Introduction
Any task which is repeated more than one time is called a loop. Basically, loops can be divided into three types as while, do-while and for loop. There are so many programming languages like C, C++, JAVA, PYTHON, and many more where looping statements can be used for repetitive execution.
Loops in C
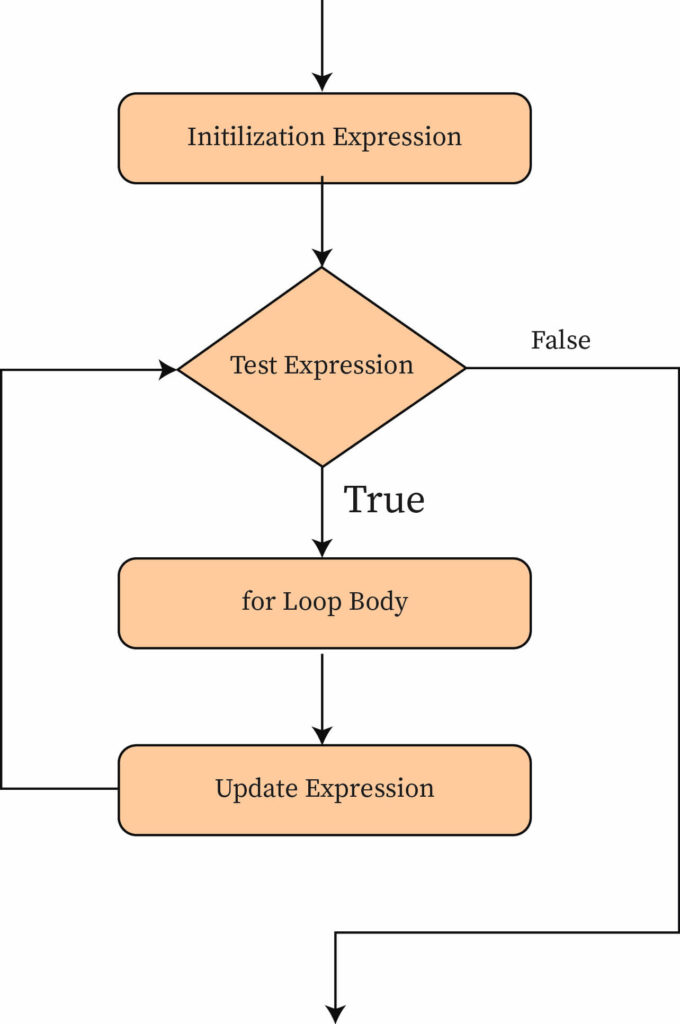
In the C programming language, three types of loops have been used for the execution of the programs.
- While loop: While loop is a pretest loop. It first tests a specified conditional expression and as long as the condition, expression is true, action taken. While loop also known as an entry control loop.
While (condition)
{
Statement;
}
- Do-while loop: Do-while loop more like a while loop & except that it tests the condition at the end of the loop body. Do-while loop also known as exit control loop.
Do
{
Statement;
}
While (condition);
- For loop: A for loop enables us to program n number of steps together in one line. In for loop, a loop variable is used to control the loop.
For (initialization ; condition ; increment / decrement)
{
Statement;
}
Loops in C++
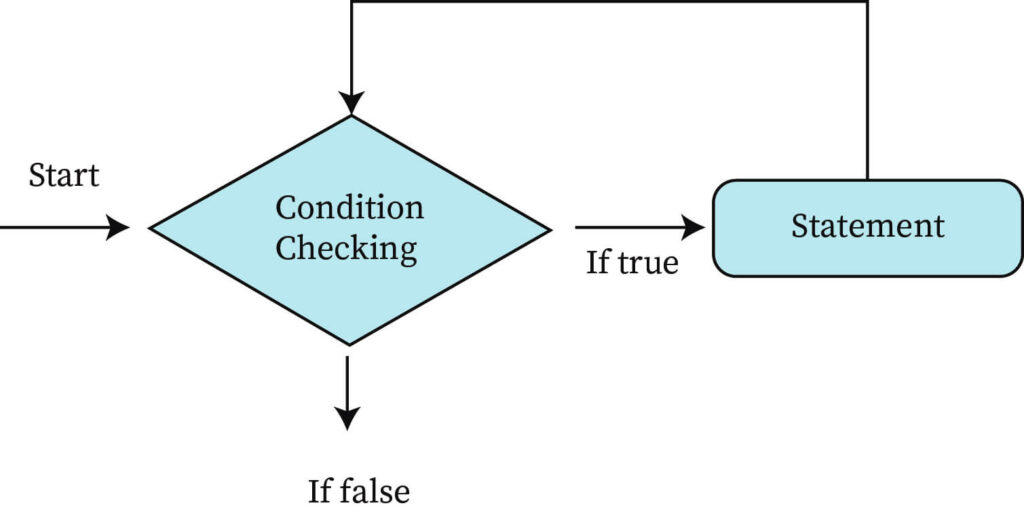
There are no additional loops in C++ for programming execution. It is totally like C language.
Loops in Python
- While loops: These are also known as indefinite pre conditional loops. There is no guarantee ahead of time regarding how many times the loop will iterate.
While expression:
Statements
- For Loops: For loop is a python loop which repeats a group of statements a specified number of times. Boolean condition, initial values of the counting variable and incrementation of counting variable specifies the component of loop.
For < variable > in < range >:
Statement 1
Statement 2
……….
……….
Statement n
- Nested loops: Python programming allows use of a loop inside another loop. This is called nested loop.
For iterating _ var in sequence:
For iterating _ var in sequence:
Statements
Statements
Loops in Java
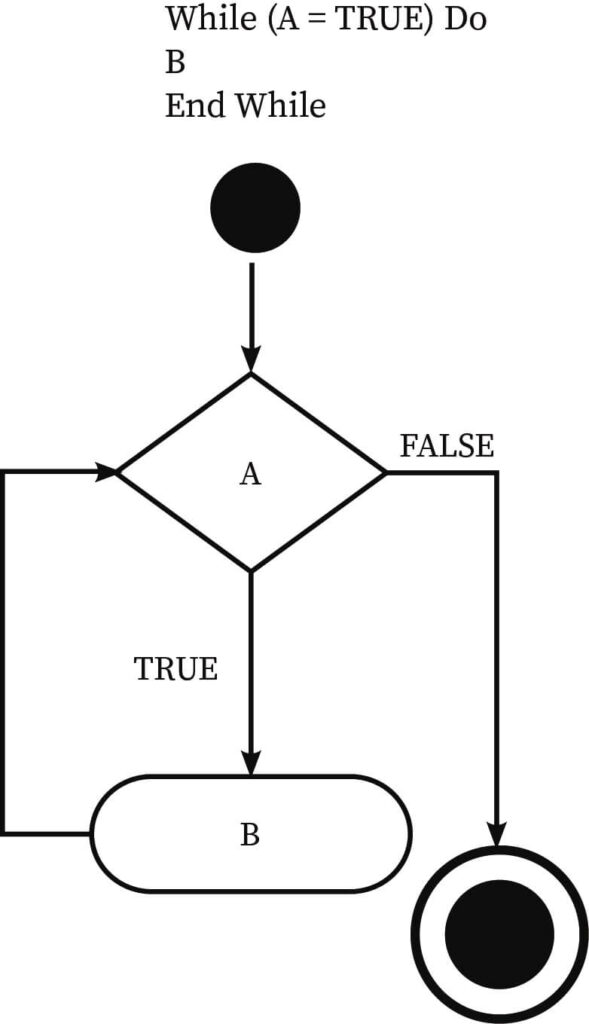
All other loops are the same as other languages & in this language a loop named as for-each loop have been specified.
- For each-loop: For Each loop is another approach to navigate through the array or collection
for (data type variable: array | collection)
{
…………
…………
Statements
…………
…………
}
- While loop: While loop iterates through a block of code if a specified condition is true
While (condition)
{
…………
…………
Statements
…………
…………
}
- Do-while loop: Do-while loop will execute the code block once, before checking if the condition is true, then it will repeat the loop if the condition is true.
do
{
…………
…………
Statements
…………
…………
}
While (condition);
- For loop: for loop is a control flow statement that iterates a part of the programs multiple times.
for (initialization; condition; increment/decrement)
{
…………
…………
Statements
…………
…………
}
Loops in R
- For loop: This loop iterates over a collection of values.
For any _ Variable in Collection _ of _ Values
{
// code to be executed
}
- Repeat loop: A repeat loop executes a set of statements till a terminate statement is found.
Repeat
{
# commands
If (condition)
{
Break
}
}
- While loop: Executes a set of statements till a condition is true,
While (condition)
{
// code to be executed
}
Loops in MATLAB
- For loop: for loop is a repetition control structure that allows you to efficiently write a loop that needs to execute a specific number of times.
for index = values
…………
Statements
…………
End
Example:
for a = 10:20
fprintf('value of a: %dn', a);
End
- While loop: The while loop repeatedly executes statements while a specified condition is true.
while expression
statements
end
Example: n = 10;
f = n;
while n > 1
n = n – 1;
n = f * n;
end
disp ([‘n! = ‘num2str (f)])
Output: 3628800
- Nested loops: Uses one loop inside another loop.
for m = 1:j
for n = 1:k
<statements>
end
- Nested while loop: While statement inside another while statement.
While < expression1>
While < expression2 >
< Statements >
end
end
Macro Loops
- For the next loop: Allow us to go through a block of code for the specified number of times. Counter or any variable can be used to run the loop.
For Counter = Start To End [Step Value]
[Code Block to Execute]
Next [counter]
- Do while loop: Allow us to check for a condition and run the loop while the condition is met.
Do [while condition]
[Code block to execute]
Loop
Or we can write it as:
Do
[Code block to execute]
Loop [while condition]
- For each loop: Helps to go through each of the objects in a collection and perform some action on it. Also referred to as a for-each-next loop.
For each element in collection
[Code block to execute]
Next [element]
- Do until loop: It loops until the specified condition is met.
Do [ until condition]
[Code block to execute]
Loop
Or we can write it as:
Do
[ Code block to execute]
Loop [until condition]
The various loops in most common programming languages are
Types | Explanation |
for | It iterates a portion of the program multiple times. |
while | It iterates a portion of the program repetitively until the condition is true. |
do while | It iterates a portion of the programs once and then iterates according to the boolean condition. |
Nested | It is a loop that iterates within another loop. |
Nested while | While statement inside another while statement. |
For each | Helps to go through each of the objects in a collection and perform some action on it. |
Repeat | It iterates a block of code repetitively until break statement is found. |
For Next | It iterates a portion of code for a specified number of times. |
Do Until | It loops until specific condition has happened. |
Common Mistakes
- Syntax should be used as mentioned.
- Do not misplace a semicolon.
- Usage of comparison operators should be correct for example ‘<’ operator returns numbers lesser than the given number and ‘<=’ operator returns both the given number and the number lesser than that. This error is known as an off-by-one error.
- Usage of wrong conditional expression as the loop iterates as the condition is true and the loop will not iterate if the condition is false.
- While using the loop for iterating arrays use the correct initializing value because the array element starts from 0.
- Always initialize conditional value.
- Do not use the wrong condition as it will stop the loop to execute.
Context and Applications
This topic is significant in the professional exams for both undergraduate and graduate courses, especially for
- B.Tech. / B.E. in Computer Science
- M.Tech. / M.E. in Computer Science
Want more help with your computer science homework?
*Response times may vary by subject and question complexity. Median response time is 34 minutes for paid subscribers and may be longer for promotional offers.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.
Loops Homework Questions from Fellow Students
Browse our recently answered Loops homework questions.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.