please rewrite down code using for loop without iterator
Types of Loop
Loops are the elements of programming in which a part of code is repeated a particular number of times. Loop executes the series of statements many times till the conditional statement becomes false.
Loops
Any task which is repeated more than one time is called a loop. Basically, loops can be divided into three types as while, do-while and for loop. There are so many programming languages like C, C++, JAVA, PYTHON, and many more where looping statements can be used for repetitive execution.
While Loop
Loop is a feature in the programming language. It helps us to execute a set of instructions regularly. The block of code executes until some conditions provided within that Loop are true.
please rewrite down code using for loop without iterator
using namespace std;
struct Assest {
string Type;
string Title;
string Author;
string Year_Publication;
string UnQID;
string ISBN;
int num_Items_Record;
int num_Items_lib;
bool isExistIntoLib;
Assest() {
}
Assest(string tmpType, string tmpTitle, string tmpAuthor, string tmpYear_Publication, string tmpISBN , int tmpNum_Items_lib, int tmpNum_Items_Record, string tmpUnQID ) {
Type = tmpType;
Title = tmpTitle;
Author = tmpAuthor;
Year_Publication = tmpYear_Publication;
UnQID = tmpUnQID;
num_Items_Record = tmpNum_Items_Record;
num_Items_lib = tmpNum_Items_lib;
ISBN = tmpISBN;
isExistIntoLib = false;
}
string toString() {
return Type + ";"+ Title + ";" + Author + ";" + Year_Publication + ";" + ISBN + ";" + to_string(num_Items_lib) + ";" + to_string(num_Items_Record) + ";" + UnQID+";"+ (isExistIntoLib ? "Borrowed" : "BACK");
}
// function Print
void PrintAll() {
cout << "Type : " << Type << endl <<"Title : " << Title << " "<< "Author : " << Author << endl << "Year : " << Year_Publication << endl;
cout << "ID: " << UnQID << endl<< "ISBN : " << ISBN << endl;
cout << "Num Items For Record : " << num_Items_Record << " " << "Num Items For Library : " << num_Items_lib << endl;
cout << "Asset is " << (isExistIntoLib ? "Borrowed" : "BACK") << endl << endl;
}
void setDataRows(vector<Assest> tmpDataRows) {
this->DataRows = tmpDataRows;
}
/// ADD function
void ADD() {
string tmpType, tmpTitle, tmpAuthor, tmpYear_Publication, tmpUnQID;
int tmpNum_Items_Record, tmpNum_Items_lib;
string tmpISBN;
cin.ignore();
cout << "Enter Type : ";
getline(cin, tmpType, '\n');
cout << "Enter Title : ";
getline(cin, tmpTitle, '\n');
cout << "Enter AUTHOR : ";
getline(cin, tmpAuthor, '\n');
cout << "Enter YEAR : ";
getline(cin, tmpYear_Publication, '\n');
cout << "Enter ID : ";
getline(cin, tmpUnQID, '\n');
cout << "Enter ISBN : ";
getline(cin, tmpISBN, '\n');
cout << "Enter Num Items record : ";
cin >> tmpNum_Items_Record;
cout << "Enter Num itms library : ";
cin >> tmpNum_Items_lib;
Assest MyAssest = Assest(tmpType, tmpTitle, tmpAuthor, tmpYear_Publication, tmpISBN , tmpNum_Items_lib, tmpNum_Items_Record,tmpUnQID);
DataRows.push_back(MyAssest);
}
/// remove function
void REMOVE() {
string assetId;
cout << "INPUT ID : " << endl;
cin >> assetId;
for (vector<Assest>::iterator it = DataRows.begin(); it != DataRows.end();)// LOOP
{
if (it->UnQID == assetId) {
it = DataRows.erase(it);//remove if get id
cout << "Asset is Removed" << endl;
break;
}
else
++it;
}
}
//SEARCH Title
void searchTITLE() {
bool isexist = false;
string title;
cout << "INPUT Title : " << endl;
cin.ignore();
getline(cin, title);
for (vector<Assest>::iterator it = DataRows.begin(); it != DataRows.end();)//loop
{
if (it->Title == title) {
it->PrintAll();
isexist = true;
}
++it;
}
if (!isexist)cout << "Sorry Your searched asset not found" << endl;
}

Step by step
Solved in 2 steps

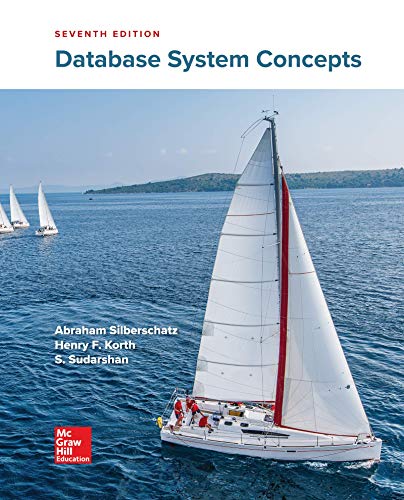
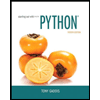
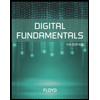
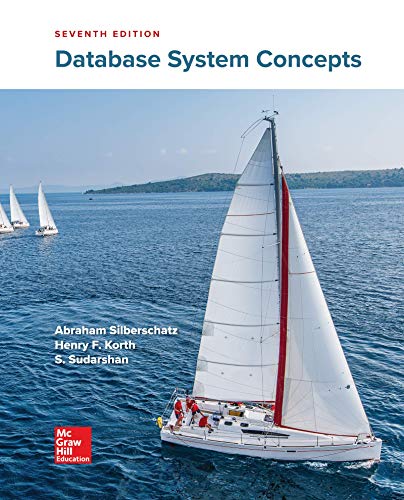
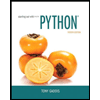
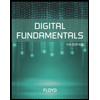
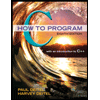
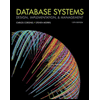
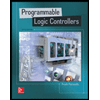