Please follow instructions package chapter07;
public class BSTNode
{
private int info;
private BSTNode left;
private BSTNode right;
public BSTNode(int info)
{
this.info = info;
this.left = null;
this.right = null;
}
public void setInfo(int info)
{
this.info = info;
}
public int getInfo()
{
return this.info;
}
public void setRight(BSTNode right)
{
this.right = right;
}
public BSTNode getRight()
{
return this.right;
}
public void setLeft(BSTNode left)
{
this.left = left;
}
public BSTNode getLeft()
{
return this.left;
}
} package chapter07;
public class BinarySearchTree
{
protected BSTNode root;
protected boolean success;
public BinarySearchTree()
{
this.root = null;
}
public boolean contains(int value)
{
BSTNode curr = root;
while(curr != null)
{
if(value == curr.getInfo())
{
return true;
}
else if(value < curr.getInfo())
{
curr = curr.getLeft();
}
else
{
curr = curr.getRight();
}
}
return false;
}
public boolean add(int value)
{
root = add(value, root);
return success;
}
private BSTNode add(int value, BSTNode curr)
{
if(curr == null)
{
curr = new BSTNode(value);
success = true;
}
else if(value < curr.getInfo())
{
curr.setLeft(add(value, curr.getLeft()));
}
else if(value > curr.getInfo())
{
curr.setRight(add(value, curr.getRight()));
}
else
{
success = false;
}
return curr;
}
public void preOrder()
{
System.out.print("Pre Order: ");
preOrder(root);
System.out.println();
}
private void preOrder(BSTNode curr)
{
if(curr != null)
{
System.out.print(curr.getInfo() + " ");
preOrder(curr.getLeft());
preOrder(curr.getRight());
}
}
public void inOrder()
{
//Complete this method as required in the homeowrk instructions
} public void postOrder()
{
//Complete this method as required in the homeowrk instructions
}
public int countLeaves()
{
//Complete this method as required in the homeowrk instructions
}
public int size()
{
//Complete this method as required in the homeowrk instructions
}
public int height()
{
//Complete this method as required in the homeowrk instructions
}
public boolean remove(int target)
{
root = remove(target, root);
return success;
}
private BSTNode remove(int target, BSTNode curr)
{
if(curr == null)
{
success = false;
}
else if(target < curr.getInfo())
{
curr.setLeft(remove(target, curr.getLeft()));
}
else if(target > curr.getInfo())
{
curr.setRight(remove(target, curr.getRight()));
}
else
{
curr = removeNode(curr);
success = true;
}
return curr;
}
private BSTNode removeNode(BSTNode curr)
{
if(curr.getLeft() == null)
{
return curr.getRight();
}
else if(curr.getRight() == null)
{
return curr.getLeft();
}
else
{
int data = getRightMostData(curr.getLeft());
curr.setInfo(data);
curr.setLeft(remove(data, curr.getLeft()));
return curr;
}
}
private int getRightMostData(BSTNode subtree)
{
BSTNode temp = subtree;
while (temp.getRight() != null)
{
temp = temp.getRight();
}
return temp.getInfo();
}
}
Please follow instructions package chapter07; public class BSTNode { private int info; private BSTNode left; private BSTNode right; public BSTNode(int info) { this.info = info; this.left = null; this.right = null; } public void setInfo(int info) { this.info = info; } public int getInfo() { return this.info; } public void setRight(BSTNode right) { this.right = right; } public BSTNode getRight() { return this.right; } public void setLeft(BSTNode left) { this.left = left; } public BSTNode getLeft() { return this.left; } } package chapter07; public class BinarySearchTree { protected BSTNode root; protected boolean success; public BinarySearchTree() { this.root = null; } public boolean contains(int value) { BSTNode curr = root; while(curr != null) { if(value == curr.getInfo()) { return true; } else if(value < curr.getInfo()) { curr = curr.getLeft(); } else { curr = curr.getRight(); } } return false; } public boolean add(int value) { root = add(value, root); return success; } private BSTNode add(int value, BSTNode curr) { if(curr == null) { curr = new BSTNode(value); success = true; } else if(value < curr.getInfo()) { curr.setLeft(add(value, curr.getLeft())); } else if(value > curr.getInfo()) { curr.setRight(add(value, curr.getRight())); } else { success = false; } return curr; } public void preOrder() { System.out.print("Pre Order: "); preOrder(root); System.out.println(); } private void preOrder(BSTNode curr) { if(curr != null) { System.out.print(curr.getInfo() + " "); preOrder(curr.getLeft()); preOrder(curr.getRight()); } } public void inOrder() { //Complete this method as required in the homeowrk instructions } public void postOrder() { //Complete this method as required in the homeowrk instructions } public int countLeaves() { //Complete this method as required in the homeowrk instructions } public int size() { //Complete this method as required in the homeowrk instructions } public int height() { //Complete this method as required in the homeowrk instructions } public boolean remove(int target) { root = remove(target, root); return success; } private BSTNode remove(int target, BSTNode curr) { if(curr == null) { success = false; } else if(target < curr.getInfo()) { curr.setLeft(remove(target, curr.getLeft())); } else if(target > curr.getInfo()) { curr.setRight(remove(target, curr.getRight())); } else { curr = removeNode(curr); success = true; } return curr; } private BSTNode removeNode(BSTNode curr) { if(curr.getLeft() == null) { return curr.getRight(); } else if(curr.getRight() == null) { return curr.getLeft(); } else { int data = getRightMostData(curr.getLeft()); curr.setInfo(data); curr.setLeft(remove(data, curr.getLeft())); return curr; } } private int getRightMostData(BSTNode subtree) { BSTNode temp = subtree; while (temp.getRight() != null) { temp = temp.getRight(); } return temp.getInfo(); } }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Please follow instructions
package chapter07;
public class BSTNode
{
private int info;
private BSTNode left;
private BSTNode right;
public BSTNode(int info)
{
this.info = info;
this.left = null;
this.right = null;
}
public void setInfo(int info)
{
this.info = info;
}
public int getInfo()
{
return this.info;
}
public void setRight(BSTNode right)
{
this.right = right;
}
public BSTNode getRight()
{
return this.right;
}
public void setLeft(BSTNode left)
{
this.left = left;
}
public BSTNode getLeft()
{
return this.left;
}
}
package chapter07;
public class BinarySearchTree
{
protected BSTNode root;
protected boolean success;
public BinarySearchTree()
{
this.root = null;
}
public boolean contains(int value)
{
BSTNode curr = root;
while(curr != null)
{
if(value == curr.getInfo())
{
return true;
}
else if(value < curr.getInfo())
{
curr = curr.getLeft();
}
else
{
curr = curr.getRight();
}
}
return false;
}
public boolean add(int value)
{
root = add(value, root);
return success;
}
private BSTNode add(int value, BSTNode curr)
{
if(curr == null)
{
curr = new BSTNode(value);
success = true;
}
else if(value < curr.getInfo())
{
curr.setLeft(add(value, curr.getLeft()));
}
else if(value > curr.getInfo())
{
curr.setRight(add(value, curr.getRight()));
}
else
{
success = false;
}
return curr;
}
public void preOrder()
{
System.out.print("Pre Order: ");
preOrder(root);
System.out.println();
}
private void preOrder(BSTNode curr)
{
if(curr != null)
{
System.out.print(curr.getInfo() + " ");
preOrder(curr.getLeft());
preOrder(curr.getRight());
}
}
public void inOrder()
{
//Complete this method as required in the homeowrk instructions
}
public void postOrder()
{
//Complete this method as required in the homeowrk instructions
}
public int countLeaves()
{
//Complete this method as required in the homeowrk instructions
}
public int size()
{
//Complete this method as required in the homeowrk instructions
}
public int height()
{
//Complete this method as required in the homeowrk instructions
}
public boolean remove(int target)
{
root = remove(target, root);
return success;
}
private BSTNode remove(int target, BSTNode curr)
{
if(curr == null)
{
success = false;
}
else if(target < curr.getInfo())
{
curr.setLeft(remove(target, curr.getLeft()));
}
else if(target > curr.getInfo())
{
curr.setRight(remove(target, curr.getRight()));
}
else
{
curr = removeNode(curr);
success = true;
}
return curr;
}
private BSTNode removeNode(BSTNode curr)
{
if(curr.getLeft() == null)
{
return curr.getRight();
}
else if(curr.getRight() == null)
{
return curr.getLeft();
}
else
{
int data = getRightMostData(curr.getLeft());
curr.setInfo(data);
curr.setLeft(remove(data, curr.getLeft()));
return curr;
}
}
private int getRightMostData(BSTNode subtree)
{
BSTNode temp = subtree;
while (temp.getRight() != null)
{
temp = temp.getRight();
}
return temp.getInfo();
}
}

Transcribed Image Text:Look for BSTNode.java and BinarySearch Tree.java
BinarySearch Tree.java class contains the methods completed
few additional methods.
You are required to complete the following method
for binarysearchtree.java
and
countLeaves (): The method doesn't take any parameters and returns an
int, the method returns the number of leaves in the Binary Search Tree.
-size(): The method doesn't take any parameters and returns an int, the
method returns the nodes in the Binary Search Tree.
inOrder (): The method doesn't take any parameters and doesn't return
any value, the method displays the values stored in the BST using an In
Order traversal.
postOrder (): The method doesn't take any parameters and doesn't
return any value, the method displays the values stored in the BST using a
Post Order traversal.
height(): The method doesn't take any parameters and returns an int,
the method returns the height of the Binary Search Tree.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 3 images

Recommended textbooks for you
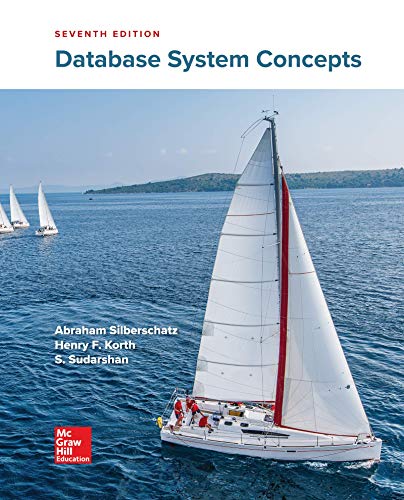
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
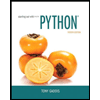
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
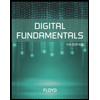
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
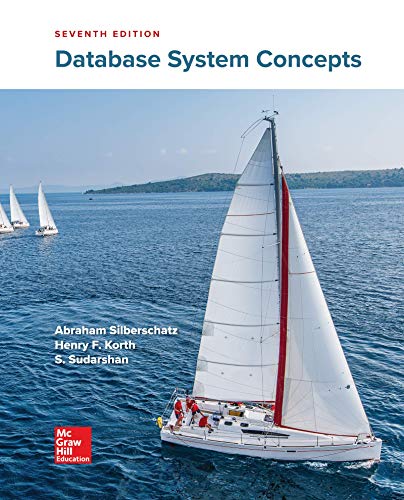
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
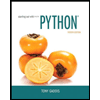
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
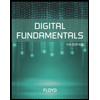
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
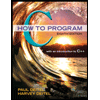
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
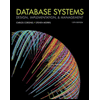
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
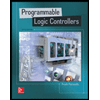
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education