2) if result == 1: winCounter += 1 print("You win\n") else: loseCounter += 1 print("You lose\n") i += 1 print(f"Game results: {winCounter} wins and {loseCounter} losses") if __name__ == "__main__": main()
Pseudocode for this Python code (I wrote one but it does not match):
import random
def rollDice():
num1 = random.randint(1, 6)
num2 = random.randint(1, 6)
return num1, num2
def determine_win_or_lose(num1, num2):
total = num1 + num2
print(f"You rolled {num1} + {num2} = {total}")
if total == 2 or total == 3 or total == 12:
return 0
elif total == 7 or total == 11:
return 1
else:
print(f"Point is {total}")
return determinePointValueResult(total)
def determinePointValueResult(pointValue):
total = 0
result = -1
while total != 7 and total != pointValue:
num1, num2 = rollDice()
total = num1 + num2
print(f"You rolled {num1} + {num2} = {total}")
if total == pointValue:
result = 1
elif total == 7:
result = 0
return result
def main():
n = int(input("Enter the number of games to play: "))
i = 0
winCounter = 0
loseCounter = 0
while i < n:
num1, num2 = rollDice()
result = determine_win_or_lose(num1, num2)
if result == 1:
winCounter += 1
print("You win\n")
else:
loseCounter += 1
print("You lose\n")
i += 1
print(f"Game results: {winCounter} wins and {loseCounter} losses")
if __name__ == "__main__":
main()

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

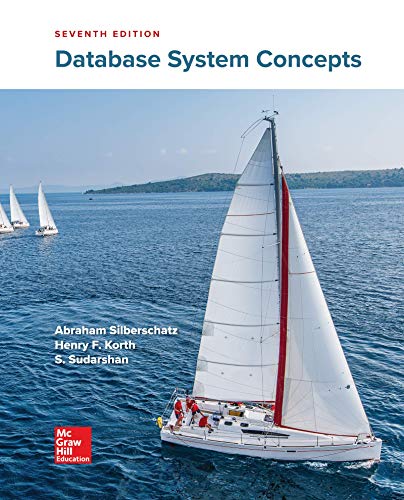
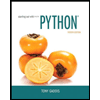
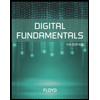
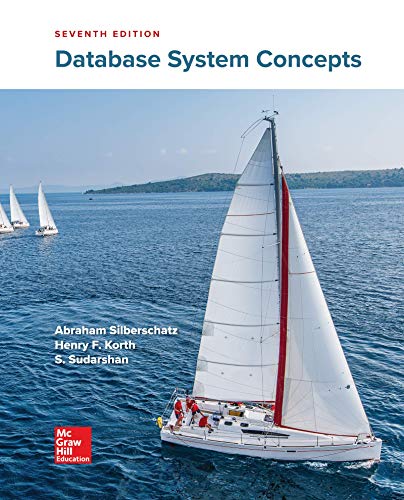
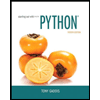
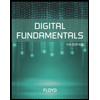
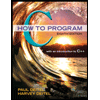
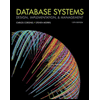
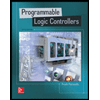